The advanced sorts like merge sort (O(n log2 n) in the worst-case) are faster than the simple sorts (O(n2 )) when n is large, but
The advanced sorts like merge sort (O(n log2 n) in the worst-case) are faster than the simple sorts (O(n2 )) when n is large, but the simple sorts are actually faster when n is small. The original merge sort code in project6/mergesort.py continues to split the list in two if its length is bigger than 1. For Part B, I want you to improve merge sort by not splitting the list down if it is faster to do a simple sort (i.e., selection sort) Use the project6/compareSorts.py program to experimenting determine the threshold of when the selection sort (a simple sort) is faster on randomly ordered lists than merge sort on your computer. THEN, implement an improved merge sort that utilizes selection sort on small (i.e., less than your threshold) length lists, but continues to recursively split the list in two if it is bigger than the threshold. The diagram below illustrates the improved versions behavior:
The code for the previously mentioned programs are
MergeSort
def mergeSort(alist): if len(alist)>1: mid = len(alist)//2 lefthalf = alist[:mid] righthalf = alist[mid:]
mergeSort(lefthalf) mergeSort(righthalf) merge(alist, lefthalf, righthalf)
def merge(alist, lefthalf, righthalf): """ Merge the sorted list lefthalf and righthalf back into alist in sorted order.""" i=0 j=0 k=0 while i while i while j CompareSort import time import random from mergesort import mergeSort from selectionSort import selectionSort def main(): n = int(input("Enter the number of items you would like to sort: ")) randomLists1 = [] randomLists2 = [] for count in range(1000): list1 = [] list2 = [] for value in range(n): rand = random.randint(1,1000) list1.append(rand) list2.append(rand) randomLists1.append(list1) randomLists2.append(list2) start = time.perf_counter() for count in range(1000): mergeSort(randomLists1[count]) endSort = time.perf_counter() #print ("Sorted List: ",myList) print ("Total merge sort time of", n, "random items",endSort - start) start = time.perf_counter() for count in range(1000): selectionSort(randomLists2[count]) endSort = time.perf_counter() #print ("Sorted List: ",myList) print ("Total selection sort time of", n, "random items",endSort - start) main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
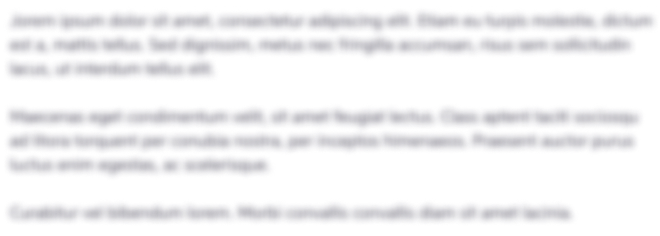
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started