Question
Study the following code and use for a Web server program. /* This program listens to TCP socket connections on port 8000. For each connecting
Study the following code and use for a Web server program.
/* This program listens to TCP socket connections on port 8000. For each connecting client, the program prints the data it receives and then returns an HTTP response message that contains the word 'hi' in an H1 element. Assuming this file is named 'server.js', run the following from the command line to start the server. node server.js To test, go to http://localhost:8000/ in a browser. */ const net = require('net'); const server = net.createServer(); server.listen(8000, () => { console.log('Open the following URL in browser:'); console.log('http://localhost:8000/'); console.log(''); }); /* The following string is the HTTP response message returned to connecting clients (browsers). The structure of an HTTP response message is as follows: status line general headers blank line message data In an HTTP response message, a blank line ends the msg header and starts the msg body. */ const response = 'HTTP/1.1 200 OK' + ' ' + // status line 'Content-length: 11' + ' ' + // general header 'Content-type: text/html' + ' ' + // general header ' ' + // blank line '
hi
'; // message body (11 bytes) server.on('connection', (socket) => { console.log('Something connected to me.'); socket.on('data', (data) => { console.log('Received from client: ' + data); socket.end(response); // Send response and close connection. }); });
Instructions
Create a folder in your repository named http to store the files for this assignment.
server.js
In the http folder, create a file named server.js with the code given above. Run this program as follows.
node server.js
Go to http://localhost:8000/ to test the server.
Examine the HTTP request headers received by your browser. See one of the following based on the browser you use.
How to view HTTP headers in Google Chrome?
In Firefox, how do I see HTTP request headers? (where in web console?)
How to inspect HTTP requests in Safari 8 or later
client.js
Use Node.js to create a TCP client named client.js that connects to the TCP server given above, sends the following HTTP request message for the server's root resource ('/') and prints the response message from the server.
GET / HTTP/1.1 Host: localhost
Note that in the client's HTTP request message above, the HTTP request line is terminated by a carriage return ( ) line feed ( ) combination. Also note that a blank line follows the request line, hence the additional carriage return line feed pair.
Use the Node.js net module to solve this problem; do not use the http module. You'll need the following in your code to access the net module.
const net = require('net');
You will need to read the Node.js documentation to solve this problem. In particular, you will need to read about the net module. One way to do this is by calling net.createConnection(), which returns a socket object. Here is what this would look like to connect to your server listening to port 8000 on the localhost. (The term localhost refers to the computer the program is running on.)
const socket = net.createConnection({ host: 'localhost', port: 8000 });
The above code creates a client TCP socket that will be connected to the running server. The connection is not made immediately when the above function runs but is made after the function returns. For this reason, you need to register event handlers with the socket to monitor state changes and take appropriate actions. The first event that should occur is the connection event, which means the two-way communication channel (TCP) is established so that both sides may start sending and receiving data. In the HTTP protocol, after the two-way communication channel is established, the client should send the first message, called the HTTP request message. The following shows how this could be done.
socket.on('connect', () => { const req = 'GET / HTTP/1.1 ' + 'Host: localhost ' + ' '; socket.end(req); // Send req string and then close this side of the TCP socket. });
Node.js sockets emit events. To solve this problem, you can use the events named connect and data. To register handlers for these events, you call the on method of the socket object. The following shows how to print the data received from the server when the data event occurs.
socket.on('data', (chunk) => { console.log(chunk.toString('utf8')); });
It is possible for the data event to occur multiple times for a single connection, which is why we use the name chunk for the callback parameter in the above code. Also, the data received is raw bytes. We use the toString function of the chunk object to convert to a printable string. In Web programming the most common character encoding is UTF-8, which is the encoding used by default by our server. For this reason, in the above code, we call the toString function on the chunk object while specifying the UTF-8 character encoding to use.
To test your client program against the server program you'll need two terminal windows; one to run the server and another to run the client. Run the client as follows.
node client.js
problem: server2.js
Create an alternative server with filename server2.js based on the http module. For each request received, have the server send the following content.
const html = "
" + "405" + "
hi
" + "";
Also, specify a content type of text/html.
res.setHeader('Content-Type', 'text/html');
Note that the http server will automatically add a content length header for you but it will not automatically add the correct content type header. That's why we need to do this explicitly.
This is for LOCAL HOST
Step by Step Solution
There are 3 Steps involved in it
Step: 1
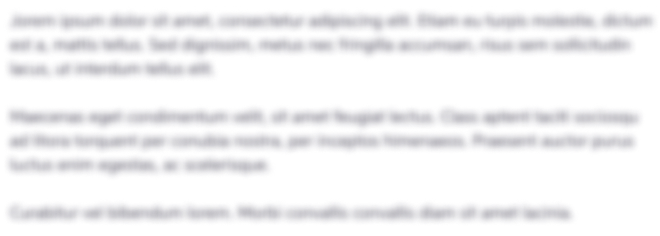
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started