Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Student Roster using Linked List For this assignment, you are tasked with implementing a student roster using a sorted linked list. Each student should include
Student Roster using Linked List
For this assignment, you are tasked with implementing a student roster using a sorted linked list. Each student should include the student's name, SSN and grade. The linked list data structure will be utilized to store all student records.
The test program is expected to read data from an input file and then insert each student record into the sorted list based on SSN Additionally, students should attempt to delete specific students from the list. After each insert or delete operation, it is required to print the entire student list.
StudentLinkedListh
struct StudentData
string name;
int ssn;
char grade;
;
struct StudentNode
StudentData student;
StudentNode next;
;
class StudentLinkedList
public:
StudentLinkedList;
void addStudentStudentData student;
void deleteStudentint;
void printListostream&;
private:
StudentNode head;
;
StudentLinkedList::StudentLinkedList
head NULL;
Implement all methods except default constructor.
Drivercpp
Underlined provides an explanation of the methods:
pts addStudent: read each student from file, insert into linked list.
pts PrintList: Print all students on the screen.
pts deleteStudent: Delete a student based on the given Social Security Number SSN
#include StudentLinkedListh
int main
StudentLinkedList slist;
StudentData student;
Read each student from the file and insert into linked list using while loop
slist.printListcout;
cout endl;
Deleting one student from the student linked list!!!
cout Enter SSN to be deleted: ;
int sno;
cin sno;
slist.deleteStudentsno;
slist.printListcout;
return ;
studentstxt: Download this file from Canvas.
Betsy A
Sam Spade B
Annie Smith A
Thomas J Jones A
Allan Adaire C
Jennifer Smallville A
Note: Each value name ssn and grade is separated by tab key t Sample output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
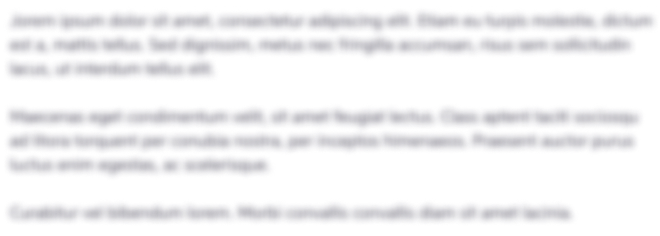
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started