Question
The code is for an app that creates bookmarks. Bookmarks are stored in a sqlite database Perform a unit test for step #4 of barky.py
The code is for an app that creates bookmarks. Bookmarks are stored in a sqlite database
Perform a unit test for step #4 of barky.py "Clears the screen and executes the command corresponding to the users choice" based on these 3 modules
Modules are separated by underscores
___________________________barky.py_______________________________
'''
import os
import commands
class Option:
def __init__(self, name, command, prep_call=None):
self.name = name
self.command = command
self.prep_call = prep_call
def choose(self):
data = self.prep_call() if self.prep_call else None
message = self.command.execute(
data) if data else self.command.execute()
print(message)
def __str__(self):
return self.name
def print_options(options):
for shortcut, option in options.items():
print(f'({shortcut}) {option}')
print()
def option_choice_is_valid(choice, options):
return choice in options or choice.upper() in options
def get_option_choice(options):
choice = input('Choose an option: ')
while not option_choice_is_valid(choice, options):
print('Invalid choice')
choice = input('Choose an option: ')
return options[choice.upper()]
def get_user_input(label, required=True):
value = input(f'{label}: ') or None
while required and not value:
value = input(f'{label}: ') or None
return value
def get_new_bookmark_data():
return {
'title': get_user_input('Title'),
'url': get_user_input('URL'),
'notes': get_user_input('Notes', required=False),
}
def get_bookmark_id_for_deletion():
return get_user_input('Enter a bookmark ID to delete')
def clear_screen():
clear = 'cls' if os.name == 'nt' else 'clear'
os.system(clear)
def loop():
# All steps for showing and selecting options
# https://www.w3schools.com/python/python_dictionaries.asp
options = {
'A': Option('Add a bookmark', commands.AddBookmarkCommand(), prep_call=get_new_bookmark_data),
'B': Option('List bookmarks by date', commands.ListBookmarksCommand()),
'T': Option('List bookmarks by title', commands.ListBookmarksCommand(order_by='title')),
'D': Option('Delete a bookmark', commands.DeleteBookmarkCommand(), prep_call=get_bookmark_id_for_deletion),
'Q': Option('Quit', commands.QuitCommand()),
}
clear_screen()
print_options(options)
chosen_option = get_option_choice(options)
clear_screen()
chosen_option.choose()
_ = input('Press ENTER to return to menu')
# this ensures that this module runs first
if __name__ == '__main__':
commands.CreateBookmarksTableCommand().execute()
# endless program loop
while True:
loop()
______________________________________________________
________________commands.py___________________________
from datetime import datetime
import sys
from database import DatabaseManager
# module scope
db = DatabaseManager('bookmarks.db')
class CreateBookmarksTableCommand:
def execute(self):
db.create_table('bookmarks', {
'id': 'integer primary key autoincrement',
'title': 'text not null',
'url': 'text not null',
'notes': 'text',
'date_added': 'text not null',
})
class AddBookmarkCommand:
def execute(self, data):
data['date_added'] = datetime.utcnow().isoformat()
db.add('bookmarks', data)
return 'Bookmark added!'
class ListBookmarksCommand:
def __init__(self, order_by='date_added'):
self.order_by = order_by
def execute(self):
return db.select('bookmarks', order_by=self.order_by).fetchall()
class DeleteBookmarkCommand:
'''
We also need to remove bookmarks.
'''
def execute(self, data):
db.delete('bookmarks', {'id': data})
return 'Bookmark deleted!'
class QuitCommand:
def execute(self):
sys.exit()
__________________________________________
________database.py________________________
import sqlite3
class DatabaseManager:
def __init__(self, database_filename) -> None:
self.connection = sqlite3.connect(database_filename)
def __del__(self):
self.connection.close()
def _execute(self, statement, values=None):
with self.connection:
cursor = self.connection.cursor()
cursor.execute(statement, values or [])
return cursor
def create_table(self, table_name, columns):
columns_with_types = [
f'{column_name} {data_type}'
for column_name, data_type in columns.items() #this loop reads all column information
]
self._execute(
f'''
CREATE TABLE IF NOT EXISTS {table_name}
({','.join(columns_with_types)});
'''
)
def add(self, table_name, data):
placeholders = ', '.join('?' * len(data))
column_names = ', '.join(data.keys())
column_values = tuple(data.values())
self._execute(
f'''
INSERT INTO {table_name}
({column_names})
VALUES ({placeholders});
''',
column_values,
)
def delete(self, table_name, criteria):
placeholders = [f'{column} = ?' for column in criteria.keys()]
delete_criteria = ' AND '.join(placeholders)
self._execute(
f'''
DELETE FROM {table_name}
WHERE {delete_criteria};
''',
tuple(criteria.values())
)
def select(self, table_name, criteria=None, order_by=None):
criteria = criteria or {}
query = f'SELECT * FROM {table_name}'
if criteria:
placeholders = [f'{column} = ?' for column in criteria.keys()]
select_criteria = ' AND '.join(placeholders)
query += f' WHERE {select_criteria}'
if order_by:
query += f' ORDER BY {order_by}'
return self._execute(
query,
tuple(criteria.values()),
)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
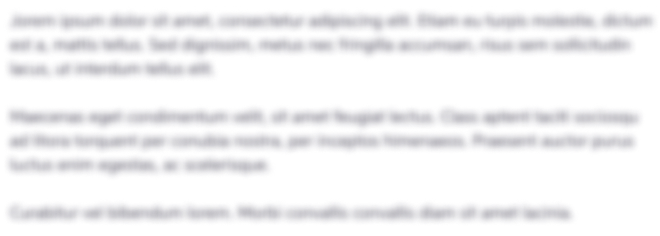
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started