Question
String Manipulation The task of this program is to read numbers from data/Phone.txt and display appropriately. Right now the code just prints out the files
String Manipulation
The task of this program is to read numbers from data/Phone.txt and display appropriately. Right now the code just prints out the files contents line by line. You should create an arrayList of every phone number and return that to the caller. We have created output variable for you to insert each number into. So we will go through what you are given first. You can see the format of the phone numbers are the following:
Country code is before the first dash (1-3 digits)
Between the first and second dashes are the city code (or area code)
After the second dash is the phone number
5-8 digits
May or may not have dash in the middle
Your job is to separate the phone number String into 3 parts and print out as shown in the expected output.
Making an ArrayList of People
We will practice using more Arraylist to create another list of objects. This time it will be a list of person objects, ie people. First you will have to complete the class definition of a Person object. Then by utilizing an arrayList keep track of all the people listed in different files.
Task 2: Person.java
Take a look at Person.java. It has 3 private variables: name, age and location. Fill-in or fix all the methods inside this Class.
Task 3: People.java
This program works by reading information about each person inside the file. There are three traits for each person: name, age and location. You need to create a Person object pointed to by people array for each line in the input file. The expected output is created by calling accessor methods on each person object. You job is to just change the part that says fill-in. The rest of the code is there to make testing easier and to make sure you do everything in an expected way. The program should runs as is but you will see it prints out no useful information.
people1.txt has the following features:
First entry is the name of the person (one word)
Second entry is the age (one number)
Third entry is the location (one word)
people2.txt has more complicated data format:
Tab delimited
First entry can be multiple words separated by space
Second entry is a number for age
Last entry can also be multiple words for location
Give the method call to useDelimiter() to use tab character.
Do you need next() or nextLine() to handle multiple words?
Task 4: IORunner.java
Put in appropriate calls to People.java so it prints out exactly as the Expected output for each of the files.
Expected Output: Enter the file name: data/people1.txt Abhay @SJ is 82 Adam @SC is 71 Alan @SC is 69 Alexander @LA is 66 Alexander @NF is 64 Alfred @PD is 64 Ana @Madera is 63 Analisa @SF is 63 Andrea @LA is 62 Andrew @SF is 62 Angelina @LA is 61 Total people 11 ********************************* Enter the file name: data/people2.txt Abhay @San Jose is 82 Adam @Santa Clara is 71 Alan @Santa Cruz is 69 Alexander @Los Angeles is 66 Alexander @North Fork is 64 Alfred @Palm Desert is 64 Analisa @San Francisco is 63 Andrea @La is 62 Andrew @San Francisco is 62 Angelina @Los Angeles is 61 Antonietta Vicky @Altadena is 60 Arun Premnath @San Francisco is 60 Chih-sung (aka Ja) @North Fork is 52 Colin @San Francisco is 51 Cynthia @Eureka is 50 Dalila @Utrecht is 50 Dana Colleen @Santa Monica is 50 Daniel @San Jose is 49 Kristal @OR is 36 Kristin Lunney @San Francisco is 36 Kristina @Santa Monica is 35 Kurt @FL is 35 Lauren @San Francisco is 34 Lien T. @Santa Cruz is 34 Lifen @Del Mar is 34 Total people 25
package io;
import java.util.ArrayList;
public class IORunner {
public static void main(String[] args) { // edit as necessary //testPeople();
}
public static void testPeople() { // fill in as necessary }
}
package io;
import java.util.*; import java.io.*;
public class People { public ArrayList
// end of code to be filled in } input.close(); } catch ( NoSuchElementException e){ System.out.println(e); } catch (FileNotFoundException e) { System.out.println(e); } return people; }
public void printPeople(ArrayList
+1-555-555-5555 +1-800-555-1212 +61-3-9527-9527 44-1289-555555 }
package io;
// CONTAINS SOLUTIONS -- JAR THIS
public class Person { private String name; private int age; private String location;
public Person() { this.name = ""; this.age = 0; this.location = ""; } public Person(String name, int age, String location) { // Fill-in
}
public void setName(String name) { // Fill-in
}
public String getName() { // Fix return "";
}
public void setAge(int age) { // Fill-in }
public int getAge() { // Fix return 0;
} public void setLocation(String location) { // Fill-in
}
public String getLocation() { // Fix return "";
} // every object in java has a toString method. The @Override line // is an annotation telling Java that the definition below should // replace the default method. // Fill in the definition below such that it returns a String that // describes a particular Person. @Override public String toString() { // fix // return "a person"; return ""; }
}
package io;
import java.util.*; import java.io.*;
public class PhoneNums {
public ArrayList
String filename = "data/phone.txt"; ArrayList
try { Scanner input = new Scanner ( new FileReader(filename) );
while (input.hasNextLine()) { /* * Fill-in */ System.out.println(input.nextLine()); // Comment this line out } input.close(); } catch ( NoSuchElementException e){ System.out.println(e);
} catch (FileNotFoundException e) { System.out.println(e); } return output; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
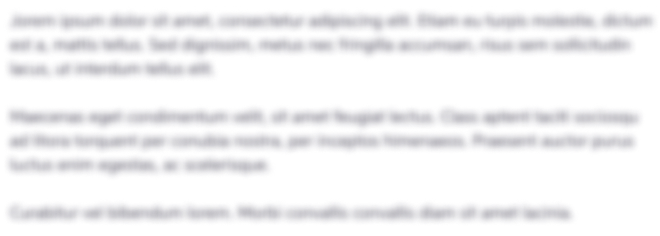
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started