Question
test0.c // g++ text.c -l sqlite3 #include #include int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc; rc = sqlite3_open(test.db,
test0.c
// g++ text.c -l sqlite3
#include
int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc;
rc = sqlite3_open("test.db", &db);
if( rc ){ fprintf(stderr, "Can't open database: %s ", sqlite3_errmsg(db)); }else{ fprintf(stderr, "Opened database successfully "); } sqlite3_close(db); }
test1.c
#include
static int callback(void *NotUsed, int argc, char **argv, char **azColName){ int i; for(i=0; i int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc; char *sql; /* Open database */ rc = sqlite3_open("test.db", &db); if( rc ){ fprintf(stderr, "Can't open database: %s ", sqlite3_errmsg(db)); exit(0); }else{ fprintf(stdout, "Opened database successfully "); } /* Create SQL statement */ sql = "CREATE TABLE COMPANY(" \ "ID INT PRIMARY KEY NOT NULL," \ "NAME TEXT NOT NULL," \ "AGE INT NOT NULL," \ "ADDRESS CHAR(50)," \ "SALARY REAL );"; /* Execute SQL statement */ rc = sqlite3_exec(db, sql, callback, 0, &zErrMsg); if( rc != SQLITE_OK ){ fprintf(stderr, "SQL error: %s ", zErrMsg); sqlite3_free(zErrMsg); }else{ fprintf(stdout, "Table created successfully "); } sqlite3_close(db); return 0; } test2.c #include static int callback(void *NotUsed, int argc, char **argv, char **azColName){ int i; for(i=0; i int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc; char *sql; /* Open database */ rc = sqlite3_open("test.db", &db); if( rc ){ fprintf(stderr, "Can't open database: %s ", sqlite3_errmsg(db)); exit(0); }else{ fprintf(stderr, "Opened database successfully "); } /* Create SQL statement */ sql = "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY) " \ "VALUES (1, 'Paul', 32, 'California', 20000.00 ); " \ "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY) " \ "VALUES (2, 'Allen', 25, 'Texas', 15000.00 ); " \ "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY)" \ "VALUES (3, 'Teddy', 23, 'Norway', 20000.00 );" \ "INSERT INTO COMPANY (ID,NAME,AGE,ADDRESS,SALARY)" \ "VALUES (4, 'Mark', 25, 'Rich-Mond ', 65000.00 );"; /* Execute SQL statement */ rc = sqlite3_exec(db, sql, callback, 0, &zErrMsg); if( rc != SQLITE_OK ){ fprintf(stderr, "SQL error: %s ", zErrMsg); sqlite3_free(zErrMsg); }else{ fprintf(stdout, "Records created successfully "); } sqlite3_close(db); return 0; } test3.c #include static int callback(void *data, int argc, char **argv, char **azColName){ int i; fprintf(stderr, "%s: ", (const char*)data); for(i=0; i int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc; char *sql; const char* data = "Callback function called"; /* Open database */ rc = sqlite3_open("test.db", &db); if( rc ){ fprintf(stderr, "Can't open database: %s ", sqlite3_errmsg(db)); exit(0); }else{ fprintf(stderr, "Opened database successfully "); } /* Create SQL statement */ sql = "SELECT * from COMPANY"; /* Execute SQL statement */ rc = sqlite3_exec(db, sql, callback, (void*)data, &zErrMsg); if( rc != SQLITE_OK ){ fprintf(stderr, "SQL error: %s ", zErrMsg); sqlite3_free(zErrMsg); }else{ fprintf(stdout, "Operation done successfully "); } sqlite3_close(db); return 0; } test4.c #include static int callback(void *data, int argc, char **argv, char **azColName){ int i; fprintf(stderr, "%s: ", (const char*)data); for(i=0; i int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc; char *sql; const char* data = "Callback function called"; /* Open database */ rc = sqlite3_open("test.db", &db); if( rc ){ fprintf(stderr, "Can't open database: %s ", sqlite3_errmsg(db)); exit(0); }else{ fprintf(stderr, "Opened database successfully "); } /* Create merged SQL statement */ sql = "UPDATE COMPANY set SALARY = 25000.00 where ID=1; " \ "SELECT * from COMPANY"; /* Execute SQL statement */ rc = sqlite3_exec(db, sql, callback, (void*)data, &zErrMsg); if( rc != SQLITE_OK ){ fprintf(stderr, "SQL error: %s ", zErrMsg); sqlite3_free(zErrMsg); }else{ fprintf(stdout, "Operation done successfully "); } sqlite3_close(db); return 0; } test5.c #include static int callback(void *data, int argc, char **argv, char **azColName){ int i; fprintf(stderr, "%s: ", (const char*)data); for(i=0; i int main(int argc, char* argv[]) { sqlite3 *db; char *zErrMsg = 0; int rc; char *sql; const char* data = "Callback function called"; /* Open database */ rc = sqlite3_open("test.db", &db); if( rc ){ fprintf(stderr, "Can't open database: %s ", sqlite3_errmsg(db)); exit(0); }else{ fprintf(stderr, "Opened database successfully "); } /* Create merged SQL statement */ sql = "DELETE from COMPANY where ID=2; " \ "SELECT * from COMPANY"; /* Execute SQL statement */ rc = sqlite3_exec(db, sql, callback, (void*)data, &zErrMsg); if( rc != SQLITE_OK ){ fprintf(stderr, "SQL error: %s ", zErrMsg); sqlite3_free(zErrMsg); }else{ fprintf(stdout, "Operation done successfully "); } sqlite3_close(db); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
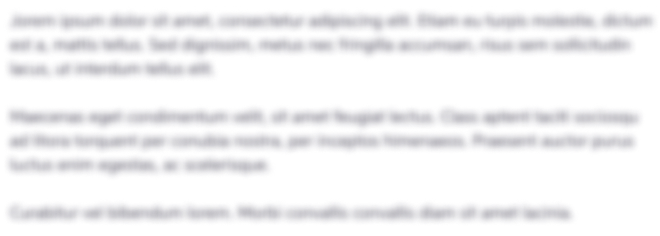
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started