Question
// Store saved numbers in an array let savedNumbers = []; // Addition function function add(num1, num2) { return num1 + num2; } // Subtraction
// Store saved numbers in an array
let savedNumbers = [];
// Addition function
function add(num1, num2) {
return num1 + num2;
}
// Subtraction function
function subtract(num1, num2) {
return num1 - num2;
}
// Multiplication function
function multiply(num1, num2) {
return num1 * num2;
}
// Division function
function divide(num1, num2) {
return num1 / num2;
}
// Remainder function
function remainder(num1, num2) {
return num1 % num2;
}
// Exponential function
function exponential(num1, num2) {
return Math.pow(num1, num2);
}
// Square root function
function sqrt(num) {
return Math.sqrt(num);
}
// Save function
function save(num) {
savedNumbers.push(num);
console.log(`Saved ${num}`);
}
// List function
function list() {
console.log(`Saved numbers: ${savedNumbers.join(', ')}`);
}
// Retrieve function
function retrieve(index) {
if (index > 0 && index <= savedNumbers.length) {
console.log(`Retrieved ${savedNumbers[index - 1]}`);
return savedNumbers[index - 1];
} else {
console.log(`Result does not exist at location.`);
}
}
// Main loop
while (true) {
// Get user input
const input = prompt('Enter a command (e.g. 10 + 20, M, P, R1)');
// Parse input
let num1, num2, operator, saveIndex;
if (input.startsWith('R')) {
// Retrieve command
saveIndex = parseInt(input.slice(1));
result = retrieve(saveIndex);
} else if (input === 'M') {
// Save command
save(result);
} else if (input === 'P') {
// List command
list();
} else {
// Calculate result
[num1, operator, num2] = input.split(' ');
num1 = parseInt(num1);
num2 = parseInt(num2);
switch (operator) {
case '+':
result = add(num1, num2);
break;
case '-':
result = subtract(num1, num2);
break;
case '*':
result = multiply(num1, num2);
break;
case '/':
result = divide(num1, num2);
break;
case '%':
result = remainder(num1, num2);
break;
case '^':
result = exponential(num1, num2);
break;
case 'sqrt':
result = sqrt(num1);
break;
default:
console
}
}
}
Can you fix this code in javascript so it can work following these rules:
Users now should be able to use the = operator or enter to do calculations.
Program should be able to handle incorrect inputs (like other characters or symbols). If a user enters incorrect input, the program resumes from the last correct input. No exceptions should occur.
Users should be able to use the M character to save the last input or result. Users can save as many numbers as they want. Users can list the saved numbers by using the P character. User can retrieve and continue with any number they want from the list by using Rn where n is any number from the list larger than 0 (e.g. R1 will retrieve the first save element, R2 second, ) Entering invalid number should print: Result does not exist at location. List exists as long as the program runs. Everytime program is restarted the list is empty. Store list in memory.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
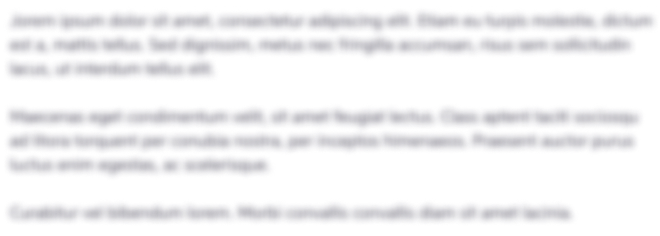
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started