Question
Suppose that we have the two classes below. Trace the execution of the main program in the Course class. public class Student { private String
Suppose that we have the two classes below. Trace the execution of the main program in the Course class.
public class Student
{
private String name;
private int exam1;
private int exam2;
private char grade;
public Student(String name, int exam1, int exam2)
{
this.name = name;
this.exam1 = exam1;
this.exam2 = exam2;
}
public char findGrade()
{
double avg = getAverage();
if (avg >=90.0)
return 'A';
else if (avg >= 80.0)
return 'B';
else if (avg >= 70.0)
return 'C';
else if (avg >= 40.0)
return 'D';
else
return 'F';
}
public String getName()
{
return this.name;
}
public double getAverage()
{
return (exam1 + exam2)/2.0;
}
public void setExam1(int exam1)
{
this.exam1 = exam1;
}
public void setExam2(int exam2)
{
this.exam2= exam2;
}
}
import java.util.ArrayList;
public class Course
{
public static void main(String[] args)
{
ArrayList
Student donald = new Student("Donald Duck", 75, 90);
Student goofy = new Student ("Goofy", 55, 40);
Student minnie = new Student("Minnie Mouse", 88, 95);
students.add(donald);
students.add(goofy);
students.add(minnie);
Student change = students.get(1);
change.setExam1(80);
}
}
Main stack frame
identifier | address | contents |
donald | 100 |
|
goofy | 101 |
|
minnie | 102 |
|
students | 103 |
|
change | 104 |
|
Heap
identifier | address | contens |
| 1000 |
|
| 1001 |
|
| 1002 |
|
| 1003 |
|
| 1004 |
|
| 1005 |
|
| 1006 |
|
| 1007 |
|
| 1008 |
|
| 1009 |
|
| 1010 |
|
| 1011 |
|
| 1012 |
|
| 1013 |
|
| 1014 |
|
| 1015 |
|
| 1016 |
|
| 1017 |
|
| 1018 |
|
| 1019 |
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
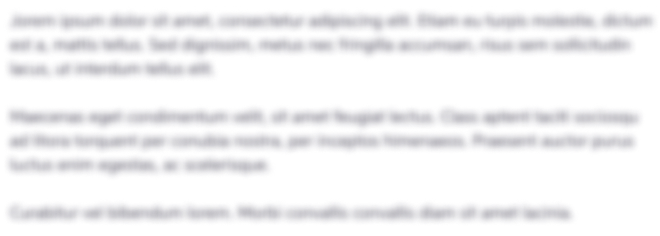
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started