Question
Suppose that over the years you collected some music CDs and vinyl records, so its time to keep track of your music. Your job is
Suppose that over the years you collected some music CDs and vinyl records, so its time to keep track of your music. Your job is to read a text file into memory that contains the music collection, so it can be displayed, searched, etc. To do this, I would like your program to use a user-controlled loop where the user can search for a specific album (CD or record), list all the albums stored in memory, add a new album (to memory only, not to the file), or quit. See below for file format details and sample output.
Input File Format
For this assignment, the file that your program will load contains a few details about the albums in your collection. Specifically,
- the file will contain the name of the album, the band name, the release date
- the type (CD, vinyl, tape, etc.)
- the album number. The album number is based on how many albums they have produced. If the album is the artists 3rd, for example, then the album number is 3.
Use a single line to store all the data about a single album. An example file will be provided with the assignment, but you may create your own. Please do not include headers, a file description, or other non-data information in the file.
Use commas to separate the data items on each line of the file. Like this:
Pork Soda,Primus,1993,CD,3
Vitalogy,Pearl Jam,1994,CD,3
Example Output (User Input in Bold):
Welcome to the music database program. What is the name of the album database file? notAFile.txt
notAFile.txt was not found. Type quit to exit the program.
What is the name of the album database file? CDsAndRecords.txt
Successfully loaded 5 albums.
Name Band Release Date Type Album number
---------------------------------------------------------------
Pork Soda Primus 1993 CD 3
Vitalogy Pearl Jam 1994 CD 3
What would you like to do? (S)earch for an album, (A)dd an album, (L)ist all albums, or (Q)uit? A
What is the name of the album? Houses of the Holy
What is the band name? Led Zeppelin
What is the release date? Nineteen Seventy Three
That is not a number. Please enter a date in numeric form.
What is the release date? 1973
What is the type of the album (CD, vinyl, tape, etc.)? vinyl
What is the album number? 5
Successfully added Houses of the Holy to the database.
What would you like to do? (S)earch for an album, (A)dd an album, (L)ist all albums, or (Q)uit? S
What is the name of the album? Soup
Information on the album Soup is as follows:
Band: Blind Melon, Release Date: 1994, Type: CD, Album number: 2.
What would you like to do? (S)earch for an album, (A)dd an album, (L)ist all albums, or (Q)uit? S
What is the name of the album? Briefcase Full of Blues
Error, unable to find Briefcase Full of Blues in the album database.
What would you like to do? (S)earch for an album, (A)dd an album, (L)ist all albums, or (Q)uit? L
Name Band Release Date Type Album number
---------------------------------------------------------------
Pork Soda Primus 1993 CD 3
Vitalogy Pearl Jam 1994 CD 3
Houses of the Holy Led Zeppelin 1973 vinyl 5
What would you like to do? (S)earch for an album, (A)dd an album, (L)ist all albums, or (Q)uit? Q
Terminating Program.
Programming Requirements
- Place your global constants, libraries, function prototypes and struct definition in a header file, perhaps called music.hpp. Place your main function in one source code file (main.cpp) and your function definitions in a separate source code file (functions.cpp perhaps).
- Create a makefile to compile your assignment using the make Linux utility.
- Dont use the string library or the vector library. Use the
library and c-strings (char arrays) to store strings. You may use a reasonable length for your c-strings, such as 128 or 256, declared as a global constant (remember not to use literals in your code for things like array size): const int strSize = 256; const int arraySize = 256; -
struct albumData {
char albumName[strSize];
char bandName[strSize];
int releaseDate;
char albumType[strSize];
int albumNumber;
};
char albumNames[arraySize][strSize];
char bandNames[arraySize][strSize]; int releaseDates[arraySize];
char albumTypes[arraySize][strSize]; int albumNumbers[arraySize];
Create your arrays or your array of structs in the main function. Do not use any global variables (constant globals are OK).
- Make sure you check for index out of bounds before adding new data to the arrays or array. When loading data, compare the index to the arraySize constant. If they are equal, there is no more room, so stop loading data. Suppose you use a variable called index to know where to place new data. Index will start out at zero, and then add 1 to move to the next index: index++; You can check if the array or arrays are out of room with an if statement:
if(index >= arraySize) // dont load the new data.
There are two functions where you need to do this check: loadData() and addAlbum(). See below.
- You must create at least three functions in addition to the main function. One of the functions must be to load the data from the file (loadData()), the second must be to search the arrays or array for the name of the album (searchData()), and the third will be to add a new album (addAlbum()). Place these three functions in a separate source code file (separate from the main function). You may create more than those three functions, but you must implement at least those three.
- Check to make sure that the input file is open before reading from it, using ifstream.is_open(). You may terminate the program if the file does not open, or you may ask the user to try a different file name. Make sure to close the input file before program termination.
- Make sure that you check for negative and bad data when reading number data from the user. You may assume that the input file is formatted correctly, so no error checking is necessary when reading from the file. There are two different ways to check for non-number input. The first method is to check for input failure, and the other is to use atoi(), found in the
library. Suppose you have a variable called releaseDate set up to take input from cin. After storing the input, use an if statement to check for input failure:
cout << "What is the release date? ";
cin >> releaseDate;
while(cin.fail() || releaseDate <= 0) {
cin.clear();
cin.ignore(MAX_CHAR, );
cout << "Input failure. Please enter a positive date in numeric format.";
.
}
The other way to check for non-number input is to use atoi(), which means ascii to int. If the input string cant be converted to a number, then atoi will return zero. So, check for input failure by checking the result for less than or equal to zero:
char dateString[strSize];
cout << "What is the release date? ";
cin.getline(dateString, strSize);
releaseDate = atoi(dateString);
while(releaseDate <= 0) {
cout << "Input failure. Please enter a positive date in numeric format.";
}
By the way, dont copy-and-paste any of this code from the document into your code file. If you do, you will have non-ASCII characters in your code file, which will cause errors.
Programming Strategies
Use the
Do not assume that the input file contains a certain number of albums, which means you should read from the file using an EOF-controlled loop.
Do not use regular cin or ifstream extraction (>>) to read data into any c-strings, because this is a potential security issue called buffer overflow. Use cin.getline() or inFile.getline() instead. These functions can take 2 or 3 arguments: the c-string, the number of characters to read, and the delimiter (optional). If you leave off the delimiter, the default is the newline (' '). The other version of ifstream.getline() allows you to set the delimiter. For example, the comma symbol:
albumFile.getline(name, strSize, ','); There is another method called get() to read data from an input stream. The difference between get() and getline() is that getline() removes the delimiter from the stream, whereas get() does not. So you will most likely want to use getline().
You can either create your ifstream object in main, or wait to create it in the load data function. Dont forget to close the file at the appropriate time. If you create it in main, then you must pass it as a reference parameter to the load data function. Similarly, you can ask the user for the name of the album to search for in main, or you can wait to ask in the search function. The arrays or struct array must be passed to loadData(), searchData() and addAlbum(), because the arrays or array must be declared in main. Suppose you use an array of structs to hold your data. Then the prototype for the load data function would be either:
int loadData (albumData albums[], ifstream & albumFile);
or:
int loadData (albumData albums[]);
The first version passes the file to the function, and the second one assumes that it will be created in the body of loadData().
If you use parallel arrays, you will have to send all of them to the functions:
int loadData (char albumNames[][strSize], char bandNames[][strSize], int releaseDates[], char albumTypes[][strSize], int albumNumbers[], ifstream & albumFile);
For the search function, you will need to send the current number of items in the array (size in the prototypes below), so that you will know when to stop the search loop. So, if you are using an array of structs, the prototype for the search function may be something like:
bool searchData (albumData albums[], char searchName[], int size);
or:
bool searchData (albumData albums[], int size);
For the multiple array version, you will have to pass all of them, similar to the loadData() example above.
Notice that the loadData prototypes return an int, so your main function will know how many albums were loaded. You will use this information to load a new album into the proper index or indices, and also to send to the search() function (as the size argument). Notice that search returns a bool. You can use the return value to signal whether the search was successful or unsuccessful. The loadData function may also be unsuccessful, in which case it could return an error code. Remember not to use literals, so set up a constant: const int ERROR = -1;
When testing your code for loadData(), it may appear that your program is loading the last line of the file twice. Whats really happening is that you are trying to read a line from the end of the file before the end-of-file marker has been reached. A common fix for this is to always read a new line as the last statement in a loop, and check for eof in the loop condition. This strategy may also require you to read data once before entering the loop. Another common way to check for end of file is to use a getline statement as the loop condition:
while (inFile.getline(myCString, STRSIZE)) // false if eof().
The last way to check for eof() is to use a peek() statement near the end of the loop: inFile.peek(). If peek() encounters the end of file marker, then inFile.eof() will return true.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
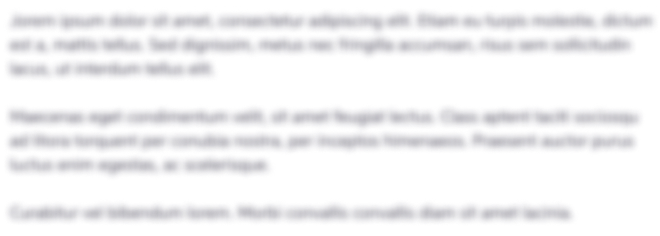
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started