Question
Struggling with getting my could to test out correctly and I'm not sure what I'm doing wrong: My Code: import static org.junit.jupiter.api.Assertions.*; import org.junit.jupiter.api.Test; class
Struggling with getting my could to test out correctly and I'm not sure what I'm doing wrong:
My Code:
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
class ClassTester {
@Test
void test() {
Traveler t = new Traveler();
Traveler t2 = new Traveler("Jay", Location.EARTH);
assertEquals(t2.current,Location.EARTH);
SpaceShip s = new SpaceShip();
s.setCurrent(Location.EARTH);
s.setCapacity(5);
s.setDestination(Location.RING);
s.setName("Millennium Falcon");
System.out.println("Ship: " + s.getName() + " Current Loc: " + s.getCurrent() + " Destination Loc: "+ s.getDestination() + " Number of Passengers" + s.getCapacity());
SpaceShip s2 = new SpaceShip("Millennium Falcon", Location.EARTH, Location.MARS, 5);
assertEquals(s2.current, s2.destination);
}
}
public enum Location
{
EARTH, BELT, MARS, RING
}
public class SpaceShip
{
private String name;
private int capacity;
private int numOfTravelers;
Traveler travelers[];
Location destination;
Location current;
public SpaceShip()
{
this.name = null;
this.current = null;
this.destination = null;
this.capacity = 10;
this.travelers= new Traveler[capacity];
}
public SpaceShip(String name, Location current, Location destination,
int capacity)
{
this.name = name;
this.current = current;
this.destination = destination;
this.capacity = capacity;
this.travelers= new Traveler[capacity];
}
public void setName(String name)
{
this.name = name;
}
public void setCurrent(Location current)
{
this.current = current;
}
public void setDestination(Location destination)
{
this.destination = destination;
}
public void setCapacity(int capacity)
{
this.capacity = capacity;
}
public String getName()
{
return name;
}
public int getCapacity()
{
return capacity;
}
public Location getCurrent()
{
return current;
}
public Location getDestination()
{
return destination;
}
boolean board(Traveler t)
{
//check for traveler on ship
isOnBoard(t);
if(numOfTravelers < capacity - 1)
{
this.travelers[numOfTravelers] = t;
numOfTravelers++;
return true;//if boarding is successful
}else{
return false;
}
}
boolean leave(Traveler t)
{
//If traveler is on-board, in the array, remove them and return true.
//2. If not on-board, do nothing and return false.
boolean check = false;
for(int i = 0; i < numOfTravelers; i++)
{
if(t.getID() == travelers[i].getID())
{
travelers[i] = null;
numOfTravelers--;
check = true;
}
}
return check;
}
boolean isOnBoard(Traveler t)
{
for(int i = 0; i < numOfTravelers; i++)
{
if(t.getID() == travelers[i].getID())
{
return true;
}
}
return false;
}
void move()
{
/*moves the ship to its destination
1. sets the ships current location to its destination.
2. sets the destination to null
3. sets all travelers locations to the current location of the spaceship
4. removes all travelers from the spaceship (array)
*/
}
public String toString()
{
return "name"; //place holder
}
}
public class Traveler
{
public static int nextIDNum = 0;
private String name;
private int id;
Location current;
Traveler()
{
this.name = null;
this.current = null;
this.id = nextIDNum;
}
Traveler(String name, Location location)
{
this.name = name;
this.current = location;
this.id = nextIDNum;
}
public void setName(String name)
{
this.name = name;
}
public void setLocation(Location location)
{
this.current = location;
}
public String getName()
{
return this.name;
}
public int getID()
{
return this.id;
}
public String toString()
{
return "Traveler[name = " + name + "id = " + id + "current = " + current;
}
}
Overview
You will be creating a the start of replay system that allows replaying events from a SciFi series. This project specifically focuses on using multiple classes and is therefore larger than the previous project, please try to plan accordingly.
Program Requirements
Location class - the class for identifying the four Locations:
The location are EARTH, BELT, MARS, RING.
Instance Variables
name - the name of the Spaceship. ii. capacity maximum number of travelers allowed on the spaceship
current current location of the spaceship
numOfTravelers actual number of Travelers on spaceship, partially filled array value initialize to zero.
travelers array that contains Traveler Objects that have boarded the spaceship vi. destination destination location of the spaceship
Constructors
public SpaceShip() - default constructor setting name, current and destination to null, capacity to 10 and creates the travelers array and sets size to capacity.
public SpaceShip(String name, Location current, Location destination, int capacity)
1. sets instance variables to matching parameter value
2. creates the travelers array and sets size to capacity.
Methods
Accessor methods for all instance variables as describe in UML class diagram above. (No accessor or mutator for collections such as Arrays!!!!)
No mutator method for numOfTravelers as this is our partially filled array value. iii. boolean board(Traveler t) travelers board the spaceshipIf spaceship is not full (at capacity) and the traveler is not already on board
add traveler to array
increment numOfTravelers
return true.
If spaceship is full or traveler already on board, return false.
static Variable - int nextIDNum - starts at 0 and is used to generate the next Travelers id. b. Instance Variables
name - the name of the traveler. ii. id - id number of traveler
iii. current current location of the traveler
c. Constructors
public Traveler() default constructor setting name and current to null and the id to the next id number
public Traveler(String name, Location l)
Sets name and location to parameter value
Sets id instance variable to next id number.
d. Methods
set/get methods according to the diagram
Remember in setId method use nextIDNum to determine new ID number.
public String toString()
returns a nicely formatted String (see below) that represents the state of the object (the value of the instance variables)
Traveler [name=Klaes Ashford, id=3, current=BELT]
Instance Variables:
name - the name of the Spaceship. ii. capacity maximum number of travelers allowed on the spaceship
current current location of the spaceship
numOfTravelers actual number of Travelers on spaceship, partially filled array value initialize to zero.
travelers array that contains Traveler Objects that have boarded the spaceship vi. destination destination location of the spaceship
Constructors
public SpaceShip() - default constructor setting name, current and destination to null, capacity to 10 and creates the travelers array and sets size to capacity.
public SpaceShip(String name, Location current, Location destination, int capacity) 1. sets instance variables to matching parameter value
2. creates the travelers array and sets size to capacity.
Methods
Accessor methods for all instance variables as describe in UML class diagram above. (No accessor or mutator for collections such as Arrays!!!!)
No mutator method for numOfTravelers as this is our partially filled array value. iii. boolean board(Traveler t) travelers board the spaceshipIf spaceship is not full (at capacity) and the traveler is not already on board
add traveler to array
increment numOfTravelers
return true.
If spaceship is full or traveler already on board, return false.
Travelers are exclusively identified by their ID!
iv. boolean leave(Traveler t) travelers leave the spaceship
If traveler is on-board, in the array, remove them and return true.
If not on-board, do nothing and return false.
v. boolean isOnBoard(Traveler t) checks if a traveler is on-board.
1. returns true if passenger on-board 2. returns false is passenger not on-board.
3. Called a Predicate method (Hint may be used in others methods above to keep code modular)
vi. void move()- moves the ship to its destination
sets the ships current location to its destination.
sets the destination to null
sets all travelers locations to the current location of the spaceship
removes all travelers from the spaceship (array)
vii. public String toString()
returns a nicely formatted String (see below) that represents the state of the object (the value of the instance variables)
Spaceship [name=Behemoth, current=RING, destination=null, capacity=20, passengers=0]
SpaceShipTester class with a main method:
A text-based driver program that performs the following steps:
It asks for four Travelers and two StarShips (Part 1, below)
It executes a text-menu with the following options (Parts 2-4 below)
Add traveler to a Spaceship
Search for traveler on a Spaceship
Move a spaceship to a new location
Exit the program
Step by Step Solution
There are 3 Steps involved in it
Step: 1
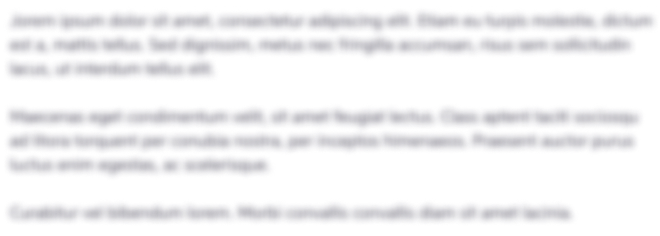
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started