Question
The arrays list1 and list2 are identical if they have the same size and content only (same content with different order still consider as identical).
The arrays list1 and list2 are identical if they have the same size and content only (same content with different order still consider as identical). Write a method that returns 1 if list1 and list2 are identical, using following header:
public int equals(int[] list1, int[] list2)
The program will get the data as an string. So you need to convert the String to int[] array using the following headers:
public int equals(String list1, String list2)
public int[] convert(String sArray)
Here are the sample runs.
Note: The first number is the size of the array followed by array elements separated by single space.
Input1
5 2 -5 6 6 1
5 -5 2 6 1 6
Output1
1
---------------------
Input2
5 5 5 6 6 1
5 2 5 6 6 1
Output2
0
----------------------
Input3
5 -5 5 6 6 1
5 2 5 6 6 1
Output3
0
**** The above is the problem, and written below is my attempt to solve it. I keep getting the following errors on Eclipse.
Exception in thread "main" java.util.NoSuchElementException
at java.util.Scanner.throwFor(Scanner.java:862)
at java.util.Scanner.next(Scanner.java:1485)
at java.util.Scanner.next(Scanner.java:1418)
at HW3_P1.convert(HW3_P1.java:15)
at HW3_P1.equals(HW3_P1.java:24)
at Driver.main(Driver.java:16)
I belive these errors are related to the fact that the input has spaces and I am not properly handling that.
What do I do to fix thi???
***** CLASS*****
import java.util.Arrays;
import java.util.StringTokenizer;
import java.util.Scanner;
public class HW3_P1 {
public int[] convert(String sArray){
int size = sArray.charAt(0);
int [] intArray = new int[size];
//intArray[i] = Integer.parseInt(String.valueOf(sArray.charAt(i)));
for(int i=2; i intArray[i] = Integer.parseInt(String.valueOf(sArray.charAt(i))); } return intArray; } //method overloading public int equals(String list1, String list2){ //write your code that convert the String list to int[] using the convert method convert(list1); convert(list2); //and call the equals method again return equals(list1, list2); } public int equals(int[] list1, int[] list2){ //Write your code to compare the int[] arrays Arrays.sort(list1); Arrays.sort(list2); int identical=0; for(int c = 0; c < list1.length; c++) { if(list1[c] != list2[c]) { identical = 0; }else { identical = 1; } } //return 1 if they are identical otherwise 0 return identical; } } import java.util.*; //import java.lang.*; //import java.io.*; ****** MAIN******* public class Driver { public static void main(String args[]){ HW3_P1 hw3P1 = new HW3_P1(); Scanner input = new Scanner(System.in); // get the user input as a string String sArray1 = input.nextLine(); String sArray2 = input.nextLine(); input.close(); System.out.print(hw3P1.equals(sArray1,sArray2)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
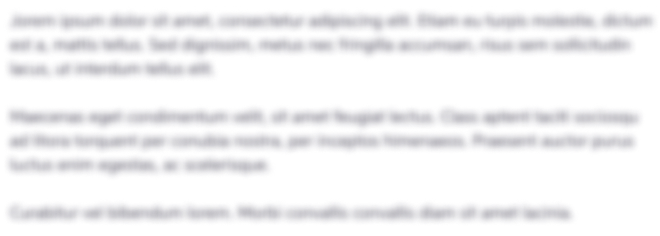
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started