Question
Tester: public class UnorderedArrayListTester { public static void main(String[] args) { // Test the unordered array list UArrayList al = new UArrayList(); System.out.println(List [] :
Tester:
public class UnorderedArrayListTester {
public static void main(String[] args) { // Test the unordered array list
UArrayList al = new UArrayList(); System.out.println("List [] : " + al); System.out.println( " ****Testing empty exception - should throw exceptions ****"); testEmptyCollectionException(al); testObserverMethods(al);
addToRearAndFrontTest(al);
System.out.println( " ****Testing empty exception - should not throw any exceptions ****"); testEmptyCollectionException(al);
// testRemoveMethods(al);
// addToRearAndFrontTest(al); testAddAfter(al); testAddAt(al);
testRemoveMethods(al);
System.out.println( " ****Testing empty exception - should throw exceptions ****"); testEmptyCollectionException(al);
}
private static void testObserverMethods(UArrayList al) { // test contains(), isEmpty(), size()
}
public static void testEmptyCollectionException(UArrayList al) { // test all methods that would throw the exception // including first(), last(), remove(), removeLast(), // add your code here
}
public static void testNoSuchElementException(UArrayList al) { // test all methods that throw the exception // add your code here
}
public static void addToRearAndFrontTest(UArrayList al) { System.out.println(" ****Testing add and addToFront methods - ****"); System.out.println("List [] : " + al); System.out.println("*Add B, then A"); al.add("B"); al.add("A"); System.out.println("List [B A] : " + al); // System.out.println("*Add W to front, then add J to front"); // al.addToFront("W"); // al.addToFront("J"); // System.out.println("List[J W B A] : " + al); }
public static void testAddAfter(UArrayList al) { System.out.println(" ****Testing addAfter method****");
al.clear(); al.add("J"); al.add("W"); al.add("B"); al.add("A"); System.out.println("List[J W B A] : " + al);
al.addAfter("W", "Z"); System.out.println("List[J W Z B A] : " + al);
al.addAfter("B", "K"); System.out.println("List[J W Z B K A] : " + al);
System.out.println("Size [6]: " + al.size());
System.out .println(" ****Testing addAfter -- should throw exceptions ****"); // add your code here
}
private static void testAddAt(UArrayList al) { // test scenarios that are valid, as well as scenarios that throw the // exception // add your code here
}
private static void testRemoveMethods(UArrayList al) { System.out.println(" ****Testing remove methods****"); // test all remove options, some that work, some that throw exceptions // you don't need to test remove from empty list - that should be part of // testEmptyCollectionException
System.out.println("List[J W B A] : " + al); al.removeFirst(); System.out.println("List[W B A] : " + al); System.out.printf("Size[3]: %d, last[A]: %s ", al.size(), al.last());
// add your code here
}
}
UArrayList Code:
import java.util.ConcurrentModificationException; import java.util.Iterator; import java.util.NoSuchElementException;
public class UArrayList extends AArrayList implements UnorderedListADT {
// Append element to list (at the end) // allocate larger array when list is full @Override public boolean add(T element) { if (isFull()) // list is full growList();
listArray[listSize++] = element; modifiedCount++; return true; }
// Add element to front of the list // allocate larger array when list is full @Override public void addToFront(T element) { // TODO Auto-generated method stub if (isFull()) // list is full growList();
// loop to shift the elements to right by 1 position for (int i = listSize; i > 0; i--) listArray[i] = listArray[i - 1]; listArray[0] = element; // insert element at index 0 listSize++; // increment listSize }
// Add element after an existing element in the list // Throw NoSuchElementException if existingElement is not found in the list @Override public void addAfter(T existingElement, T element) throws NoSuchElementException { if(isFull()) // list is full growList();
// loop over the list for(int i=0;i {
if(listArray[i].equals(existingElement)) // existingElement found, insert element at index i+1 { // loop to shift the elements to right by 1 position from index i+1 to end for(int j=listSize;j>i+1;j--) listArray[j] = listArray[j-1]; listArray[i+1] = element; // insert element at index i+1 listSize++; // increment listSize return; // return from method } }
throw new NoSuchElementException(); // existingElement not found }
// Add element at specified index location // Throw IndexOutOfBoundsException if index is invalid public void addAt(int index, T element) throws IndexOutOfBoundsException { if (index listSize - 1) // check for index value it must be // between 0 and number of elements-1 throw new IndexOutOfBoundsException(); // if the above condition is true if (isFull()) // if list is full then growlist growList();
for (int j = listSize - 1; j >= index; j--) // move the elements forward { listArray[j + 1] = listArray[j]; // moving the elements the elements one // step forward } listArray[index] = element; // insert the given element at the specified index
}
}
public abstract class AArrayList
// Constructors // Create a new list object with maximum size "size" @SuppressWarnings("unchecked") // Generic array allocation AArrayList(int size) { // maxSize = size; listSize = modifiedCount = 0; listArray = (T[]) new Object[size]; // Create listArray }
// Create a list with the default capacity AArrayList() { this(DEFAULT_SIZE); // Just call the other constructor }
// Reset the list -- note that we could also iterate the array and null all // values public void clear() { // Reinitialize the list listSize = modifiedCount = 0; // Simply reinitialize values }
@Override // the add method will be different for each subclass public abstract boolean add(T element);
// Increase the size of the array when it becomes full protected void growList() { @SuppressWarnings("unchecked") T[] newList = (T[]) (new Object[size() + DEFAULT_SIZE]);
for (int i = 0; i
listArray = newList; }
@Override public T remove(T element) throws NoSuchElementException { if (isEmpty()) // list is empty throw NoSuchElementException throw new NoSuchElementException(); else { // loop over the list searching for element for (int i = 0; i
@Override public T removeFirst() { // Remove first element from the list T retElement; if (isEmpty()) throw new EmptyCollectionException("list");
retElement = listArray[FRONT];
// shift elements for (int i = 0; i
listArray[listSize] = null; // null where the last element used to be listSize--; modifiedCount++;
return retElement; }
@Override public T removeLast() throws NoSuchElementException { if (isEmpty()) // empty list, throw NoSuchElementException throw new NoSuchElementException();
listSize--; // decrement listSize by 1, thus removing the last element from list return listArray[listSize]; // return the last element }
@Override public int size() { return listSize; // Return list size } public boolean isEmpty() { return listSize == 0; }
protected boolean isFull() { return (listSize == listArray.length); }
@Override public T first() { // if the list is empty, throw an exception if (isEmpty()) throw new EmptyCollectionException("list"); // returns the first element in the list return listArray[0]; }
@Override public T last() { if (isEmpty()) throw new EmptyCollectionException("list"); return listArray[listSize - 1]; }
@Override public boolean contains(T element) { // iterate through the list length for (int i = 0; i
@Override public int indexOf(T element) { for (int i = 0; i
@Override public void toArray(T[] anArray) { int k = 0; if (anArray == null) { throw new NullPointerException(); } if (this.listSize > anArray.length) { // Throw the ArrayCapcityException by importing it in code ,below } for (int i = FRONT; i
@Override public String toString() { String retString = "[";
for (int i = 0; i
// You may use this method to shift elements and open an index location to add protected void shiftElements(int insertingIndex) { for (int i = size(); i > insertingIndex; i--) { listArray[i] = listArray[i - 1]; } listArray[insertingIndex] = null; }
@Override public Iterator
private class ArrayListIterator implements Iterator
int currentPos; int currentModCount;
public ArrayListIterator() { currentPos = 0; currentModCount = modifiedCount;
}
@Override public boolean hasNext() { // Indicate if there are elements left to iterate if (currentModCount != modifiedCount) { throw new ConcurrentModificationException(); } return (currentPos
@Override public T next() {
if (hasNext()) { currentPos++; return listArray[currentPos - 1]; } else { throw new NoSuchElementException();
Complete the UnorderedArrayListTester Use the tester that is provided and expand it to complete the methods 1. testObserver Methods() Complete the UnorderedArrayListTester Use the tester that is provided and expand it to complete the methods 1. testObserver Methods()Step by Step Solution
There are 3 Steps involved in it
Step: 1
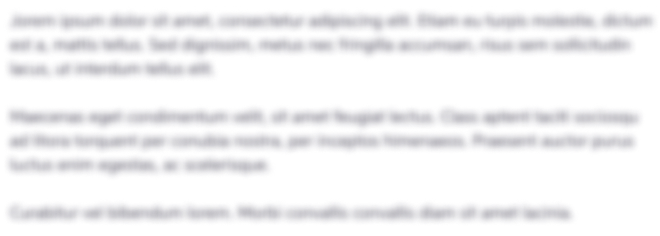
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started