Question
Task 3: toString() method Define a public toString() method on class Ground that returns a String in the following format: Jack[](0) [Quartz#4](1) [Pearl#3](2) [Opal#2](3) /6(4)
Task 3: toString() method Define a public toString() method on class Ground that returns a String in the following format: Jack[](0) [Quartz#4](1) [Pearl#3](2) [Opal#2](3) /6\(4) This string describes the player Jack with an empty jar at position 0, three jars at positions 1, 2 and 3 containing rocks named Quartz, Pearl and Opal with weights of 4, 3 and 2 respectively, and the chest with combination 6 at position 4. The numbers and names may of course differ depending on what data is entered by the user when the constructors are executed. Thus, your string should always incorporate data directly from the fields. If the chest is unlocked, it should be rendered as \6/(4) instead of /6\(4). This task must be implemented compositionally with a toString() method in each class. The String returned by this method can embed variables this way: return "string1 + string2 + variable + another string + You can also use the StringBuffer class described in the text book. You must not, however, use the sprintf method (since it uses the untaught varargs feature).
Task 4: turn() method Define a method in class Ground called turn() which is public, takes no parameters and returns nothing. This method allows the player to have his/her turn by asking the user to type in some command, either 'l' to move the player left, 'r' to move the player right, 'p' to pick up a rock at the current position or 'd' to drop a rock at the current position.
Your method should follow exactly the following output format: Move (l/r/p/d): r
Your program must print the prompt Move (l/r/p/d): and then read the user's input. 6. If the user types l or r, your program should send a message to the player object to move one position to the left or right respectively. 7. If the user types p, your program should send a message to the player to pick up a rock from a jar on the ground at the current position. This command does nothing if the players jar already contains a rock. Note that you should apply good design principles to simplify your code, since you will also receive marks for the quality of your design. 8. If the user types d, your program should send a message to the player to drop a rock into a jar on the ground at the current position. This command does nothing if there is already a rock in a jar at that position. (You should define any additional methods as necessary)
Task 5: Placing rocks on the chest Modify the behaviour of the p (pickup) and d (drop) commands so that if the players current position is the same as that of the chest, a rock will be picked up from, or dropped onto the chest. Because the chest contains 3 jars at identical positions, picking up and dropping rocks over the chest follows priority rules: When dropping a rock, jar1 is tried first, and if it already contains a rock, then jar2 is tried, and then jar3. When picking up a rock, the player looks in jar3 first, and if no rock is in there, then jar2 is tried, and then jar1
Task 6: Loops You will now implement some loops in your program. After completing each loop, submit it to PLATE to receive marks and feedback for that loop. Loop 1: Define a method in class Ground called play() which is public, takes no parameters, and returns nothing. This method repeatedly invokes the turn() method until the treasure is unlocked and the game is over. When the loop stops, the program should print You unlocked the treasure!. You should also print out the toString() representation of the ground before each turn and after the last turn. You can test this method in BlueJ by right-clicking on the Ground class to create a new Ground, and then right-clicking on the created object and selecting the play() method. Loop 2: When the user is asked to specify the treasure chest combination, this number must be within the range 5-10. Write a loop which repeatedly asks the user to enter a number until the user enters a valid number within the range 5-10. Your output should follow the following format exactly: Enter chest combination (5-10): 4 Invalid combination. Enter chest combination (5-10): 2 Invalid combination. Enter chest combination (5-10): 12 Invalid combination. Enter chest combination (5-10): 5 As you attempt each loop, submit your project to PLATE to see the expected input/output, and adjust your code to comply with PLATEs output. After you have done this, define a class called Main, which has a correctly defined main() method according to the lecture notes. This method should create a new Ground object, and then invoke its play() method.
my Code:
import java.util.Scanner; public class Global { public static final Scanner keyboard = new Scanner(System.in); }
public class Ground { private Player player; private Jar jar1, jar2, jar3; private Chest chest; public Ground() { player = new Player(); jar1 = new Jar(1, new Stone()); jar2 = new Jar(2, new Stone()); jar3 = new Jar(3, new Stone()); chest = new Chest(); }
public boolean isChestUnlocked() { return chest.ChestUnlocked(); }
public void turn() { System.out.print("Move (l/r/p/d): "); String input = Global.keyboard.nextLine(); if(input == "l") { player.move(-1); } if(input == "r") { player.move(1); } if(input == "p") { if(player.getJar() == null) { System.out.print("Player's Jar already contains a rock"); } else { // Jar.moveTo(player.getJar()); } if(input == "d") { //if(Player(jar) == null) { //player.getJar() = ; } //else System.out.print("There is already a rock in a jar at that positon"); } } } public String toString() { if(isChestUnlocked() == true) { return this.player.toString() + " " + jar1.toString() + " " + jar2.toString() + " " + jar3.toString() + " \\" + chest.getCombination() + "/(4)"; } else { return player.toString() + " " + jar1.toString() + " " + jar2.toString() + " " + jar3.toString() + " /" + chest.getCombination() + "\\(4)"; } } }
public class Player { private String name; private int position; private Jar jar; public Player() { position = 0; jar = new Jar(); System.out.print("Enter player's name: "); name = Global.keyboard.nextLine(); }
public void move(int distance) { position = position+distance; jar.move(distance); }
public void pickUp(Jar jar) { jar.moveTo(this.jar); }
public String getName() { return name; }
public Jar getJar() { return this.jar; } public String toString() { return getName() + "[](" + position + ")"; } }
public class Chest { private int combination; private int position; private Jar jar1, jar2, jar3; public Chest() { System.out.print("Enter chest combination (5-10): "); combination = Global.keyboard.nextInt(); Global.keyboard.nextLine(); position = 4; jar1 = new Jar(4, null); jar2 = new Jar(4, null); jar3 = new Jar(4, null); }
public int getCombination() { return combination; } public boolean ChestUnlocked() { if(jar1.getStoneWeight()+jar2.getStoneWeight()*jar3.getStoneWeight()==getCombination()) { return true; } return false; } }
public class Jar { private int position; private Stone stone; private Player player; public Jar() { position = 0; stone = null; }
public Jar(int position, Stone stone) { this.position = position; this.stone = stone; }
public void move(int distance) { position = position+distance; }
public void moveTo(Jar jar) { jar.stone = stone; stone = null; }
public int getStoneWeight() { if(stone == null) { return 0; } else return stone.getWeight(); }
public String getStonesWeight() { if(stone == null) { return ""; } else return "#" + String.valueOf(stone.getWeight()); } public String getStoneName() { if(stone == null) { return ""; } else return stone.getName(); }
public String getPlayerName() { return player.getName(); }
public Jar getPlayerJar() { return player.getJar(); } public int getStonePosition() { return position; } public String toString() { return "[" + getStoneName() + getStonesWeight() +"](" + getStonePosition() + ")"; } }
public class Stone { private String name; private int weight; public Stone() { System.out.print("Enter stone name: "); name = Global.keyboard.nextLine(); System.out.print("Enter stone weight: "); weight = Global.keyboard.nextInt(); Global.keyboard.nextLine(); }
public int getWeight() { return weight; }
public String getName() { return name; }
public String getWeightNumber() { return "#" + getWeight(); } public String toString() { return getName() + getWeightNumber(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
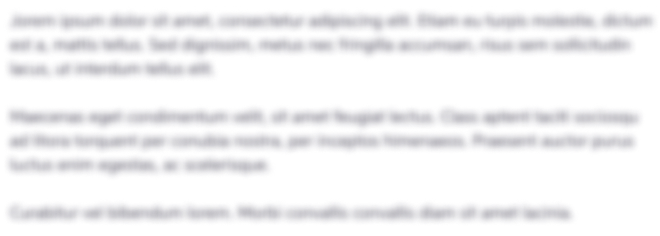
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started