Question
The array in arrayListType is being used as a partially filled array which means that items are stored from the beginning to the end of
The array in arrayListType is being used as a partially filled array which means that items are stored from the beginning to the end of the array with no gaps in between them. This restriction becomes part of the class invariant for the arrayListType and unorderedArrayListType classes as follows:
Items can be stored in the array in any order.
The length private instance variable keeps track of the number of items currently in the list.
New items are added at the location represented by length.
No gaps are allowed between items.
It doesn't matter what values are stored in locations beyond length.
The number of items in the array cannot exceed the value stored in the maxSize private instance variable.
Also note that the instance variables in the arrayList class are specified with the access type protected, meaning that they can be used without going through the public access functions by classes derived from this class. The public accessor functions must still be used by entities outside of the class and its derived classes.
Take the files for the arrayListType and unorderedArrayListType classes that are in the Chapter 12/Source Code From Textbook/arrayList ADT folder on the Blackboard, create a project and make the following modifications:
The function retrieveAt of the class arrayListType is written as a void function. Rewrite this function so that it is written as a value returning function, returning the required item. If location of the item to be returned is out of range, use the assert function (part of the
The function removeAt of the class arrayListType removes an element from the list by shifting the elements of the list. However, if the element to be removed is at the beginning of the list and the list is fairly large it could take a lot of computer time. Because the list elements are in no particular order, you could simply remove the element by swapping the last element of the list with the item to be removed and reducing the length of the list. Rewrite the definition of the function removeAt using this technique. Use the class unorderedArrayListType to test your function.
The function remove of the class arrayListType removes only the first occurrence of an element. Add the function removeAll as a pure virtual function in the class arrayListType, which would remove all occurrences of a given element. Also, write the definition of the function removeAll in the class unorderedArrayListType.
Write one or more programs to test your modifications. Turn in all your header files, all your implementation files, and all your test programs. Also turn in one or more screen shots showing the results of your testing.
Upon completion of this project you will demonstrate that you can:
Define the term partially filled array and explain how it is used to maintain the class invariant.
Utilize the class invariant to simplify the processing of member functions.
Modify and build member functions that maintain the class invariant.
Apply the assert function to terminate processing from inside a class.
Add a pure virtual function to an abstract class
Construct a pure virtual function in a derived class.
Design a program that utilizes a class derived from an abstract base class.
Utilize protected variables in a derived class.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
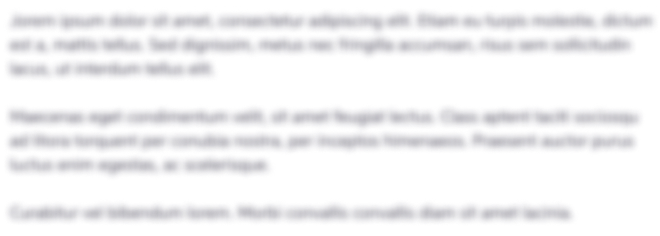
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started