Question
Stuck writing the code for this assignment. Any help is appreciated! C++ Programming: Inheritance and Operator Overloading Assignment Instructions Overview The objective of this assignment
Stuck writing the code for this assignment. Any help is appreciated!
C++ Programming: Inheritance and Operator Overloading Assignment Instructions
Overview
The objective of this assignment is to demonstrate an ability to implement inheritance, composition, and overloaded operators in a program. This program leverages many of the concepts that you have learned in this class and combines them into a professional-style program.
There are some helpful links at the bottom of the instructions.
Instructions
You are working in the IT department for a corporation and have been tasked with developing an application to manage the process of assigning people to a team. This process involves creating a team with a name and a purpose, the assignment of people in the team, and the assignment of a person to lead the team.
Classes are getting to be more realistic with each programming assignment. This assignment includes the valuable aspect of inheritance to facilitate the reuse of code and operator overloading to allow the usage of familiar operators tailored specifically to the Person, TeamMember, and Leader classes. Composition is also demonstrated in the use of a Team class that contains objects of the TeamMember and Leader classes.
Ask the professor questions early if you need help with the assignment.
You will need to create several classes to maintain this information: Team, Person, TeamMember, Leader, and Date.
The characteristics of TeamMember and Leader are shown below:
TeamMember: Leader:
ID (string) ID (string)
First Name (string) First Name (string)
Last Name (string) Last Name (string)
Birthdate (Date) Birthdate (Date)
Department (string) Division (string)
Date hired (Date) Date promoted (Date)
Position (string) (i.e. Programmer, Writer, etc.) Title (string)
SkillLevel (string) (Entry Level, Skilled, Master) Last team lead (string)
Last performance rating (double) (1.0-5.0) Annual salary (double)
Several of the data members above require the use of dates. Strings will not be acceptable substitutes for date fields.
C++ does not have a built-in data type for Date. Use the provided Date Class below for your Lab, as one of the grading criteria is that you used this class correctly. (Tip-practice with this in a separate program.) Cite your source.
Date class:
#pragma once
#include
#include
#include
class Date{
friend std::ostream& operator<<(std::ostream&, Date);
public:
Date(intd=0, int m=0, int yyyy=0) {
setDate(d, m, yyyy);
}
~Date() {}
void setDate(int d, int m, int yyyy){
day = d;
month = m;
year = yyyy;
}
private:
int day;
int month;
int year;
};
std::ostream& operator<<(std::ostream& output, Dated){
output<< d.month << "/"<< d.day << "/"<< d.year;
returnoutput;
}
Person class (base class)
The Person class should contain data members that are common to both TeamMembers and Leaders in the above list.
Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor.
TeamMember class and Leader class. (derived classes)
Both theTeamMemberandLeaderclasses must be derived from thePersonclass.
Each must have the member variables shown above that are unique to each class. The data members that are common to both classes must be inherited from thePersonclass.
The constructor in the derived class must call the constructor in the base class correctly through the constructor definition.
Write member functions to store and retrieve information in the appropriate member variables. Be sure to include a constructor and destructor in each derived class.
In each derived class, the<< operator should be overloadedto output, neatly formatted, all of the information associated with a team member or leader.
Here is an example of an operator overload.
class MyClass
{
friend std::ostream& operator<<(std::ostream& output, MyClass b);
public:
...
...
private:
int ID;
std::string FirstName;
std::string LastName;
...
...
std::ostream& operator<<(std::ostream& output, MyClass s)
{
output << std::fixed;
output << std::setw(30) << std::left << "ID: " << s.getID() << std::endl;
output << std::setw(30) << std::left << "Name: " << s.getFirstName() + " " + s.getLastName() << std::endl;
return output;
}
There are links to other examples of operator overloading located at the bottom of the instructions.
Team class
TheTeamclass has anamedata member (i.e. a Team's name might be "Project ABC").
TheTeamclass has apurposedata member (i.e. a Team's purpose might be "Feasibility study of project ABC").
The class contains aLeader objectthat represents the employee assigned to lead the team.
The class contains avector of TeamMember objectsthat represent the employees assigned to the team.
The class has an integer variable,teamSize, that represents the number of team members the team needs.
TheTeamclass should support operations to assign a leader to the team, assign team members to the team, and list all of the team information, including its name, purpose, leader, and team members assigned to it.Note: No data entry is done inside the class.
You will create a function namedsortTeamMembers()that uses thesortfunction to sort this vector. Thesortfunction is part of the <algorithm>library.
Note: The standard sort uses the first element in the vector to sort on. Make sure your data members are in the exact order listed on page 1.
Main()
You should write amain() function that begins by prompting the user for the name, purpose, and size of the team:
Figure 1-Enter Team Information
It should then present a menu to the user that looks like this:
Figure 2-Main Menu
"T"should allow the user to create a TeamMember record and assign the TeamMember to the Team.
"A" should allow the user to create a Leader record and assign the Leader member to the team as the leader.
"L"should list ALL of the team information, including the information about each TeamMember assigned to the team, (sorted by ID number), as well as all of the information about the Leader assigned to lead the team. The user should be able to create and assign only ONE Leader to the team, but create and assign as many TeamMembers to the team as the size allows.
"Q" should allow the user to exit the application.
Note: The user should be able to add repeatedly (until the team capacity is reached) and to list repeatedly until he or she selects "Q" to quit.
Below are some examples of the information that needs to be entered by the user:
Create and assign a leader for the team:
Figure 3-Enter Team Leader Information
Create and assign a team member to the team:
Figure 4-Enter Team Member Information
List - Team Information if neither team members nor a leader has been assigned to the team:
Figure 5-Team Information w/ No Leader or Team Members Assigned
List - Team Information with the leader but no team members:
Figure 6-Display Team Information w/ No Team Members
List - Team Information if Team Members have been assigned but no leader has been assigned:
Figure 7-Team Information w/ No Leader Assigned
List - Team Information after assigning Leader and three Team Members:
Figure 8-Team Information After All Members Assigned
Notice that the Team Members are sorted by IDs in the output above. This happens when ID is the first data member in the Person Class, which makes it the first element in each entry in the vector.
Output if the team already has a leader assigned:
Figure 9-Team Leader Already Assigned
Output if the team has all members and the user tries to add another member:
Figure 10-All Team Members Already Assigned
Step by Step Solution
There are 3 Steps involved in it
Step: 1
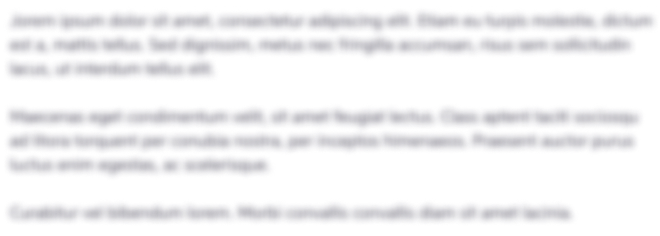
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started