Question
The assignment is to implement ThreeSum program and its experiments. The program will also create plots based on the experimental data. Use both the data
The assignment is to implement ThreeSum program and its experiments. The program will also create plots based on the experimental data. Use both the data and plots to create a one page report which accouts for observations and a hypothesis established through the experiments using power law. You can either design your own algorithm or use the Java class ThreeSum program in the textbook page 173 to do the experiments. The booksite provides convenient libraries and classes ready to be used. You will notice that in the main() method, an In class object was created to take a file containing a list of items as input to the program. The object then read the list of items as integers onto an integer array. Once the program got the answer from invoking count() method, StdOut class object is used to print the output. Both of In and StdOut classes are among many convenient utilities the booksite offered, so that when writing code, programmers can be more focused on realizing algorithms then applying programming language syntaxes. In order to use classes the booksite provided, you need to download both stdlib.jar and algs4.jar (included with the assignment instructions) to be compiled and run with your programs. To setup automatic experiments, the DoublingTest program on textbook page 177 can be used. To draw diagrams, use StdDraw class, from the booksite, to produce plots of the experimental data. Several sample usages can be observed from the examples on page 45. More API usages can be found from the booksite at http://algs4.cs.princeton.edu/home/. You can find all API documents for all the classes from the book at http://algs4.cs.princeton.edu/code/javadoc/. Create both standard and log-log plots, similar to the ones in the textbook for your experiments. Rescale as necessary so that the plot always fills a substantial portion of the whole diagram.
I have to print out those numbers and put them on a line graph but I'm stuck when I print it. I only get the 70 seconds and I can't figure out how to graph it on a line plot
A program with cubic running time. Reads n integers * and counts the number of triples that sum to exactly 0 * (ignoring integer overflow). * * % java ThreeSum 1Kints.txt * 70 * * % java ThreeSum 2Kints.txt * 528 * * % java ThreeSum 4Kints.txt * 4039 * ******************************************************************************/ /** * The {@code ThreeSum} class provides static methods for counting * and printing the number of triples in an array of integers that sum to 0 * (ignoring integer overflow). *
* This implementation uses a triply nested loop and takes proportional to n^3, * where n is the number of integers. *
* For additional documentation, see Section 1.4 of * Algorithms, 4th Edition by Robert Sedgewick and Kevin Wayne. * * @author Robert Sedgewick * @author Kevin Wayne */ public class ThreeSum { // Do not instantiate. private ThreeSum() { } /** * Prints to standard output the (i, j, k) with {@code i < j < k} * such that {@code a[i] + a[j] + a[k] == 0}. * * @param a the array of integers */ public static void printAll(int[] a) { int n = a.length; for (int i = 0; i < n; i++) { for (int j = i+1; j < n; j++) { for (int k = j+1; k < n; k++) { if (a[i] + a[j] + a[k] == 0) { StdOut.println(a[i] + " " + a[j] + " " + a[k]); } } } } } /** * Returns the number of triples (i, j, k) with {@code i < j < k} * such that {@code a[i] + a[j] + a[k] == 0}. * * @param a the array of integers * @return the number of triples (i, j, k) with {@code i < j < k} * such that {@code a[i] + a[j] + a[k] == 0} */ public static int count(int[] a) { int n = a.length; int count = 0; for (int i = 0; i < n; i++) { for (int j = i+1; j < n; j++) { for (int k = j+1; k < n; k++) { if (a[i] + a[j] + a[k] == 0) { count++; } } } } return count; } /** * Reads in a sequence of integers from a file, specified as a command-line argument; * counts the number of triples sum to exactly zero; prints out the time to perform * the computation. * * @param args the command-line arguments */ public static void main(String[] args) { In in = new In(args[0]); int[] a = in.readAllInts(); Stopwatch timer = new Stopwatch(); int count = count(a); StdOut.println("elapsed time = " + timer.elapsedTime()); StdOut.println(count); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
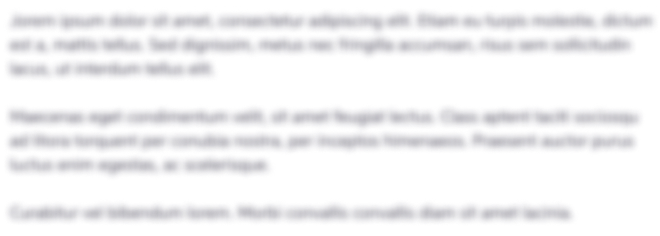
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started