Question
The C library function memset(p,c,n) writes (uint8_t)(c) repeatedly n times begininig at the address held in pointer p. Sometimes it is more efficient or convenient
The C library function memset(p,c,n) writes (uint8_t)(c) repeatedly n times begininig at the address held in pointer p. Sometimes it is more efficient or convenient to copy a multibyte quantity instead of a single byte one. Implement the following function.
void memset16(void *b, int num_bytes, void *pattern16)
In this function, b has the address of a memory buffer that is num_bytes long. The function should repeatedly copy the 16 byte pattern that pattern16 points at into the memory buffer until num_bytes have been written. If num_bytes is not a multple of 16, the final wriite of the 16 byte pattern should be truncated to finish filling the buffer.
For example if the 16 bytes that pattern16 points at is 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff, then memset(b, 20, pattern16) should write to the buffer pointed at by p the 20 bytes 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff 00 11 22 33.
Use SSE instructions to improve efficiency. Here's pseudocode.
x = SSE unaligned load from pattern16 while (num_bytes >= 16) SSE unaligned store x to p advance p by 16 bytes decrement num_bytes by 16 while (num_bytes > 0) store 1 byte from pattern16 to p advance p by 1 byte advance pattern16 by 1 byte decrement num_bytes by 1
NOTE: There are alternatives to this pseudocode. It is only for demonstartion purposes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
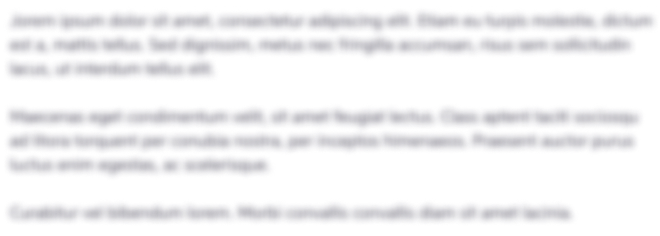
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started