Question
Task 01) Run the following code: void countDown(int num) { if (num == 0) // test System.out.println(Blastoff!); else { System.out.println(...); countDown(num-1); // recursive call }
Task 01) Run the following code:
void countDown(int num)
{
if (num == 0) // test
System.out.println("Blastoff!");
else {
System.out.println("...");
countDown(num-1); // recursive call
}
}
what is the output of this code?
- modify it so that it prints only the even numbers.
- what is the time complexity of this algorithm and why?
Task 02) Run the following code:
int gcd(int x, int y) {
if (x % y == 0) //base case
return y;
else
return gcd(y, x % y);
}
what is the output of this code?
- modify it to find the gcd using subtraction instead of %.
Task 03) Run the following code:
int fib(int n)
{
if (n <= 0) // base case
return 0;
else if (n == 1) // base case
return 1;
else
return fib(n 1) + fib(n 2);
}
what is the time complexity of this algorithm and why?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
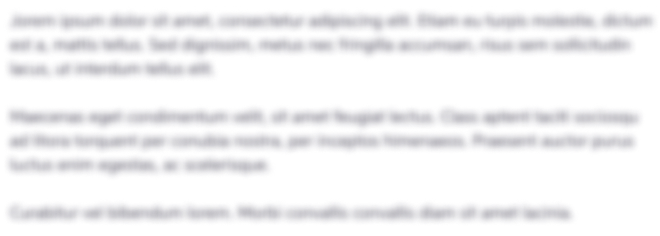
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started