Question
Thank you in advance for the help, here is our assignment: We are expected to write the above code in C language in a linux
Thank you in advance for the help, here is our assignment:
We are expected to write the above code in C language in a linux terminal.
Here is the md5.c file, implement code in the md5sum method:
#include #include #include #include #include char* md5sum(char* payload,size_t sz);
int main(int argc,char* argv[]) { char* fName = argv[1]; FILE* src = fopen(fName,"r"); fseek(src,0,SEEK_END); long sz = ftell(src); fseek(src,0,SEEK_SET); char* payload = malloc(sizeof(char)*sz); fread(payload,sizeof(char),sz,src); fclose(src); char* rv = md5sum(payload,sz); printf("Got md5 [%s] ",rv); free(rv); return 0; }
void sendPayload(int fd,char* payload,size_t sz) { int sent=0,rem = sz; while(sent != sz) { int nbSent = write(fd,payload+sent,rem); sent += nbSent; rem -= nbSent; } }
char* readResponse(int fd) { int sz = 8; char* buf = malloc(sz); int ttl = 0,at = 0; int recvd; do { recvd = read(fd,buf+at,sz - at); ttl += recvd; at += recvd; if (recvd > 0 && ttl == sz) { buf = realloc(buf,sz*2); sz *= 2;
} } while (recvd >0 ); char* final = malloc(ttl+1); memcpy(final,buf,ttl); final[ttl] = 0; free(buf); return final; } // Implement your solution here // /* // 1. Forking child process // 2. Bi-directional pipes w/ appropriate std i/o // 3. child exec md5Sum and upgrades // 4. parent sends content of file (given from command arg) to child and gets back md5Sum char* md5sum(char* payload,size_t sz) { }
The purpose of this lab is to get some practice with communication between processes via pipes. The MDS hash (or checksum) is a 128-bit digital fingerprint that can be computed for a file using the md5sum program. Such fingerprints are commonly used for detecting files corrupted, e.g., during transfer over the internet, since the chance that a corrupted file results in the same MD5 hash as the original file is very low. For this lab you need to implement a function that computes the MD5 hash. Since the md5sum program is installed by default on Linux systems you can call it from your code to compute the MD5 hash. Test it at the command line, e .g., Dy running echo "Hello world" | md5sum and you will notice that without parameters md5sum reads the data it receives on its standard input and produces the MD5 hash on its standard output. The implemented function must work even when the file system is mounted read only, so you cannot use temporary files to get the MD5 hash returned by the call to md5sum. How do you do it? The idea is to have your function set up two pipes to establish bi-directional communication with a forked child process. Naturally, the child process must hookup the respective ends of the pipe to its standard input and output. The parent process then merely sends the file to the child using one pipe, gets the MD5 hash back from the other pipe, and then waits on the child to reap it. Problem solved! Your task is to implement the function char* md5sum (char* payload, size t sz) Note that it receives a byte array with the payload, its size (in bytes) and outputs a pointer to a freshly allocated buffer holding the string with the MD5 hash (the caller of md5sum should release the buffer of course). In the file, you wil find two convenience functions sendPayload to send the actual payload over a file descriptor and readResponse to pick up in a fresh buffer the MD5 hash - as a string - from a file descriptor The purpose of this lab is to get some practice with communication between processes via pipes. The MDS hash (or checksum) is a 128-bit digital fingerprint that can be computed for a file using the md5sum program. Such fingerprints are commonly used for detecting files corrupted, e.g., during transfer over the internet, since the chance that a corrupted file results in the same MD5 hash as the original file is very low. For this lab you need to implement a function that computes the MD5 hash. Since the md5sum program is installed by default on Linux systems you can call it from your code to compute the MD5 hash. Test it at the command line, e .g., Dy running echo "Hello world" | md5sum and you will notice that without parameters md5sum reads the data it receives on its standard input and produces the MD5 hash on its standard output. The implemented function must work even when the file system is mounted read only, so you cannot use temporary files to get the MD5 hash returned by the call to md5sum. How do you do it? The idea is to have your function set up two pipes to establish bi-directional communication with a forked child process. Naturally, the child process must hookup the respective ends of the pipe to its standard input and output. The parent process then merely sends the file to the child using one pipe, gets the MD5 hash back from the other pipe, and then waits on the child to reap it. Problem solved! Your task is to implement the function char* md5sum (char* payload, size t sz) Note that it receives a byte array with the payload, its size (in bytes) and outputs a pointer to a freshly allocated buffer holding the string with the MD5 hash (the caller of md5sum should release the buffer of course). In the file, you wil find two convenience functions sendPayload to send the actual payload over a file descriptor and readResponse to pick up in a fresh buffer the MD5 hash - as a string - from a file descriptorStep by Step Solution
There are 3 Steps involved in it
Step: 1
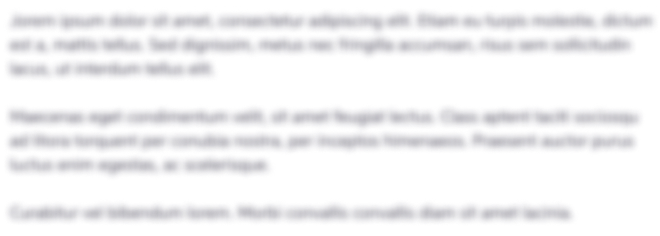
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started