Question
the code given below is for the 4*5 matrix i need help doing same with the 5*6 matrix. Write an assembly language program that accumulates
the code given below is for the 4*5 matrix i need help doing same with the 5*6 matrix.
Write an assembly language program that accumulates and reports the sales for the Albatross Corporation. The Albatross Company has four salespeople who sell five different products. The four salesperson are each identified by a number from 1 to 5. Each product is identified by a number from 1 to 6. Sales are reported by entering the salespersons number, the quantity sold, and the product number. For example, to report that salesperson 2 sold 100 units of product 4, you would enter 2 100 4.
Your program must store the sales data in a two-dimensional array (5 rows by 6 columns). Each row represents a salesperson. Each column represents a product. Initialize the array to zeroes and allow your user to enter data. When the user enters a negative number for a salesman number, terminate the input phase of your program and display a report that shows the total sales for each salesperson and the total sales for each product.
; sales.asm-This program accumulates and reports the sales INCLUDE Irvine32.inc
.data Rowsize= 4 Colsize=5 compSales DWORD 0,0,0,0,0 DWORD 0,0,0,0,0 DWORD 0,0,0,0,0 DWORD 0,0,0,0,0 sno DWORD 0 qty DWORD 0 pno DWORD 0 pmptMsg1 BYTE "Enter salesman number,quanity,product number(Enter -ve number to stop input):",0 msg1 BYTE "Sales matrix :",0 msg2 BYTE "Total sales of salesperson ",0 msg3 BYTE "Total sales of product ",0 .code main PROC ;Read input loop1: mov ebx,OFFSET compSales mov edx,OFFSET pmptMsg1 call WriteString call crlf call readInt ; read salesman number mov [sno],eax ; save salesman number into sno cmp eax,0 ; if input is negative number ; exit the loop and stop input jl endInput call readInt ; read quantity sold mov [qty],eax ; save quantity sold in qty call readInt ; read product number mov [pno],eax ; save the product number in pno ;Save the report into matrix mov eax,sno dec eax mov edi,Colsize mul edi mov edi,pno dec edi add eax,edi mov edi,TYPE DWORD mul edi add ebx,eax ; calculate appropriate location in the ; matrix to save the quantity mov eax,qty mov [ebx],eax ; save the quantity into matrix jmp loop1 ; continue until negative number is entered
endInput:
; display sales matrix mov edx, OFFSET msg1 call WriteString call crlf mov ecx,Rowsize*Colsize mov ebx,OFFSET compSales mov esi,0 L1: mov eax,[ebx+esi] ; get each value from matrix call WriteDec ; print the value mov al,32 ; al= ascii of space character call WriteChar ; print space add esi,4 ; print only 5 values per row cdq mov eax,esi mov edi,20 div edi cmp edx,0 jne next call crlf next: loop L1
; Display sales for each salesmen mov ecx,Rowsize mov esi,0 mov edi,1 L2: mov ebx,OFFSET compSales mov edx,OFFSET msg2 call WriteString mov eax,edi call WriteDec mov al,':' call WriteChar call crlf mov eax,esi call get_row_sum call WriteDec call crlf inc esi inc edi loop L2 call crlf ; Display sales for each product mov ecx,Colsize mov esi,0 mov edi,1 L3: mov ebx,OFFSET compSales mov edx,OFFSET msg3 call WriteString mov eax,edi call WriteDec mov al,':' call WriteChar call crlf mov eax,esi call get_col_sum call WriteDec call crlf inc esi inc edi loop L3 call crlf exit main ENDP ;------------------------------------------------------------------------ ; get_row_sum ; Computes the row sum in the given matrix ; Receives: EBX= offset of the matrix, EAX= salesman number(row number) ; Retruns: EAX = sum of the specified row ;------------------------------------------------------------------------ get_row_sum PROC USES ebx ecx edx esi edi mov edi,Rowsize inc edi mul edi mov edi,TYPE DWORD mul edi add ebx,eax ; starting address of the row mov eax,0 ; sum=0 mov esi,0 ; index=0 mov ecx , Colsize ; loop counter L4: ; beginning of the loop mov edx,[ebx+esi] ; get a row value add eax,edx ; add the row value to sum add esi,4 ; increment the index to point ; next value in the row loop L4 ; continue until ecx is not 0 ret ; return to main get_row_sum ENDP ;------------------------------------------------------------------------ ; get_col_sum ; Computes the column sum in the given matrix ; Receives: EBX= offset of the matrix, EAX= column number(product number) ; Retruns: EAX = sum of the specified column ;------------------------------------------------------------------------ get_col_sum PROC USES ebx ecx edx esi edi mov edi,TYPE DWORD mul edi add ebx,eax ; address of the first value of the column mov eax,0 ; sum=0 mov esi,0 ; index=0 mov ecx , Rowsize ; loop counter L5: ; beginning of the loop mov edx,[ebx+esi] ; get a row value add eax,edx ; add the row value to sum push eax mov eax,Colsize mov edi,TYPE DWORD mul edi add esi,eax pop eax loop L5 ; continue until ecx is not 0 ret ; return to main get_col_sum ENDP END main
The output should look like this and should be on 5*6 matrix
Step by Step Solution
There are 3 Steps involved in it
Step: 1
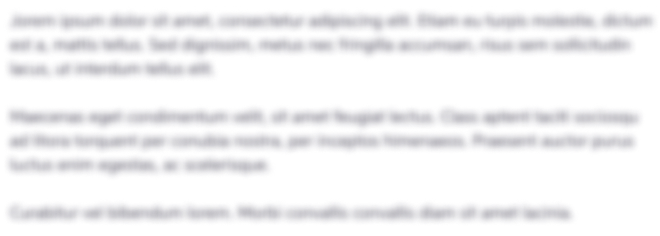
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started