Question
The code Inventory.cpp is almost complete is just has a few errors when I'm trying to build it. Can you please fix these couple errors
The code Inventory.cpp is almost complete is just has a few errors when I'm trying to build it. Can you please fix these couple errors like (Line 70: and Line 130: , etc.). I've provided Inventory.cpp and Inventory.h but Inventory.cpp is the only one that needs to be fixed.
COPYABLE CODES(C++):
//Inventory.cpp (one that needs to be fixed)
#include #include
#include "Inventory.h" using namespace std::rel_ops;
//------------------------------------------------------------------------------ Inventory::Inventory() :Inventory(10) { }
//------------------------------------------------------------------------------ Inventory::Inventory(int n) { this->slots = n;
// this->allItemStacks.reserve(n); // only works for std::vector }
//------------------------------------------------------------------------------ Inventory::Inventory(const Inventory& src) :Inventory(src.slots) { for(const ItemStack& srcItemStack : src){ addItemStackNoCheck(srcItemStack); } /* Inventory::const_iterator srcIt = src.begin();
while(srcIt != src.end()){ addItemStackNoCheck(*srcIt);
it++; } */ }
//------------------------------------------------------------------------------ Inventory::~Inventory() { // Done! Be able to explain why. }
//------------------------------------------------------------------------------ int Inventory::utilizedSlots() const { return allItemStacks.size(); } //------------------------------------------------------------------------------ int Inventory::emptySlots() const { return slots - utilizedSlots(); }
//------------------------------------------------------------------------------ int Inventory::totalSlots() const { return slots; }
//------------------------------------------------------------------------------ bool Inventory::isFull() const { return (occupied == slots); }
//------------------------------------------------------------------------------ Inventory::iterator Inventory::begin() { return allItemStacks.begin(); }
//------------------------------------------------------------------------------ Inventory::iterator Inventory::end() { return allItemStacks.end(); }
//------------------------------------------------------------------------------ Inventory::const_iterator Inventory::begin() const { return allItemStacks.begin(); }
//------------------------------------------------------------------------------ Inventory::const_iterator Inventory::end() const { return allItemStacks.end(); }
//------------------------------------------------------------------------------ void Inventory::display(std::ostream &outs) const { outs << " -Used " << utilizedSlots() << " of " << slots << " slots" << " ";
const_iterator it = this->begin(); while(it != this->end()){ outs << " " << *it << " ";
it++; } // 2 spaces " " before each ItemStack line } //------------------------------------------------------------------------------ Inventory::iterator Inventory::findMatchingItemStackIterator(const ItemStack& itemStack) { iterator it=this->begin();
while(it != this->end()){ if(*it == itemStack){ return it; } it++; }
return this->end(); }
//------------------------------------------------------------------------------ void Inventory::addItemStackNoCheck(ItemStack itemStack) { ItemStack.push_back(itemStack); }
//------------------------------------------------------------------------------ Inventory& Inventory::operator=(Inventory rhs) { std::swap(*this, rhs); return *this; }
//------------------------------------------------------------------------------ void swap(Inventory& lhs, Inventory& rhs) { using std::swap;
swap(lhs.allItemStacks, rhs.allItemStacks); swap(lhs.slots, rhs.slots); }
//------------------------------------------------------------------------------ bool operator==(const Inventory& lhs, const Inventory& rhs) { if (lhs.utilizedSlots() != rhs.utilizedSlots()) { return false; }
if (lhs.emptySlots() != rhs.emptySlots()) { return false; }
// The two Inventory objects have the same number of used & unused slots
using const_iterator = Inventory::const_iterator;
const_iterator lhsIt = lhs.begin(); const_iterator rhsIt = rhs.begin();
while (lhsIt != lhs.end() && rhsIt != rhs.end()) { if (*lhsIt != *rhsIt) { return false; }
lhsIt++; rhsIt++; }
// If the two Inventory objects are identical, both iterators // will have reached end positions. return lhsIt == lhs.end() && rhsIt == rhs.end(); }
//------------------------------------------------------------------------------ void Inventory::mergeStacks(ItemStack& lhs, const ItemStack& rhs) { lhs.addItems(rhs.size()); }
//Inventory.h (completed code)
#ifndef INVENTORY_H_INCLUDED #define INVENTORY_H_INCLUDED
#include #include #include
#include "ItemStack.h"
class Inventory { public: using ItemStackCollection = std::list; // using ItemStackCollection = std::vector;
using iterator = ItemStackCollection::iterator; using const_iterator = ItemStackCollection::const_iterator;
private: /** * All `ItemStack`s in _this_ `Inventory` */ ItemStackCollection allItemStacks; int slots; ///< Capacity
public: /** * Default to 10 slots */ Inventory();
/** * Create an inventory with n slots * * @pre n > 0 */ Inventory(int n);
/** * Duplicate an existing Inventory */ Inventory(const Inventory& src);
/** * Empty all Inventory slots. */ ~Inventory();
/** * Add one or more items to the inventory list * * @return true if *stack* was added and false otherwise */ bool addItems(ItemStack itemStack);
/** * Check the number of used/utilized (i.e., non-empty). */ int utilizedSlots() const;
/** * Check the number of unused (i.e., empty) slots. */ int emptySlots() const;
/** * Retrieve the total size (number of slots in total). */ int totalSlots() const;
/** * Check if this inventory is full * * @return (occupied < slots) // **technically a typo** */ bool isFull() const;
/** * Print a Summary of the Inventory and all Items contained within */ void display(std::ostream& outs) const;
// Begin Iterator Support (begin/end) iterator begin(); iterator end();
const_iterator begin() const; const_iterator end() const; // End Iterator Support
/** * */ Inventory& operator=(Inventory rhs);
/** * Swap the contents of two `Inventory`s * (Yes this should be spelled "ies"). However, we * need to recognize that Inventory is the type of both * `lhs` and `rhs` *
* I am using a friend function here and only here (under protest) *
* [Refer here](http://stackoverflow.com/questions/3279543/what-is-the-copy-and-swap-idiom) */ friend void swap(Inventory& lhs, Inventory& rhs);
private:
/** * Find Node containing and ItemStack with a matching id * * @param itemStack ItemStack for which we want a match * * @return pointer to a Node containing a matching ItemStack * or nullptr if no such Node exists */ iterator findMatchingItemStackIterator(const ItemStack& itemStack);
/** * Add a new ItemStack to an empty Inventory slot. *
* This is simliar to the code we discussed in Review-02 * * When this method is invoked all special cases * have already been covered in `addItems`. *
* Abstraction and Interfaces */ void addItemStackNoCheck(ItemStack itemStack);
public: /** * Merge two item stacks. * * @param lhs item stack where items need to be `add`ed * @param rhs item stack with the *number* of items to add * * @pre lhs.item == rhs.item */ static void mergeStacks(ItemStack& lhs, const ItemStack& rhs); };
//------------------------------------------------------------------------------ inline bool Inventory::addItems(ItemStack itemStack) { iterator matchingIterator = findMatchingItemStackIterator(itemStack);
// A match was found if (matchingIterator != this->end()){ Inventory::mergeStacks(*matchingIterator, itemStack);
return true; }
// There is no space for a new type of `ItemStack` if (this->isFull()) { return false; }
// This is a new type of item and there is plenty of room addItemStackNoCheck(itemStack); return true; }
/** * Print the Inventory through use of the display member function */ inline std::ostream& operator<<(std::ostream& outs, const Inventory& prt) { prt.display(outs); return outs; }
/** * Compare two Inventory objects, without direct access */ bool operator==(const Inventory& lhs, const Inventory& rhs);
#endif
//ItemStack.h
#ifndef ITEMSTACK_H_INCLUDED #define ITEMSTACK_H_INCLUDED #include#include "Item.h" using namespace std::rel_ops; /** * A Homogeneous--i.e., uniform--stack of Items. */ class ItemStack { private: Item item; ///< Item out of which the stack is composed int quantity; ///< Number of items in the stack public: /** * Default to an empty stack composed of Air */ ItemStack(); /** * Create a stack of type *item* * * @param item Item out of which the stack is composed * @param s size of the stack * * @pre (s > 0) */ ItemStack(const Item& item, int s); /** * Retrieve the Item out of which the stack is composed */ Item getItem() const; /** * Retrieve the size of the stack */ int size() const; /** * Increase the size of the stack * * @param a number of items to add * @pre a > 0 */ void addItems(int a); /** * Increase the size of the stack * * @param other ItemStack with the items to move (i.e., steal). * * @pre *this.item == other.item */ void addItemsFrom(const ItemStack& other); /** * Consider two stacks to be the same if * they contain the same type of Item */ bool operator==(const ItemStack& rhs) const; /** * Order stacks based on Item id */ bool operator<(const ItemStack& rhs) const; }; /** * Print the ItemStack directly */ std::ostream& operator<<(std::ostream& outs, const ItemStack& prt); //------------------------------------------------------------------------------ inline Item ItemStack::getItem() const { return this->item; } //------------------------------------------------------------------------------ inline int ItemStack::size() const { return this->quantity; } //------------------------------------------------------------------------------ inline void ItemStack::addItems(int a) { this->quantity += a; } //------------------------------------------------------------------------------ inline bool ItemStack::operator==(const ItemStack& rhs) const { return this->item == rhs.item; } //------------------------------------------------------------------------------ inline bool ItemStack::operator<(const ItemStack& rhs) const { return this->item < rhs.item; } #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
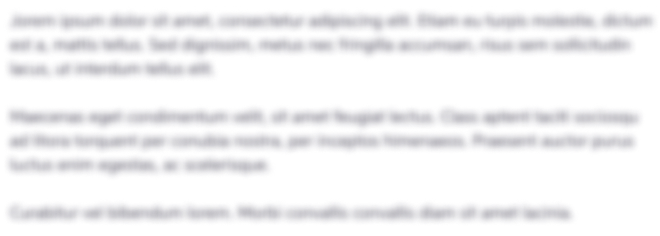
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started