Question
Subject : C++ Add a copy constructor, an assignment (=) operator overload, and an equality (==) operator overload method to the Stack class. Add exception
Subject : C++
Add a copy constructor, an assignment (=) operator overload, and an equality (==) operator overload method to the Stack class. Add exception handling (both try/catch and throw) to the Stack class as necessary. Avoid code replication by creating helper method(s) and utilizing existing class methods where possible.
The following test code will be added at the beginning of main() during the grading process:
{ // Read Top Element / Pop from an empty stack - catch the exceptions Stack stack0; try { stack0.readTop(); } catch (exception &e) { cerr << "Caught: " << e.what() << endl; cerr << "Type: " << typeid(e).name() << endl; } try { stack0.pop(); } catch (exception &e) { cerr << "Caught: " << e.what() << endl; cerr << "Type: " << typeid(e).name() << endl; }
Stack stack1(stack0); // test initialization with an empty stack stack1.push(1); stack1.push(2); stack1.push(3); stack1.push(4);
cout << boolalpha << "stack1: " << stack1 << ", equal to stack1? " << (stack1 == stack1) << endl; // Test self equality stack1 = stack1; // Test the = operator on self cout << "stack1: " << stack1 << endl;
{ // Test the copy constructor Stack stack2(stack1); cout << "stack2: " << stack2 << ", equal to stack1? " << (stack1 == stack2) << endl; } // Test the stack2 destructor
{ // Test the = operator Stack stack3; stack3.push(10); stack3.push(11); cout << "stack3: " << stack3 << ", equal to stack1? " << (stack1 == stack3) << endl; stack3 = stack1; cout << "stack3: " << stack3 << ", equal to stack1? " << (stack1 == stack3) << endl; } // Test the stack3 destructor cout << "stack1: " << stack1 << endl; // Has stack 1 changed? }
STACK.cpp
#include "stdafx.h" #include "Stack.h" #include
using namespace std;
// Constructor Stack::Stack() { top = nullptr; numNodes = 0; }
Stack::Stack(const Stack &stack) // doing this right? {
}
void Stack::push(int newPayload) { top = new Node(newPayload, top); numNodes++; }
int Stack::pop() { assert(top != nullptr); // Make sure stack isnt empty
int returnVal = top->getPayload();
Node *tempPtr = top->getNext();
delete top; numNodes--; top = temp;
return(returnVal); }
ostream &operator << (ostream &output, const Stack &displayStack) { Node *current; current = displayStack.top;
while (current != nullptr) { output << current->getPayload(); current = current->getNext(); }
return output; }
// Clear Stack void Stack::clear() { while (top != nullptr) { pop(); } }
int Stack::readTop() const { assert(top != nullptr); return (top->getPayload()); }
// retrieve numbers of elements unsigned int Stack::getNumElements() const { return (numNodes); }
void Stack::displayNodesReverse(ostream &output, Node *current) const { if (current != nullptr) { displayReverse(current->getNext()); output << *current; if (current != top) { output << " , "; } } }
//Overload operator ostream& operator <<(ostream& output, const Stack &stack) { stack.displayNodesReverse(output, stack.top); return(output); }
// Destructor Stack::~Stack() { clear(); }
STACK.h
#pragma once #include "Node.h" #include
using namespace std;
class Stack { private: Node *top; // Pointer to the top element unsigned int numNodes; void displayNodesHelper(Node *current) const;
public: //Constructor Stack();
Stack(const Stack& stack); // push method void push(int newPayload);
// pop method int pop();
// ACCESSORS // top accesor int readTop() const;
// retrieve numbers of elements unsigned int getNumElements() const; // make sure theres no disks void clear();
// Display stack void reversePrint() const; // output stack friend ostream &operator << (ostream &output, const Stack &displayStack);
// Deconstructor ~Stack();
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
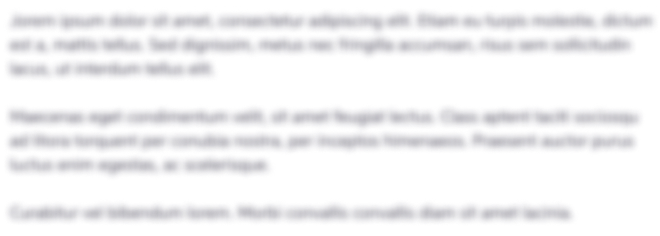
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started