Question
Tasks: Follow the directions below to complete your lab assignment For today's lab we will be completing Exercise P9.5 from the book. Book =Big Java
Tasks: Follow the directions below to complete your lab assignment For today's lab we will be completing Exercise P9.5 from the book. Book =Big Java Early Objects
IMPORTANT!! All of your method/class names need to match what is shown in this document. This is the public interface of your class. The classes and methods you need to implement are as follows: Circuit single method getResistance. Note that this is simply an empty circuit with no resistors, and therefore the resistance is always 0 for objects of this class. This is the parent class. Resistor subclass of Circuit. Represents a single resistor. Needs to override the getResistance method from the superclass Circuit. To compute the value of the Resistor object you simply return the resistance value. Serial subclass of Circuit. Contains an ArrayList
Read the problem description in the book for more details! (additionally, you will want to reference the material from chapter 9).
I have uploaded a file called CircuitDemo.java to Google Drive. This demonstrates how your code should function. (Note that you will need to create appropriate constructors for each class shown above). This sample code builds the circuit shown in the book with the top resistor = 100 Ohms, the bottom left resistor = 100 Ohms, and the bottom right resistor = 200 Ohms. You should make sure you can calculate this circuit by hand. If you need help with this just let me know. (Often programmers have to write programs using formulas outside of their area of expertise. Make sure you can do calculations by hand or your program will never work!).
You can choose which way to test your circuit from the two choices below. You can either use standard test procedures as we have used previously (in the CircuitDemo.java file), or you can create a new class CircuitTester to run tests using the JUnit unit test framework. Using JUnit is bonus material. What I am saying is you must test your program in either case, but if you go to the extra work to use JUnit you will receive bonus points. I would suggest at the least you create 2-3 tests in the CircuitDemo.java file before attempting the JUnit tests.
Choice 1: You should add multiple circuits to the CircuitDemo.java file to verify that your program works as expected. Take screen-shots or capture the output text and include this in your project folder. Sample output from a working project using the given CircuitDemo.java file is shown below. (Look at the java file on UTC Learn to see the test code that produced this output).
Combined resistance: 75.0 Expected: 75.0
Choice 2: BONUS: Use the JUnit unit testing framework to run multiple tests on your circuit. Use class name CircuitTester. The completeness of your testing will determine the number of bonus points you receive. (Also, your tests should pass). Feel free to use google to figure out how to use junit and get it running. (You may also discuss this among yourselves JUST HOW TO GET IT WORKING). Once junit is working, you must think of and implement YOUR OWN tests. Of course, I am available to help you with this as well. (The basic idea is that you will need to import two .jar files into your current project, and then write tests as demonstrated in section 8.6 of the book).
Some helpful tips:
Compile often do it.
You are responsible for a larger amount of the design process in this lab. You need to draw on paper how you want your subclasses to work and plan before you actually write code.
It may be helpful to use the Debugger or print statements to check your work. (always).
We will have a demo/tester class for this lab called CircuitDemo. You will need to fill in this class with enough tests to verify that your project works as expected. (Alternately, you may use the JUnit unit test framework from Section 8.6 in the book more details below).
Resistor values should be stored as doubles.
There are two types of circuits we are creating, series and parallel (as well as a single resistor). The formula for computing the entire resistance of each type of circuit is given below. I googled these images from http://hyperphysics.phy-astr.gsu.edu/hbase/electric/resis.html
SAMPLE CODES
1.
public class CircuitTester01 {
public static void main(String[] args) { Serial c1 = new Serial(); c1.add(new Resistor(100)); c1.add(new Resistor(200)); c1.add(new Resistor(150)); System.out.println("c1 total R = " + c1.getResistance()); Parallel c2 = new Parallel(); c2.add(new Resistor(100)); c2.add(new Resistor(100)); c2.add(new Resistor(100)); System.out.println("c2 total R = " + c2.getResistance()); Serial c3 = new Serial(); c3.add(c1); c3.add(c2); System.out.println("c3 total R = " + c3.getResistance()); Parallel c4 = new Parallel(); c4.add(c1); c4.add(c2); System.out.println("c4 total R = " + c4.getResistance()); } }
2.
/** * A class to run tests on the Circuit class and subclasses * @author Horstman * @version 02/06/2014 * */ public class CircuitDemo { /** method that implements tests for Circuit class and sublclasses @param args - Not Used. */ public static void main(String[] args) { Parallel circuit1 = new Parallel(); circuit1.add(new Resistor(100)); Serial circuit2 = new Serial(); circuit2.add(new Resistor(100)); circuit2.add(new Resistor(200)); circuit1.add(circuit2); System.out.println("Combined resistance: " + circuit1.getResistance()); System.out.println("Expected: 75.0"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
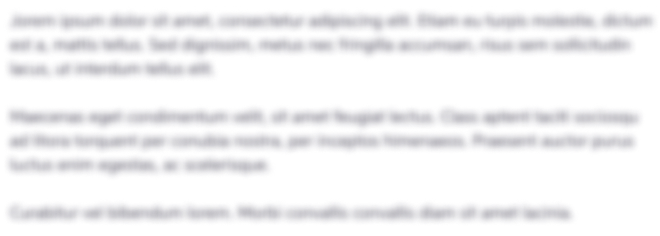
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started