Question
Support Vector Machine Bob thinks it is clearly not a regression problem, but a classification problem. He thinks that we can change it into a
Support Vector Machine
Bob thinks it is clearly not a regression problem, but a classification problem. He thinks that we can change it into a binary classification and use the support vector machine we learned in Lecture 4 to solve the problem. In order to do so, he suggests that we can build an one vs. rest model for every digit. For example, classifying the digits into two classes: 0 and not 0.
Bob wrote a function run_svm_one_vs_rest_on_MNIST where he changed the labels of digits 1-9 to 1 and keeps the label 0 for digit 0. He also found that sklearn package contains an SVM model that you can use directly. He gave you the link to this model and hopes you can tell him how to use that.
You will be working in the file part1/svm.py in this problem
Important: For this problem, you will need to use the scikit-learn library. If you don't have it, install it using pip install sklearn
My Question: What is the solution for "Binary classification error" and "Multiclass SVM Error" and how do I get them?
One vs. Rest SVM 5.0/5.0 Punkte (benotet) Use the sklearn package and build the SVM model on your local machine. Use random_state = 0 , C=0.1 and default values for other parameters. Available Functions: You have access to the sklearn's implementation of the linear SVM as Linearsvc; No need to import anything. 1 def one_vs_rest_svm (train_x, train_y, test_x): 2 3 clf = LinearSVC(random_state=0, C=0.1) 4 5 6 clf.fit(train_x, train_y) pred_test_y = clf.predict(test_x) 8 9 return pred_test_y 10 raise NotImplementedError 11 12 Klicken Sie die ESC- und TAP-Taste oder auerhalb des Codeeditors, um zu beenden. Richtig Test results See full output CORRECT See full output Binary classification error 0.0/5.0 Punkte (benotet) Report the test error by running run_svm_one_vs_rest_on_MNIST : Error 0.7698 X Multiclass SVM 5.0/5.0 Punkte (benotet) In fact, sklearn already implements a multiclass SVM with a one-vs-rest strategy. Use LinearSvc to build a multiclass SVM model Available Functions: You have access to the sklearn's implementation of the linear SVM as LinearSvc; No need to import anything. 1 def multi_class_svm(train_x, train_y, test_x): 2 3 Trains a linear SVM for multiclass classifciation using a one-vs-rest strategy 4 5 Args : 6 train_x (n, d) Numpy array (n datapoints each with d features) 7 train_y - (n. ) Numpy array containing the labels (int) for each training data point test_x - (m, d) Numpy array (m datapoints each with d features) Returns: 10 pred_test_y - (m) Numpy array containing the labels (int) for each test data point 11 12 clf = LinearSvc (random_state=0, C=0.1) 13 clf.fit(train_x, train_y) 14 pred_testy = clf.predict(test_x) 15 return pred_test_y 16. naics Not ImplementadError Klicken Sie die ESC- und TAP-Taste oder auerhalb des Codeeditors, um zu beenden. 8 9 Richtig Multiclass SVM error 0.0/5.0 Punkte (benotet) Report the overall test error by running run_multiclass_svm_on_MNIST . Error One vs. Rest SVM 5.0/5.0 Punkte (benotet) Use the sklearn package and build the SVM model on your local machine. Use random_state = 0 , C=0.1 and default values for other parameters. Available Functions: You have access to the sklearn's implementation of the linear SVM as Linearsvc; No need to import anything. 1 def one_vs_rest_svm (train_x, train_y, test_x): 2 3 clf = LinearSVC(random_state=0, C=0.1) 4 5 6 clf.fit(train_x, train_y) pred_test_y = clf.predict(test_x) 8 9 return pred_test_y 10 raise NotImplementedError 11 12 Klicken Sie die ESC- und TAP-Taste oder auerhalb des Codeeditors, um zu beenden. Richtig Test results See full output CORRECT See full output Binary classification error 0.0/5.0 Punkte (benotet) Report the test error by running run_svm_one_vs_rest_on_MNIST : Error 0.7698 X Multiclass SVM 5.0/5.0 Punkte (benotet) In fact, sklearn already implements a multiclass SVM with a one-vs-rest strategy. Use LinearSvc to build a multiclass SVM model Available Functions: You have access to the sklearn's implementation of the linear SVM as LinearSvc; No need to import anything. 1 def multi_class_svm(train_x, train_y, test_x): 2 3 Trains a linear SVM for multiclass classifciation using a one-vs-rest strategy 4 5 Args : 6 train_x (n, d) Numpy array (n datapoints each with d features) 7 train_y - (n. ) Numpy array containing the labels (int) for each training data point test_x - (m, d) Numpy array (m datapoints each with d features) Returns: 10 pred_test_y - (m) Numpy array containing the labels (int) for each test data point 11 12 clf = LinearSvc (random_state=0, C=0.1) 13 clf.fit(train_x, train_y) 14 pred_testy = clf.predict(test_x) 15 return pred_test_y 16. naics Not ImplementadError Klicken Sie die ESC- und TAP-Taste oder auerhalb des Codeeditors, um zu beenden. 8 9 Richtig Multiclass SVM error 0.0/5.0 Punkte (benotet) Report the overall test error by running run_multiclass_svm_on_MNIST . ErrorStep by Step Solution
There are 3 Steps involved in it
Step: 1
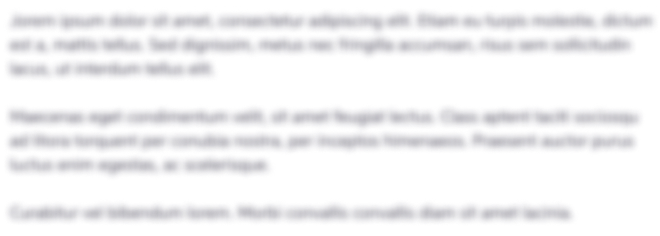
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started