Question
Task 1 We want to define our Superclass Shape, which requires two instance variables x and y. We want to define setter and getter methods
Task 1
We want to define our Superclass Shape, which requires two instance variables x and y.
We want to define setter and getter methods for both instance variables.
Task 2
We now want to create the subclass TwoDimensionalShape, which inherits the Shape class.
Our class definition includes the definition, along with extends Shape (to inherit Shape) class TwoDimensionalShape extends Shape
This class requires two additional instance variables: dimension1 and dimension2.
The x and y variables are used from super class where dimension1 and dimension2 are defined in this class.
Do not declare any other variables besides dimension1 and dimension2.
We need to define a Constructor which accepts initializing parameters for the two instance variables for Shape and the two instance variables for TwoDimensionalShape.
We then need to define the setter and getter methods for the new instance variables.
The setter and getter methods of the Shape class are inherited, thus included in TwoDimensionalShape.
We now want to define the two additional subclasses; Circle and
Rectangle. Both of these subclasses extend the TwoDimensionalShape class.
Task 3
1. Define the class Circle, which inherits TwoDimensionalShape.
Circle inherits all instance variables and methods from
Shape and all instance variables and methods from TwoDimensionalShape.
2. No new Instance variables should be defined for this class, you must use the instance variables from the inherited classes.
3. Define a Constructor, which accepts an x and y coordinate, along with the radius of the circle. This constructor should call the superclass constructor where the radius should be assigned to dimension1of the superclass. Dimension2 is not needed so you can initialize it with 0.
4. Define a method called area, which has no parameters and returns a double. This method returns the area of the circle (PI * radius * radius).
Define setter and getter methods for setting the radius; setRadius and getRadius.
Task 4
1. Define the class Rectangle, which inherits TwoDimensionalShape. Rectangleinherits all instance variables and methods from Shape and allinstance variables and methods from TwoDimensionalShape.
2. No new instance variables should be defined for this class, you must use the instance variables from the inherited classes.
3. Define a Constructor, which accepts an x and y coordinate, along with the length and width of the rectangle. This constructor should call the superclass constructor where the length of the rectangle should be assigned to dimension1 and the width of the rectangle should be assigned to dimension2 of the superclass.
4. Define a method called area, which has no parameters and returns a double. This method returns the area of the rectangle (length x width)
5. Define setter and getter methods for setting both the length and width of the rectangle; getLength, getWidth, setLength and setWidth.
Use the following test harness to test your
subclasses
public class ShapeTest
{
public static void main(
String[] args
)
{
Circle c = new Circle( 1, 1, 5 );
Rectangle r = new Rectangle( 1, 1, 4, 3 );
System.out.println( "Area of the Circle is " + c.area());
System.out.println( "Area of the Rectangle is " + r.area());
}
}
Which will result in the following output:
Area of the Circle is 78.53981633974483
Area of the Rectangle is 12
Step by Step Solution
There are 3 Steps involved in it
Step: 1
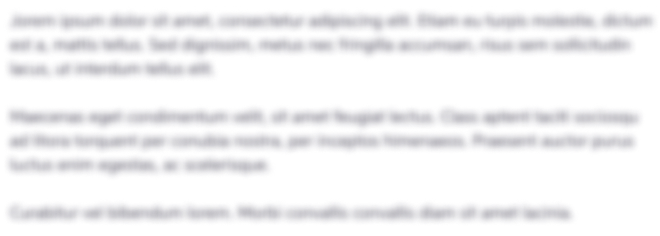
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started