Question
// The code has to be in C++, and please strictly follow the instructions. Task You will develop a class to represent a directory or
// The code has to be in C++, and please strictly follow the instructions. Task
You will develop a class to represent a directory or employees. We provide you with some code to start with in the attached file rec06-orig.cpp. It provides the basic class definitions that we will need for modeling a directory of employees in a company. In particular, it provides the code you will need for the classes Entry and Position, along with a start for the Directory class.
Your job is to:
Read and understand the given code.
Implement the Big 3 (i.e., destructor, copy constructor and assignment operator) for the Directory class. At the beginning of each of these functions, add a print statement to show when you have entered them. This will help you understand when they are each being used.
Overload Directory's [] operator to allow looking up a person's phone number, by passing in their name.
You should (as always) consider if there is any way to further expand the code in main() to test your new features.
We provide a display method for Directory. If you have covered it in class, overload Directory's output operator.
Dynamic Array?
Some might ask, "Why are we using a dynamic array of Entry pointers for our Directory?" Sure, you are [much] more likely to use a vector or other container type (e.g. set), but this provides you with a good exercise in implementing copy control, which is the point of this exercise.
Note that the Directory is responsible for both the Entries and the dynamic array itself.
You should certainly think about how using a vector of pointers would change your code.
Additional resources for assignment rec06-orig.cpp. rec06-orig.cpp is a file that contains the following code. This following code has to be implemented by the class directory that is required to be created. (Opened through TextEdit)
#include
#include
using namespace std;
class Position {
public:
Position(const string& aTitle, double aSalary)
: title(aTitle), salary(aSalary) {}
const string& getTitle() const { return title; }
double getSalary() const { return salary; }
void changeSalaryTo(double d) { salary = d; }
void display(ostream& os = cout) const {
os << '[' << title << ',' << salary << ']';
}
private:
string title;
double salary;
}; // class Position
class Entry {
public:
Entry(const string& aName, unsigned aRoom, unsigned aPhone,
Position& aPosition)
: name(aName), room(aRoom), phone(aPhone), pos(&aPosition) {
}
void display(ostream& os = cout) const {
os << name << ' ' << room << ' ' << phone << ", ";
pos->display(os);
}
const string& getName() const { return name; }
const unsigned& getPhone() const { return phone; }
private:
string name;
unsigned room;
unsigned phone;
Position* pos;
}; // class Entry
class Directory {
public:
void add(const string& name, unsigned room, unsigned ph, Position& pos) {
if (size == capacity) {
// something is missing!!! Add it!
} // if
entries[size] = new Entry(name, room, ph, pos);
++size;
} // add
void display(ostream& os = cout) const {
for (size_t i = 0; i < size; ++i) {
entries[i]->display(os);
os << endl;
}
}
private:
Entry** entries = nullptr;
size_t size = 0;
size_t capacity = 0;
}; // class Directory
void doNothing(Directory dir) { dir.display(); }
int main() {
// Note that the Postion objects are NOT on the heap.
Position boss("Boss", 3141.59);
Position pointyHair("Pointy Hair", 271.83);
Position techie("Techie", 14142.13);
Position peon("Peonissimo", 34.79);
// Create a Directory
Directory d;
d.add("Marilyn", 123, 4567, boss);
d.display();
Directory d2 = d; // What function is being used??
d2.add("Gallagher", 111, 2222, techie);
d2.add("Carmack", 314, 1592, techie);
d2.display();
cout << "Calling doNothing ";
doNothing(d2);
cout << "Back from doNothing ";
Directory d3;
d3 = d2;
// Should display 1592
cout << d2["Carmack"] << endl;
} // main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
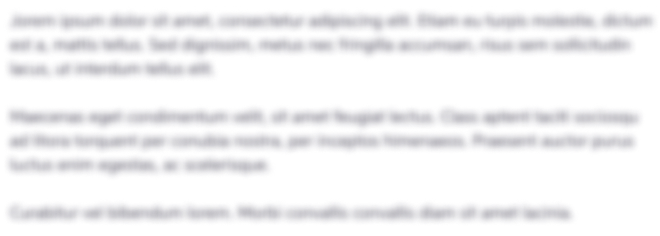
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started