Question
The assignment for my Application Development class has us creating an office system for a dentist. The following requirements are: It must display the current
The assignment for my Application Development class has us creating an office system for a dentist. The following requirements are:
It must display the current lists for Dentists, Assistants, Patients, and Services
It must allow the user to add/edit/or delete items from this list
It must display an invoice for the customer based on the chosen services
I asked this yesterday and the answer I got helped immensely. I now have a questions on the exact code layout.
Namely, what would the code have to look like for case 5 in order to actually display the list as well as the changes made to them?
import java.util.Scanner; import java.util.ArrayList; public class OfficeSystem { public static void main (String[] args) { Scanner sc = new Scanner (System.in); ArrayList patients = new ArrayList(); ArrayList doctors = new ArrayList(); ArrayList assistants = new ArrayList(); ArrayList services = new ArrayList(); Patient pat1 = new Patient (1,"James","Mack","123 ABC Street","Blue Cross"); Patient pat2 = new Patient (2,"Mark","Smith","456 DEF Street","United Health"); Patient pat3 = new Patient (3,"Doug","Barker","789 GHI Avenue","Kaiser Permanente"); patients.add(pat1); patients.add(pat2); patients.add(pat3); Doctor doc1 = new Doctor (1,"Ben","Kenobi","555 Certain Point View","DDS"); Doctor doc2 = new Doctor (2,"Darth","Vader","777 Mustafar Street","DMD"); Doctor doc3 = new Doctor (3,"Luke","Skywalker","333 Toshe Station","DDS"); Doctor doc4 = new Doctor (4,"Han","Solo","111 Millenium Drive","DMD"); doctors.add(doc1); doctors.add(doc2); doctors.add(doc3); doctors.add(doc4); Assistant assist1 = new Assistant (1,"Leia","Organa","555 Alderaan Drive","Dr. Solo"); Assistant assist2 = new Assistant (2,"Poe","Dameron","777 Resistance Blvd","Dr. Skywalker"); Assistant assist3 = new Assistant (3,"Rey","Unknown","2187 Neema Outpost","Dr. Kenobi"); assistants.add(assist1); assistants.add(assist2); assistants.add(assist3); Service serv1 = new Service (1,"Teeth Cleaning","Dr. Vader",7); Service serv2 = new Service (2,"Root Canal","Dr. Kenobi",12); Service serv3 = new Service (3,"Cavity Filling","Dr. Skywalker",20); Service serv4 = new Service (4,"Gum Cleaning","Dr. Kenobi",15); Service serv5 = new Service (5,"Tooth Pulling","Dr. Solo",15); services.add(serv1); services.add(serv2); services.add(serv3); services.add(serv4); services.add(serv5); System.out.println("***************************************************************"); System.out.println(" Kennesaw Dental Office "); System.out.println("***************************************************************"); System.out.println("1. Display Patient List"); System.out.println("2. Display Doctor List"); System.out.println("3. Display Assistant List"); System.out.println("4. Display Service Fees"); System.out.println("5. Edit Patient List"); System.out.println("6. Edit Doctor List"); System.out.println("7. Edit Assistant List"); System.out.println("8. Edit Service Fees"); System.out.println("9. Issue Patient Invoice"); System.out.println("0. Exit the Program"); System.out.println("Please select an option: "); int option = sc.nextInt(); switch(option) { case 1: System.out.println("***************************************************************************"); System.out.println(" Patients "); System.out.println("***************************************************************************"); break; case 2: System.out.println("***************************************************************************"); System.out.println(" Doctors "); System.out.println("***************************************************************************"); break; case 3: System.out.println("***************************************************************************"); System.out.println(" Assistants "); System.out.println("***************************************************************************"); break; case 4: System.out.println("***************************************************************************"); System.out.println(" Service Fees "); System.out.println("***************************************************************************"); break; case 5: System.out.println("***************************************************************************"); System.out.println(" Patients "); System.out.println("***************************************************************************"); System.out.println("Please enter an option 1-4: "); System.out.println("1. Add a new entry"); System.out.println("2. Edit an existing entry"); System.out.println("3. Delete an entry"); System.out.println("4. Return to main menu"); int patientChoice = sc.nextInt(); switch (patientChoice) { case 1: System.out.println("Please enter the patient information in the following format: "); System.out.println("Patient # First Name Last Name Address Insurance Provider"); break; case 2: System.out.println("Please enter the number of the patient you would like to edit: "); System.out.println("Please enter the patient information in the following format:"); System.out.println("Patient # First Name Last Name Address Insurance Provider"); break; case 3: System.out.println("Please enter the number of the patient you would like to delete: "); break; case 4: break; } break; case 6: System.out.println("***************************************************************************"); System.out.println(" Doctors "); System.out.println("***************************************************************************"); System.out.println("Please enter an option 1-4: "); System.out.println("1. Add a new entry"); System.out.println("2. Edit an existing entry"); System.out.println("3. Delete an entry"); System.out.println("4. Return to main menu"); int doctorChoice = sc.nextInt(); switch (doctorChoice) { case 1: System.out.println("Please enter the doctor information in the following format:"); System.out.println("Doctor # First Name Last Name Address Specialization"); break; case 2: System.out.println("Please enter the number of the doctor you would like to edit: "); System.out.println("Please enter the doctor information in the following format:"); System.out.println("Doctor # First Name Last Name Address Specialization"); break; case 3: System.out.println("Please enter the number of the doctor you would like to delete: "); break; case 4: break; } break; case 7: System.out.println("***************************************************************************"); System.out.println(" Assistants "); System.out.println("***************************************************************************"); System.out.println("Please enter an option 1-4: "); System.out.println("1. Add a new entry"); System.out.println("2. Edit an existing entry"); System.out.println("3. Delete an entry"); System.out.println("4. Return to main menu"); int assistantChoice = sc.nextInt(); switch (assistantChoice) { case 1: System.out.println("Please enter the assistant information in the following format:"); System.out.println("Assistant # First Name Last Name Address Doctor"); break; case 2: System.out.println("Please enter the number of the assistant you would like to edit: "); System.out.println("Please enter the assistant information in the following format:"); System.out.println("Assistant # First Name Last Name Address Doctor"); break; case 3: System.out.println("Please enter the number of the assistant you would like to delete: "); break; case 4: break; } break; case 8: System.out.println("***************************************************************************"); System.out.println(" Service Fees "); System.out.println("***************************************************************************"); System.out.println("Please enter an option 1-4: "); System.out.println("1. Add a new entry"); System.out.println("2. Edit an existing entry"); System.out.println("3. Delete an entry"); System.out.println("4. Return to main menu"); int serviceChoice = sc.nextInt(); switch (serviceChoice) { case 1: System.out.println("Please enter the service information in the following format:"); System.out.println("Service # Service Title Doctor Service Price"); break; case 2: System.out.println("Please enter the number of the service you would like to edit: "); System.out.println("Please enter the service information in the following format:"); System.out.println("Service # Service Title Doctor Service Price"); break; case 3: System.out.println("Please enter the number of the service you would like to delete: "); break; case 4: break; } break; } } }
public class Patient
{
private String firstName;
private String lastName;
private String address;
private String insurance;
private int identification;
public Patient (int id, String fname, String lname, String add, String ins)
{
firstName = fname;
lastName = lname;
address = add;
insurance = ins;
identification = id;
}
public void setIdentification (int id)
{
identification = id;
}
public int getIdentification ()
{
return identification;
}
public void setFirstName (String fname)
{
firstName = fname;
}
public String getFirstName ()
{
return firstName;
}
public void setLastName (String lname)
{
lastName = lname;
}
public String getLastName ()
{
return lastName;
}
public void setAddress (String add)
{
address = add;
}
public String getAddress ()
{
return address;
}
public void setInsurance (String ins)
{
insurance = ins;
}
public String getInsurance ()
{
return insurance;
}
}
public class Doctor
{
private String firstName;
private String lastName;
private String address;
private String specialization;
private int identification;
public Doctor (int id, String fname, String lname, String add, String special)
{
firstName = fname;
lastName = lname;
address = add;
specialization = special;
identification = id;
}
public void setIdentification (int id)
{
identification = id;
}
public int getIdentification ()
{
return identification;
}
public void setFirstName (String fname)
{
firstName = fname;
}
public String getFirstName ()
{
return firstName;
}
public void setLastName (String lname)
{
lastName = lname;
}
public String getLastName ()
{
return lastName;
}
public void setAddress (String add)
{
address = add;
}
public String getAddress ()
{
return address;
}
public void setPhoneNumber (String special)
{
specialization = special;
}
public String getSpecialization ()
{
return specialization;
}
}
public class Assistant
{
private String firstName;
private String lastName;
private String address;
private String doctor;
private int identification;
public Assistant (int id, String fname, String lname, String add, String doc)
{
firstName = fname;
lastName = lname;
address = add;
doctor = doc;
identification = id;
}
public void setIdentification (int id)
{
identification = id;
}
public int getIdentification ()
{
return identification;
}
public void setFirstName (String fname)
{
firstName = fname;
}
public String getFirstName ()
{
return firstName;
}
public void setLastName (String lname)
{
lastName = lname;
}
public String getLastName ()
{
return lastName;
}
public void setAddress (String add)
{
address = add;
}
public String getAddress ()
{
return address;
}
public void setDoctor (String doc)
{
doctor = doc;
}
public String getDoctor ()
{
return doctor;
}
}
public class Service
{
private String serviceName;
private String doctor;
private int identification;
private int price;
public Service (int id, String name, String doc, int pr)
{
serviceName = name;
doctor = doc;
identification = id;
price = pr;
}
public void setIdentification (int id)
{
identification = id;
}
public int getIdentification ()
{
return identification;
}
public void setServiceName (String name)
{
serviceName = name;
}
public String getServiceName ()
{
return serviceName;
}
public void setDoctor (String doc)
{
doctor = doc;
}
public String getDoctor ()
{
return doctor;
}
public void setPrice (int pr)
{
price = pr;
}
public int getPrice ()
{
return price;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
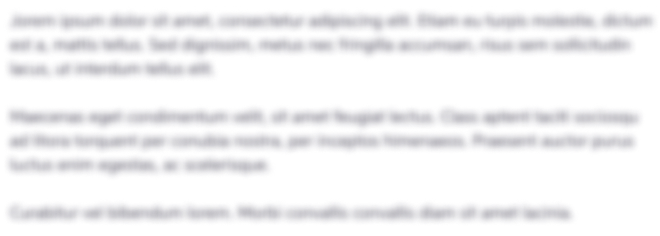
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started