Question
Task Description Please solve the following task. Design the agent program of a model-based reflex vacuum agent with the following properties: For a vacuum agent
Task Description
Please solve the following task. Design the agent program of a model-based reflex vacuum agent with the following properties:
For a vacuum agent that starts in tile (0,0), it cleans the environment entirely from dirt.
We assume that our task environment is partially observable: the percepts are of the same type as in the two-tile vacuum world problem discussed in class in the sense that for each location, the agent is able to record its current coordinates as well as the cleanliness status ('Dirty' or 'Clean').
The internal state of the vacuum agent keeps track of the tiles already visited and their cleanliness. Design the program such that an already visited tile is not visited again.
For each time step, this agent program chooses between one of the following actions:
'Suck': The vacuum agent cleans the tile. This changes its cleanliness status from 'Dirty' to 'Clean'. If the tile was already clean, nothing happens.
'NoOp': The vacuum agent does not do anything. Furthermore, the agent program terminates after this action is taken.
'Left': The vacuum agent moves from its current location to one tile on its left.
'Right': The vacuum agent moves from its current location to one tile on its right.
'Up': The vacuum agent moves from its current location to one tile above it.
'Down': The vacuum agent moves from its current location to one tile below it.
If a location change is to result in "bumping" into the boundary of the vacuum world, the agent simply does not move.
The agent shall not only be able to solve vacuum world environment vacuum_env based on random.seed(42), but also environments with different Dirt placements as long as the agent starts in (0,0). For example, it should be able to solve vacuum_env_alternative below.
The agent also keeps track of the performance measure performance as follows:
For each action among 'Left', 'Right', 'Up' and 'Down', this value is reduced by -1 (even if the agent does not move).
If a tile is successfully cleaned by the action 'Suck', the value is increased by +10.
If the action 'Suck' is used for a clean tile, the value is reduced by -1.
If the action 'NoOp', the performance measure does not change.
Code provide below
----------------------------------------------------------------------------------------------------------------------------------------------------
def ModelBasedReflexVacuumAgent(environment: VacuumEnvironment): # rules, update_state, transition_model, sensor_model """ This function returns an Agent equipped with a model-based reflex agent program for the vacuum world enviroment. We have already implemented its 'transition_model' and its 'sensor_model' below. It takes as an input argument an instance of the VacuumEnvironment class, and returns an Agent class object equipped with the agent program defined in its method 'program'. Parameters ---------- environment : VacuumEnvironment object Represents the vacuum world environment for which the agent is designed. (This is only used for the agent to know the geometry of the tiles in the enviroment.) Returns ------- Agent : Agent object Agent object provided with agent program (see class definition above) that is the one of a model-based reflex agent. """ class State: """ Class describing the current model/state of the model-based reflex agent. Initialized with empty location information and empty dictionary tracking cleanliness information. """ def __init__(self): self.location = None self.cleanliness = dict() def transition_model(state,action): """ Implements transition model of model-based reflex agent. Strictly speaking, this is not necessarily needed to implement its agent program. Parameters ---------- state : State object Represents the current (internal) state of the agent. action : str Action taken; Returns ------- state : State object New state after applying 'action' to state provided to the function. """ if action == 'Suck': state.cleanliness[state.location] = 'Clean' elif action == 'NoOp': return state else: if action == 'Left': targetloc = (state.location[0]-1,state.location[1]) elif action == 'Right': targetloc = (state.location[0]+1,state.location[1]) elif action == 'Up': targetloc = (state.location[0],state.location[1]+1) elif action == 'Down': targetloc = (state.location[0],state.location[1]-1) if environment.is_inbounds(targetloc): state.location = targetloc return state def sensor_model(state,percept): """ Implements the sensor model of the agent, i.e., it describes how the current state is updated given a new percept. Parameters ---------- state : State object Represents the current (internal) state of the agent. percept : tuple of size 2. First element contains the location (likewise a tuple of size 2, e.g., (0,0)), second element contains str encoding the cleanliness property of a tile ('Dirty' or 'Clean').
Returns ------- state : State object Represents the updated (internal) state of the agent after incorporating the percept information.
""" location, cleanliness = percept state.cleanliness[location] = cleanliness state.location = location return state def update_state(state,action,percept): """ Updates the state based on last action and current percept. (In our case, it is sufficient to use only percept and state information.)
Parameters ---------- state : State object Represents the updated (internal) state of the agent after incorporating the percept information. action : str Represents action taken most recently. percept : tuple of size 2. First element contains the location (likewise a tuple of size 2, e.g., (0,0)), second element contains str encoding the cleanliness property of a tile ('Dirty' or 'Clean').
Returns ------- state : State object Updated state. """ state = sensor_model(state,percept) return state
def program(percept): """ This function implements the agent program of the model-based reflex agent for the vacuum agent problem.
Parameters ---------- percept : tuple of size 2. First element contains the location (likewise a tuple of size 2, e.g., (0,0)), second element contains str encoding the cleanliness property of a tile ('Dirty' or 'Clean').
Returns ------- next_action : str Action taken by the agent given provided percept. """ ### Add your code below / adapt existing code ###
next_action = 'NoOp' # this line might need to be changed as well. program.action = next_action return next_action
program.state = State() program.action = None return Agent(program)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
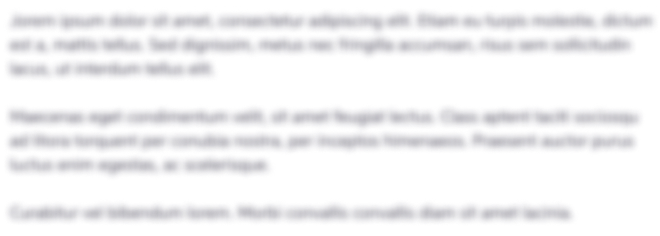
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started