Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Task 1 Task 1 is to copy and paste the code below into the project. This code asks the user for a height and then
Task
Task is to copy and paste the code below into the project. This code asks the user for a height and then prints out a sideways pyramid. For example, the goal is to produce this output with a height of although the actual code will be slightly wrongwe'll fix that in Task Task
The Task tests, however, do expect whitespace to match exactly. To do that, look at the cout lines in both while loops. They both use setw, which sets the expected width so if setw is called, and then is output, it will output spaces so that the total width is : four spaces plus the exclamation point We want to remove the from both setw calls so that it prints the extra space that is expected. Make sure you remove it from both setw calls.
Now, submit the code. It should now pass Task and Task tests.
Task Extra Credit
If you'd like to earn extra credit, use what you've learned from the code above especially the while loops and output to create a square of the given height. You should do this at the end of the main method keeping the existing code so that your program continues to pass the Task and test cases.You can assume there will always be distinct sides to the square so not a height of or
There are a few ways to make several # in a row, but one easy one is to use setfill, which changes what gets added to add width. For example:
cout setfill setw#;
would output: #
Instead of spaces to make the output characters long, there are dashes three and then the # for four total characters
Note that we use single quotes around the dash, to indicate a single character rather than a string. Also, it's important to know that setfill persists, meaning the next time I do an output:
cout setw#;
it will still use the dashes as the fill. If I want to use spaces, I need to switch it back:
cout setfill setw#;
setw on the other hand does not persist. If I next do:
cout #;
That will just produce one # with no additional text before it Copypaste or type your code here
Lab template code, CS
Write and run the tests for the individual problems one at a time.
#include
#include for setw and setfill
using namespace std;
main is where our program begins
int main
Create a height variable and get the input from the user
int height;
cout "Enter the height: ;
cin height;
Use a while loop to count up from to the height
int level ;
while level height
Note that just outputs one backslash: is a special character in C
so we just repeat it to clarify we really mean a backslash
endl starts for "end line" and will output a new line for us
As explained in Task setw specifies how many characters
should be output when we display the backslash
cout setwlevel endl;
Add one to level
level;
Now start at height, and count down to
level height;
while level
cout setwlevel endl;
Subtract one from level
level;
#include
#include for setw and setfill
using namespace std;
int main
int height;
cout "Enter the height: ;
cin height;
int level ;
while level height
cout setwlevel endl;
level;
level height;
while level
cout setwlevel endl;
level;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
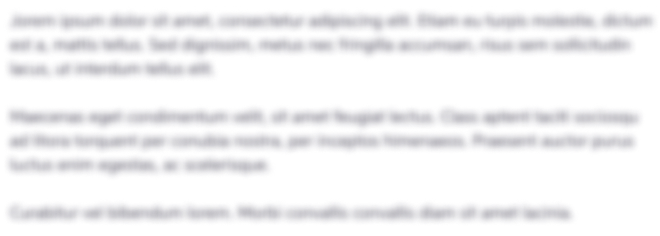
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started