Question
Task D.1 : Assign to L the list consisting of the first five letters ['A','B','C','D','E']. Next, use L in an expression whose value is [(0,
Task D.1: Assign to L the list consisting of the first five letters ['A','B','C','D','E']. Next, use L in an expression whose value is [(0, A), (1, B), (2, C), (3, D), (4, E)].
Your expression should use a range and a zip, but should not use a comprehension.
Task D.2. Starting from the lists [10, 25, 40] and [1, 15, 20], write a comprehension whose value is the three-element list in which the first element is the sum of 10 and 1, the second is the sum of 25 and 15, and the third is the sum of 40 and 20. Your expression should use zip but not list.
Task D.3. Suppose dlist is a list of dictionaries and k is a key that appears in all the dictionaries in dlist. Write a comprehension that evaluates to the list whose i-th element is the value corresponding to key k in the ith dictionary in dlist. Test your comprehension with some data. Here are some example data.
dlist = [{'James':'Sean', 'director':'Terence'}, {'James':'Roger', 'director':'Lewis'}, {'James':'Pierce', 'director':'Roger'}] k = 'James'
Task D.4. Modify the comprehension in D.3 to handle the case in which k might not appear in all the dictionaries. The comprehension evaluates to the list whose i-th element is the value corresponding to key k in the ith dictionary in dlist if that dictionary contains that key, and 'NOT PRESENT' otherwise. Test your comprehension with k = 'Bilbo' and k = 'Frodo' and with the following list of dictionaries:
dlist = [{'Bilbo':'Ian','Frodo':'Elijah'}, {'Bilbo':'Martin','Thorin':'Richard'}]
Task D.5. Using range, write a comprehension whose value is a dictionary. The keys should be the integers from 0 to 99 and the value corresponding to a key should be the square of the key.
Task D.6. Assign some set to the variable D, e.g. D ={'red','white','blue'}. Now write a comprehension that evaluates to a dictionary that represents the identity function on D.
Task D.6. Using the variables base=10 and digits=set(range(base)), write a dictionary comprehension that maps each integer between zero and nine hundred ninety nine to the list of three digits that represents that integer in base 10. That is, the value should be
{0: [0, 0, 0], 1: [0, 0, 1], 2: [0, 0, 2], 3: [0, 0, 3], ..., 10: [0, 1, 0], 11: [0, 1, 1], 12: [0, 1, 2], ..., 999: [9, 9, 9]} 1
Your expression should work for any base. For example, if you instead assign 2 to base and assign {0,1} to digits, the value should be
{0: [0, 0, 0], 1: [0, 0, 1], 2: [0, 1, 0], 3: [0, 1, 1], ..., 7: [1, 1, 1]}
Task D.7. Suppose d is a dictionary that maps some employee IDs (a subset of the integers from 0 to n1) to salaries. Suppose L is an n-element list whose i-th element is the name of employee number i. Your goal is to write a comprehension whose value is a dictionary mapping employee names to salaries. You can assume that employee names are distinct. However, not every employee ID is represented in d. Test your comprehension with the following data:
id2salary = {0:1000.0, 3:990, 1:1200.50} names = ['Larry', 'Curly', '', 'Moe']
Task D.8. For a given set of integers, write a code to find all coprime couples of the numbers in the set. Check it for the following set:
{5,17,43,51}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
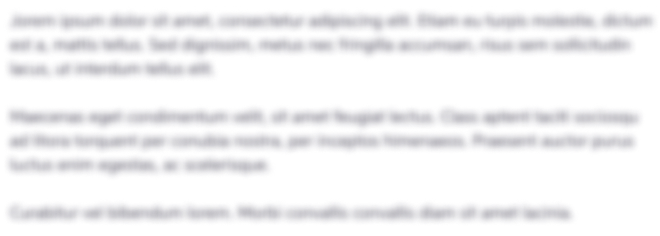
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started