Question
String Formatting Creating nicely formating strings is built into Python 3. You can read about the syntax here. Blank spaces that you want filled in
String Formatting
Creating nicely formating strings is built into Python 3. You can read about the syntax here. Blank spaces that you want filled in are given with {}. Within the brackets, you can describe how the data is shown.
>>> "I just bought ${:.2f} in goods and services.".format(19.7863) >>> 'I just bought $19.79 in goods and services.' >>> "{:+<25}".format("Oranges") 'Oranges++++++++++++++++++' >>> "{:+>25}".format("Oranges") '++++++++++++++++++Oranges'
In the above example, the {} denote a blank that should be filled. The : says we are going to format that value. The .2 says show two decimals. The f says the number is a float.
Getting the Date
Python 3 has a datetime library for working with dates. To get a timestamp for the current date, you can use the following code.
>>> import datetime >>> str(datetime.datetime.now()) >>> '2017-10-17 13:30:28.983682' The __str__ Method Python supports several "special" methods that a user can define in their class. Once such method is called __str__. If you define this method (and it must return a string), then print(Object), will call this method automatically in order to get a string representation of the object for use in printing the object to the command line. This concept is called operator overloading and will be explored in greater detail in Week 3.
The Item Class
The first thing we'll need to do is design and implement an items class! We can instantiate it to create the item objects that are part of the receipt.
The item class should have three attributes.
- __name - A string with the item name.
- __price - A float with the item price in dollars.
- __taxable - A boolean that is true if the item is taxed.
The item class should have the following methods.
- __init__ - The constructor.
- __str__ - returns the item as a string.
- getPrice - return the price of the item.
- getTax - Takes the tax rate as a parameter. Returns the tax charged on the item.
The Receipt Class
The receipt class should have two attributes.
- __tax_rate - The tax rate in this area.
- __purchases - A list of items.
The Receipt class should have the following methods.
- __init__ - The constructor. Takes the tax rate.
- __str__ - returns the full receipt as a string.
- addItem - adds a new item to the receipt.
Programming Project
Implement a program, lab2.py, that asks the users for items. For each item, read in the name, price, and if the item is taxable. When the user says that do not want to add any more items, print out the full receipt.
The receipt must have the following contents:
- Each item listed with it's price.
- The total cost of the items.
- The total tax charged on all items.
- The grand total with tax added.
- The current date when the receipt we generated.
- All values must be shown to two decimal places.
Hint: Formatted the numbers before you try to align them. Use two columns of 20 characters to make the lines look nice.
Scoring
The score for the assignment is determined as follows.
- 10pts - Attended Lab
- 5pts - Acted as Driver
- 5pts - Acted as Observer
- 10pts - Item Class Constructor
- 10pts - Item Class String Method
- 10pts - Item Class getPrice Method
- 10pts - Item Class getTax Method
- 10pts - Receipt Class Constructor
- 10pts - Receipt Class String Method
- 10pts - Receipt Class addItem Method
- 10pts - Completed Program
Example Execution Trace
Welcome to Receipt Creator Enter Item name: Hot Dog Enter Item Price: 5.15 Is the item taxable (yes/no): no Add another item (yes/no): yes Enter Item name: Soda Enter Item Price: 2.50 Is the item taxable (yes/no): yes Add another item (yes/no): yes Enter Item name: Pretzel Enter Item Price: 0.50 Is the item taxable (yes/no): no Add another item (yes/no): yes Enter Item name: Candy Bar Enter Item Price: 1.25 Is the item taxable (yes/no): yes Add another item (yes/no): no ----- Receipt 2018-01-02 16:21:49.515170 ----- Hot Dog_____________________________5.15 Soda________________________________2.50 Pretzel_____________________________0.50 Candy Bar___________________________1.25 Sub Total___________________________9.40 Tax_________________________________0.26 Total_______________________________9.66
Step by Step Solution
There are 3 Steps involved in it
Step: 1
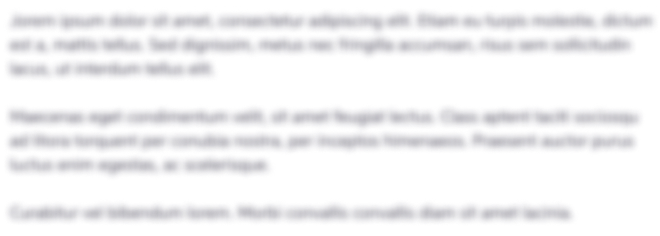
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started