Question
Stringvector.h namespace lab2 { class stringVector { private: std::string *data; unsigned length; unsigned allocated_length; public: stringVector(); virtual ~stringVector(); unsigned size()const; unsigned capacity()const; void reserve(unsigned new_size);
Stringvector.h
namespace lab2 { class stringVector { private: std::string *data; unsigned length; unsigned allocated_length; public: stringVector(); virtual ~stringVector(); unsigned size()const; unsigned capacity()const; void reserve(unsigned new_size); bool empty()const; void append(std::string new_data); void swap(unsigned pos1, unsigned pos2); stringVector &operator=(stringVector const &rhs); std::string &operator[](unsigned position); void sort(); }; }
______________________________________________________________________
Stringvector.cpp
#include "../inc/stringVector.h" #include#include namespace lab2 { stringVector::stringVector() { this->data = nullptr; length = 0; allocated_length = 0; } stringVector::~stringVector() { delete[] data; data = nullptr; } unsigned stringVector::size() const{ return length; } unsigned stringVector::capacity() const{ return allocated_length; } void stringVector::reserve(unsigned new_size) { std::string *temp = new std::string[new_size]; allocated_length = new_size; if (length > allocated_length) { length = allocated_length; } for (int i = 0; i < length; i++) { temp[i] = data[i]; } delete []data; data = temp; } bool stringVector::empty() const{ return (length == 0); } void stringVector::append(std::string new_data) { if (length == allocated_length) { reserve(length + 1); allocated_length = length + 1; } data[length] = new_data; length++; } void stringVector::swap(unsigned pos1, unsigned pos2) { std::string string1; if((pos1 >= length) || (pos2 >= length)){ std::cout << "Index is out of bounds" << std::endl; return; } string1 = data[pos1]; data[pos1] = data[pos2]; data[pos2] = string1; } stringVector &stringVector::operator=(stringVector const &rhs) { if (this == &rhs) { return *this; } length = rhs.length; data = new std::string[length]; allocated_length = rhs.allocated_length; for (int i = 0; i < length; i++) { data[i] = rhs.data[i]; } return *this; } std::string &stringVector::operator[](unsigned position) { if (position + 1 > length) { throw std::out_of_range("Position out of range"); } return data[position]; } void stringVector::sort() { for (int i = (length - 1); i > 0; i--) { for (int j = 0; j < i; j++) { if (data[j].compare(data[j + 1]) > 0) { swap(j, j+1); } } } } }
_______________________________________________________________________
Fifo.h
#include "stringVector.h" namespace lab3 { class fifo { lab2::stringVector fifo_storage; unsigned front_index; unsigned back_index; public: fifo(); //Default constructor. Reserve 100 spaces in lifo_storage explicit fifo(std::string input_string); //Create new fifo from string input fifo(const fifo &original); //Copy constructor virtual ~fifo(); //Destructor fifo &operator=(const fifo &right); //Assignment operator bool is_empty(); // Return true if the fifo is empty and false if it is not int size(); // Return the size of the fifo std::string top(); // Return the front string of the fifo. void enqueue(std::string input); // Add input string to the back of the fifo void dequeue(); // Remove the front string from the fifo }; }
_________________________________________________________________________________
fifo.cpp
#include "fifo.h" namespace lab3{ fifo::fifo() { fifo_storage.reserve(100); front_index = 0; back_index = 0; } fifo::fifo(std::string input_string) { fifo_storage.append(input_string); } fifo::fifo(const fifo &original) { back_index = original.back_index; front_index = original.front_index; } fifo::~fifo() { } fifo &fifo::operator=(const fifo &right) { if (this == &right) { return *this; } front_index = right.front_index; back_index = right.back_index; fifo_storage = right.fifo_storage; return *this; } bool fifo::is_empty(){ return !size(); } int fifo::size(){ return (back_index - front_index); } std::string fifo::top(){ return fifo_storage[front_index]; } void fifo::enqueue(std::string input) { fifo_storage.append(input); if (fifo_storage.capacity() <= back_index) { fifo_storage.reserve(fifo_storage.capacity() + 1); } back_index++; } void fifo::dequeue() { front_index++; if (front_index == back_index) { front_index = 0; back_index = 0; } } }
____________________________________________________________________________
lifo.h
#include "stringVector.h" namespace lab3 { class lifo { lab2::stringVector lifo_storage; unsigned index; public: lifo(); //Default constructor. Reserve 100 spaces in lifo_storage explicit lifo(std::string input_string); //Create new lifo from string input lifo(const lifo &original); //Copy constructor virtual ~lifo(); //Destructor lifo &operator=(const lifo &right); //Assignment operator bool is_empty(); // Return true if the lifo is empty and false if it is not int size(); // Return the size of the lifo std::string top(); // Return the top of the lifo. void push(std::string input); // Add input string to the top of the string void pop(); // Remove the top string from the lifo }; } #endif
_____________________________________________________________________________
lifo.cpp
#include "lifo.h" namespace lab3{ lifo::lifo() { lifo_storage.reserve(100); index = 0; } lifo::lifo(std::string input_string) { lifo_storage.reserve(100); lifo_storage.append(input_string); index = 1; } lifo::lifo(const lifo &original) { lifo_storage = original.lifo_storage; index = original.index; } lifo::~lifo() { } lifo &lifo::operator=(const lifo &right) { index = right.index; lifo_storage = right.lifo_storage; return *this; } bool lifo::is_empty(){ return !size(); } int lifo::size(){ return index; } std::string lifo::top(){ return lifo_storage[index - 1]; } void lifo::push(std::string input) { if (lifo_storage.size() == index) { lifo_storage.append(input); } else lifo_storage[index] = input; index++; } void lifo::pop() { if (index > 0) { lifo_storage[index - 1] = ""; index--; } } }
________________________________________________________________________________
calculator.h
#include "lifo.h" #include "fifo.h" #include "expressionstream.h" namespace lab4{ class calculator{ lab3::fifo infix_expression; lab3::fifo postfix_expression; void parse_to_infix(std::string &input_expression); //PRIVATE function used for converting input string into infix notation void convert_to_postfix(lab3::fifo infix_expression); //PRIVATE function used for converting infix FIFO to postfix public: calculator(); //Default constructor calculator(std::string &input_expression); // Constructor that converts input_expression to infix and postfix upon creation friend std::istream& operator>>(std::istream& stream, calculator& RHS); //Store the infix and postfix expression in calculator int calculate(); //Return the calculation of the postfix expression friend std::ostream& operator<<(std::ostream& stream, calculator& RHS); //Stream out overload. Should return in the format "Infix: #,#,#,# Postfix: #,#,#,#" }; } #endif
______________________________________________________________________________________
calculator.cpp
#include#include "calculator.h" #include namespace lab4 { void calculator::parse_to_infix(std::string &input_expression) { } void calculator::convert_to_postfix(lab3::fifo infix_expression) { } calculator::calculator() { } calculator::calculator(std::string &input_expression) { } std::istream &operator>>(std::istream &stream, calculator &RHS) { } int lab4::calculator::calculate() { } std::ostream &operator<<(std::ostream &stream, calculator &RHS) { } // AUXILIARY FUNCTIONS bool is_number(std::string input_string); bool is_operator(std::string input_string); int get_number(std::string input_string); std::string get_operator(std::string input_string); int operator_priority(std::string operator_in); }
______________________________________________________________________________
Please help complete the calculator.cpp using the stringvector, fifo, and lifo functions in c++.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
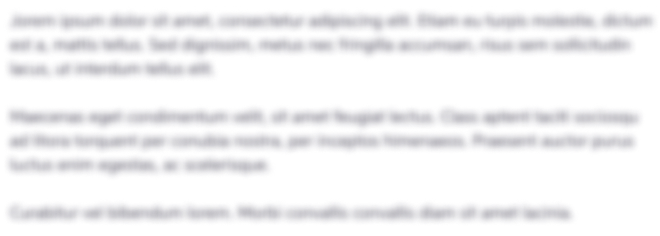
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started