Question
Ten Quick Questions. NO EXPLAINATION NECESSARY just the answer only. Will rate if fully complete. All the source files are located below. 11. T or
Ten Quick Questions. NO EXPLAINATION NECESSARY just the answer only. Will rate if fully complete. All the source files are located below.
11. T or F? The class OBJECT is defined as an abstract class.
12. T or F? The constructor for the POINT2D class (shown below) is a default constructor.
POINT2D(double x,double y): x(x),y(y) {}
13. T or F? The Type() function for the POINT2D class (shown below) is a virtual function.
const char *Type() { return( "POINT2D" ); }
14. T or F? The Print() function for the POINT2D class (shown below) is a virtual function because it is defined with the reserved word virtual.
virtual void Print();
The statements shown below are taken from the SHAPE class definition.
public:
enum COLOR { Q=0,R=1,B=2,G=3 };
15. T or F? The SHAPE constructor shown below can legally reference the COLOR enumeration constant Q using the syntax SHAPE::Q in place of the simple syntax used.
SHAPE(): color(Q) {}
16. T or F? The SHAPE constructor shown below can legally reference the enumeration type name COLOR using the syntax SHAPE::COLOR.
SHAPE(COLOR color): color(color) {}
17. T or F? When the access modifier for COLOR is changed from public to protected the answers to Questions 15 and 16 must change.
18. T or F? When the access modifier for COLOR is changed from public to private the answers to Questions 15 and 16 must change.
19. T or F? It is illegal to add the statement shown below to the Problem.cpp function main().
COLOR color = R;
20. T or F? It is illegal to add the statement shown below the Problem.cpp function main().
COLOR color = COLOR::R;
//--------------------------------------------------------
// Dr. Art Hanna
// Chapter #12 Problem
// Problem.cpp
//--------------------------------------------------------
#include
#include
using namespace std;
#include "..\..\Random.h"
#define POINT2DTYPE 1
#define LINETYPE 2
#define CIRCLETYPE 3
#define SQUARETYPE 4
#define RECTANGLETYPE 5
#define CUBETYPE 6
#define BOXTYPE 7
//--------------------------------------------------------
class OBJECT
//--------------------------------------------------------
{
private:
static int sequence;
int ID;
public:
OBJECT() { ID = sequence++; }
virtual ~OBJECT() {}
int GetID() { return( ID ); }
virtual void Print() = 0;
};
int OBJECT::sequence = 1;
//--------------------------------------------------------
class POINT2D: public OBJECT
//--------------------------------------------------------
{
protected:
double x;
double y;
public:
POINT2D(double x,double y): x(x),y(y) {}
const char *Type() { return( "POINT2D" ); }
POINT2D &operator=(POINT2D &RHS)
{
x=RHS.x, y=RHS.y, cout << "= ";
return(*this);
}
POINT2D(const POINT2D &RHS): x(RHS.x),y(RHS.y) {}
virtual void Print()
{
cout << setw(10) << Type() << ": ";
cout << "ID = " << setw(3) << GetID();
cout << ", (x,y) = (" << setw(5) << setprecision(1) << fixed << x;
cout << "," << setw(5) << setprecision(1) << fixed << y << ")";
}
};
//--------------------------------------------------------
class SHAPE: public OBJECT
//--------------------------------------------------------
{
public:
enum COLOR { Q=0,R=1,B=2,G=3 };
protected:
COLOR color;
public:
SHAPE(): color(Q) {}
SHAPE(COLOR color): color(color) {}
virtual const char *Type() = 0;
virtual void Print()
{
cout << setw(10) << Type() << ": ";
cout << "ID = " << setw(3) << GetID();
cout << ", color = " << ((color == R) ? 'R' : ((color == B) ? 'B' : ((color == G) ? 'G' : 'Q')));
}
};
//--------------------------------------------------------
class LINE: public SHAPE
//--------------------------------------------------------
{
protected:
POINT2D p1;
POINT2D p2;
public:
LINE(COLOR color,POINT2D p1,POINT2D p2): SHAPE(color),p1(p1),p2(p2) {}
virtual const char *Type() { return( "LINE" ); }
virtual void Print()
{
SHAPE::Print(), cout << ", p1 = ", p1.POINT2D::Print(),
cout << ", p2 = ", p2.POINT2D::Print();
}
};
//--------------------------------------------------------
class TWODSHAPE: public SHAPE
//--------------------------------------------------------
{
protected:
const int dimension;
public:
TWODSHAPE(COLOR color): SHAPE(color),dimension(2) {}
virtual void Print()
{
SHAPE::Print(), cout << ", dimension = " << dimension;
}
};
//--------------------------------------------------------
class CIRCLE: public TWODSHAPE
//--------------------------------------------------------
{
protected:
int radius;
public:
CIRCLE(COLOR color,int radius): TWODSHAPE(color),radius(radius) {}
virtual const char *Type() { return( " CIRCLE"); }
virtual void Print()
{
TWODSHAPE::Print(), cout << ", radius = " << radius;
}
};
//--------------------------------------------------------
class SQUARE: public TWODSHAPE
//--------------------------------------------------------
{
protected:
int side;
public:
SQUARE(COLOR color,int side): TWODSHAPE(color),side(side) {}
virtual const char *Type() { return( "SQUARE" ); }
virtual void Print()
{
TWODSHAPE::Print(), cout << ", side = " << side;
}
};
//--------------------------------------------------------
class RECTANGLE: public TWODSHAPE
//--------------------------------------------------------
{
protected:
int height;
int width;
public:
RECTANGLE(COLOR color,int height,int width): TWODSHAPE(color),height(height),width(width) {}
virtual const char *Type() { return( "RECTANGLE" ); }
virtual void Print()
{
TWODSHAPE::Print(), cout << ", height = " << height << ", width = " << width;
}
};
//--------------------------------------------------------
class THREEDSHAPE: public SHAPE
//--------------------------------------------------------
{
protected:
const int dimension;
public:
THREEDSHAPE(COLOR color): SHAPE(color),dimension(3) {}
virtual void Print()
{
SHAPE::Print(), cout << ", dimension = " << dimension;
}
};
//--------------------------------------------------------
class CUBE: public THREEDSHAPE
//--------------------------------------------------------
{
protected:
int side;
public:
CUBE(COLOR color,int side): THREEDSHAPE(color),side(side) {}
virtual const char *Type() { return( "CUBE" ); }
virtual void Print()
{
THREEDSHAPE::Print(), cout << ", side = " << side;
}
};
//--------------------------------------------------------
class BOX: public THREEDSHAPE
//--------------------------------------------------------
{
protected:
int height;
int width;
int depth;
public:
BOX(COLOR color,int height,int width,int depth):
THREEDSHAPE(color),height(height),width(width),depth(depth) {}
virtual const char *Type() { return( "BOX" ); }
virtual void Print()
{
THREEDSHAPE::Print(), cout << ", height = " << height
<< ", width = " << width
<< ", depth = " << depth;
}
};
//--------------------------------------------------------
int main()
//--------------------------------------------------------
{
OBJECT **objects;
int n;
// COLOR color = R; // Added for Question 19
// COLOR color = COLOR::R; // Added for Question 20
// SHAPE::COLOR color = SHAPE::R; // Added for Question 21
// SHAPE::COLOR color = SHAPE::COLOR::R; // Added for Question 22
SetRandomSeed();
cout << "n? "; cin >> n;
objects = new OBJECT * [ n+1 ];
for (int i = 1; i <= n; i++)
{
switch ( RandomInteger(POINT2DTYPE,BOXTYPE) )
{
case POINT2DTYPE:
objects[i] = new POINT2D(RandomReal()*100.0,
RandomReal()*100.0);
break;
case LINETYPE:
objects[i] = new LINE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
POINT2D(RandomReal()*100.0,
RandomReal()*100.0),
POINT2D(RandomReal()*100.0,
RandomReal()*100.0));
break;
case CIRCLETYPE:
objects[i] = new CIRCLE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10));
break;
case SQUARETYPE:
objects[i] = new SQUARE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10));
break;
case RECTANGLETYPE:
objects[i] = new RECTANGLE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10),
RandomInteger(1,10));
break;
case CUBETYPE:
objects[i] = new CUBE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10));
break;
case BOXTYPE:
objects[i] = new BOX((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10),
RandomInteger(1,10),
RandomInteger(1,10));
break;
}
}
for (int i = 1; i <= n; i++)
{
objects[i]->Print();
cout << endl;
}
for (int i = 1; i <= n; i++)
delete objects[i];
delete [] objects;
system("PAUSE");
return( 0 );
}
//--------------------------------------------------------
// Dr. Art Hanna
// Chapter #12 Problem-2
// Problem-2.cpp
//--------------------------------------------------------
#include
#include
#include
using namespace std;
#include "..\..\Random.h"
#define POINT2DTYPE 1
#define LINETYPE 2
#define CIRCLETYPE 3
#define SQUARETYPE 4
#define RECTANGLETYPE 5
#define CUBETYPE 6
#define BOXTYPE 7
#define POINT3DTYPE 8
#define SPHERETYPE 9
...Same code as found in Problem.cpp with added Area() and Volume() functions...
//--------------------------------------------------------
class POINT3D: public OBJECT
//--------------------------------------------------------
{
Student adds missing code
};
//--------------------------------------------------------
class SPHERE: public THREEDSHAPE
//--------------------------------------------------------
{
Student adds missing code
};
//--------------------------------------------------------
int main()
//--------------------------------------------------------
{
int n;
SetRandomSeed();
cout << "n? "; cin >> n;
for (int i = 1; i <= n; i++)
{
OBJECT *object;
switch ( RandomInteger(POINT2DTYPE,SPHERETYPE) )
{
case POINT2DTYPE:
object = new POINT2D(RandomReal()*100.0,
RandomReal()*100.0);
break;
case LINETYPE:
object = new LINE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
POINT2D(RandomReal()*100.0,
RandomReal()*100.0),
POINT2D(RandomReal()*100.0,
RandomReal()*100.0));
break;
case CIRCLETYPE:
object = new CIRCLE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10));
break;
case SQUARETYPE:
object = new SQUARE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10));
break;
case RECTANGLETYPE:
object = new RECTANGLE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10),
RandomInteger(1,10));
break;
case CUBETYPE:
object = new CUBE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10));
break;
case BOXTYPE:
object = new BOX((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10),
RandomInteger(1,10),
RandomInteger(1,10));
break;
case POINT3DTYPE:
object = new POINT3D(RandomReal()*100.0,
RandomReal()*100.0,
RandomReal()*100.0);
break;
case SPHERETYPE:
object = new SPHERE((SHAPE::COLOR) RandomInteger((int) SHAPE::R,SHAPE::G),
RandomInteger(1,10),
POINT3D(RandomReal()*100.0,
RandomReal()*100.0,
RandomReal()*100.0));
break;
}
void *pointer = object;
(static_cast(pointer))->Print();
pointer = dynamic_cast(object);
if ( pointer != NULL )
cout << ", area = "
<< setw(7) << setprecision(1) << fixed
<< (static_cast(pointer))->Area();
pointer = dynamic_cast(object);
if ( pointer != NULL )
cout << ", volume = "
<< setw(7) << setprecision(1) << fixed
<< (static_cast(pointer))->Volume();
cout << endl;
delete object;
}
system("PAUSE");
return( 0 );
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
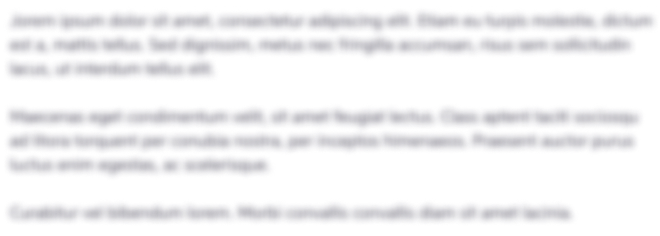
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started