Question
task 1: module alu ( input wire [11:0] A, // 12-bit input A input wire [11:0] B, // 12-bit input B input wire [3:0] opcode,
task 1:
module alu (
input wire [11:0] A, // 12-bit input A
input wire [11:0] B, // 12-bit input B
input wire [3:0] opcode, // 4-bit opcode
output wire [11:0] result, // 12-bit result
output wire zero // zero flag
);
// Internal variables
wire [11:0] temp;
// Implement the logic for each opcode
always @* begin
case (opcode)
// Rotate left
4'b0000: temp = {A[10:0], A[11]};
// Multiplication
4'b0001: temp = A * B;
// Greater comparison
4'b0010: temp = A > B;
// Logical XOR
4'b0011: temp = A ^ B;
// Logical XNOR
4'b0100: temp = ~(A ^ B);
// Subtraction
4'b0101: temp = A - B;
// Rotate right
4'b0110: temp = {A[1:0], A[11:2]};
// Logical NAND
4'b0111: temp = ~(A & B);
// Division
4'b1000: temp = A / B;
// Logical OR
4'b1001: temp = A | B;
// Equal comparison
4'b1010: temp = (A == B);
// Addition
4'b1011: temp = A + B;
// Logical NOR
4'b1100: temp = ~(A | B);
// Logical shift left
4'b1101: temp = A
// Logical shift right
4'b1110: temp = A >> 1;
// Default case (do nothing)
default: temp = A;
endcase
end
// Assign the result and zero flag outputs
assign result = temp;
assign zero = (temp == 0);
endmodule
l need task 2 and test bench
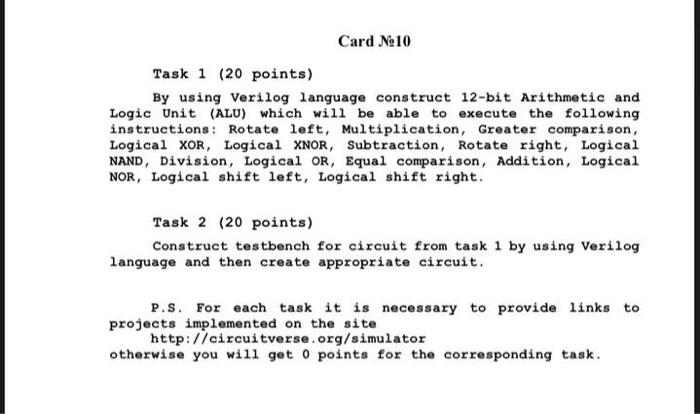
Step by Step Solution
There are 3 Steps involved in it
Step: 1
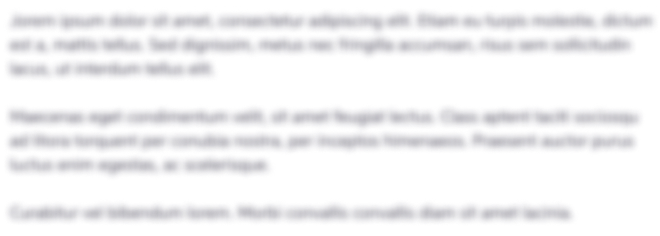
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started