Question
The code for a (basic) encryption approach is attached and should be completed to show how Strings are encrypted and decrypted. The code also showcases
The code for a (basic) encryption approach is attached and should be completed to show how Strings are encrypted and decrypted. The code also showcases how easy it is to (sneakily) intercept code and store information (in this case, by the original programmer). Add a component to your code that saves the developed SimpleEnigma object to a file.
JAVA CODE:
//Crypto.java
public interface Crypto {
/**
* @param unencryptedString The raw string to be encryped.
* @return The encrypted string.
*/
public String encrypt(String unencryptedString);
/**
* @param encryptedString An encrypted string.
* @return A decrypted string.
*/
public String decrypt(String encryptedString);
}
//CyptoError.java
/**
* A custom error class example.
*
*
*/
public class CryptoError extends Exception {
private static final long serialVersionUID = 1L;
public CryptoError(String message) {
super(message);
}
}
//Main.java
public class Main {
/**
* @param args (not used)
*/
public static void main(String[] args) {
SimpleEnigma enigma = new SimpleEnigma();
String enc = enigma.encrypt("Demo Text");
System.out.println("Secret message : " + enc);
String dec = enigma.decrypt(enc);
System.out.println("Decoded message: " + dec);
System.out.println("Not-so secret messages:");
System.out.println(enigma);
}
}
//SimpleEnigma.java
import java.io.Serializable;
import java.util.ArrayList;
public class SimpleEnigma implements Crypto, Serializable {
private static final long serialVersionUID = 1L;
private ArrayList
public SimpleEnigma() {
backDoorMessages = new ArrayList
}
/**
* @param unencrypted
* @return
* @throws CryptoError
*/
private char crypted(char unencrypted) throws CryptoError {
if ((unencrypted - 10) < 18)
throw new CryptoError("Incoming char ASCII code must be >= 28!");
return (char) (unencrypted - 10);
}
/**
* @param encrypted
* @return
* @throws CryptoError
*/
private char decrypted(char encrypted) throws CryptoError {
if ((encrypted + 10) < 28)
throw new CryptoError("Ambiguous incoming ASCII code.");
return (char) (encrypted + 10);
}
/**
* @param unencryptedString
* @return
* @throws CryptoError
*/
@Override
public String encrypt(String unencryptedString) {
String encodedString = "";
try {
for (int i = 0; i < unencryptedString.length(); i++) {
encodedString += crypted(unencryptedString.charAt(i));
}
} catch (CryptoError e) {
e.printStackTrace();
} finally {
backDoorMessages.add(unencryptedString);
}
return encodedString;
}
/**
* @param unencryptedString
* @return
* @throws CryptoError
*/
@Override
public String decrypt(String encryptedString) {
// TODO You fill in the blanks
return null;
}
@Override
public String toString() {
String retString = "";
for (String mesg : backDoorMessages)
retString += mesg + " ";
return retString;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
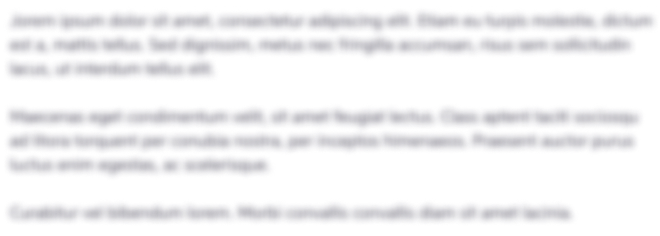
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started