Question
Sudoku is a popular logic-based number placement game. The aim of the puzzle is to enter a numerical digit from 1 through 9 in each
Sudoku is a popular logic-based number placement game. The aim of the puzzle is to enter a numerical digit from 1 through 9 in each cell of a 99 grid made up of 33 sub grids (called boxes). The game starts with various digits given in some cells (the givens). When finished, each row, column, and 3x3 regions must contain all 9 digits with no repetition.
There are 3 simple rules you must follow when playing Sudoku. Fill in the grid so that:
1. every row
2. every column
3. every 3x3 box contains the digits 1 through 9.
For this project, you will implement a class Sudoku
which has the following member functions:
1. Sudoku(std::string): The parameterized constructor accepts a filename (string) as input. The file contains an unsolved sudoku puzzle. This constructor initializes the sudoku grid from the data contained in the input file. Please see the section Sudoku File Format for the appropriate format.
2. Save(std::string): Accepts an output filename as argument and saves the sudoku grid to the output file in the format described in the section Sudoku File Format.
3. Overloaded<< operator for printing out the sudoku grid.
If A is a Sudoku object,std::cout << A;should print the content of A in the format specified in section Sudoku File Format.
4. Overloaded( ) operator for accessing the grid values at a specified row, column pair
If A is a Sudoku object,A(2, 3);should return the grid value of A at row = 2 and column = 3.
If the grid value is not a number between 1 and 9, it should return 0.
The row and the column indices should be within the range of 0 and 8. Otherwise, it shouldthrow an OutOfBounds exception.
5. Solve() for solving the sudoku puzzle
If A is a Sudoku object, A.Solve(); solves the puzzle resulting in a complete and valid grid forA.
You can assume the input puzzle is always solvable.
Sudoku File Format:
The following is an example sudoku input file (say 0 sudokugrid.txt).
*****329*
*865*****
*2***1***
**37*51**
9*******8
**29*83**
***4***8*
*****647*
*471*****
Overall, its a 99 grid containing numbers (between 1 and 9) and the character. It is unsolved and thes indicate the cells that need to be filled correctly. After solving, the above sudoku grid should look like
the following:
154873296
386592714
729641835
863725149
975314628
412968357
631457982
598236471
247189563
The project needs a header file(.hpp) to declare everything for the class and a .cpp with the definitions
Step by Step Solution
There are 3 Steps involved in it
Step: 1
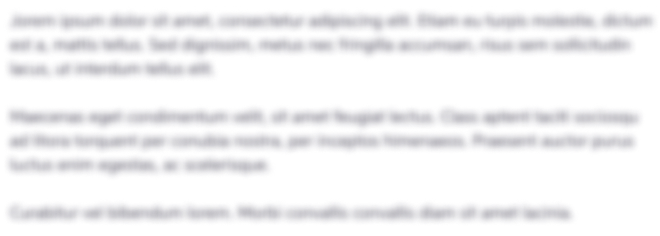
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started