Question
The code is to be written in python. Instructions are in red. The only code needed is under -------missing code--------. In this assignment, you will
The code is to be written in python. Instructions are in red. The only code needed is under -------missing code--------.
In this assignment, you will implement an online banking system. Users can sign-up with the system, log in to the system, change their password, and delete their account. They can also update their bank account balance and transfer money to another users bank account.
Youll implement functions related to File I/O and dictionaries. The first two functions require you to import files and create dictionaries. User information will be imported from the users.txt file and account information will be imported from the bank.txt file. Take a look at the content in the different files. The remaining functions require you to use or modify the two dictionaries created from the files. Each function has been defined for you, but without the code. See the docstring in each function for instructions on what the function is supposed to do and how to write the code. It should be clear enough. In some cases, we have provided hints to help you get started.
1.
CODE:
def import_and_create_dictionary(filename):
d = {}
-----------missing code--------------------
bank = import_and_create_dictionary("bank.txt")
tools.assert_false(len(bank) == 0) tools.assert_almost_equal(115.5, bank.get("Brandon")) tools.assert_almost_equal(128.87, bank.get("James")) tools.assert_is_none(bank.get("Joel")) tools.assert_is_none(bank.get("Luke")) tools.assert_almost_equal(bank.get("Sarah"), 827.43)
2
.
For example: - Calling signup(user_accounts, log_in, "Brandon", "123abcABCD") will return False - Calling signup(user_accounts, log_in, "BrandonK", "123ABCD") will return False - Calling signup(user_accounts, log_in, "BrandonK","abcdABCD") will return False - Calling signup(user_accounts, log_in, "BrandonK", "123aABCD") will return True. Then calling signup(user_accounts, log_in, "BrandonK", "123aABCD") again will return False. Hint: Think about defining and using a separate valid(password) function that checks the validity of a given password. This will also come in handy when writing the change_password() function.
CODE:
def signup(user_accounts, log_in, username, password):
--------missing code------------------------------------
3
CODE:
def import_and_create_accounts(filename):
user_accounts = {} log_in = {}
------------------missing code-------------------------
user_accounts, log_in = import_and_create_accounts("user.txt")
tools.assert_false(len(user_accounts) == 0) tools.assert_false(len(log_in) == 0) tools.assert_equal(user_accounts.get("Brandon"),"brandon123ABC") tools.assert_equal(user_accounts.get("Jack"),"jack123POU") tools.assert_is_none(user_accounts.get("Jennie")) tools.assert_false(log_in["Sarah"])
bank = import_and_create_dictionary("bank.txt") user_accounts, log_in = import_and_create_accounts("user.txt")
tools.assert_false(signup(user_accounts,log_in,"Brandon","123abcABCD"))
tools.assert_false(signup(user_accounts,log_in,"BrandonK","123ABCD")) tools.assert_false(signup(user_accounts,log_in,"BrandonK","1234ABCD")) tools.assert_false(signup(user_accounts,log_in,"BrandonK","abcdABCD")) tools.assert_false(signup(user_accounts,log_in,"BrandonK","1234abcd"))
tools.assert_false(signup(user_accounts,log_in,"123abcABCD","123abcABCD"))
tools.assert_true(signup(user_accounts,log_in,"BrandonK","123aABCD")) tools.assert_false(signup(user_accounts,log_in,"BrandonK","123aABCD")) tools.assert_true("BrandonK" in user_accounts) tools.assert_equal("123aABCD",user_accounts["BrandonK"]) tools.assert_false(log_in["BrandonK"])
4
CODE:
def login(user_accounts, log_in, username, password):
-----------------missing code---------------------------------
bank = import_and_create_dictionary("bank.txt") user_accounts, log_in = import_and_create_accounts("user.txt")
tools.assert_false(login(user_accounts, log_in,"Brandon","123abcAB")) tools.assert_true(login(user_accounts, log_in,"Brandon","brandon123ABC")) tools.assert_false(login(user_accounts, log_in,"BrandonK","123abcABC"))
MAIN:
def main(): ''' The main function is a skeleton for you to test if your overall programming is working. Note we will not test your main function. It is only for you to run and interact with your program. '''
bank = import_and_create_dictionary("bank.txt") user_accounts, log_in = import_and_create_accounts("user.txt")
while True: # for debugging print('bank:', bank) print('user_accounts:', user_accounts) print('log_in:', log_in) print('') #
option = input("What do you want to do? Please enter a numerical option below. " "1. login " "2. signup " "3. change password " "4. delete account " "5. update amount " "6. make a transfer " "7. exit ") if option == "1": username = input("Please input the username ") password = input("Please input the password ")
# add code to login login(user_accounts, log_in, username, password); elif option == "2": username = input("Please input the username ") password = input("Please input the password ")
# add code to signup signup(user_accounts, log_in, username, password) elif option == "3": username = input("Please input the username ") old_password = input("Please input the old password ") new_password = input("Please input the new password ")
# add code to change password change_password(user_accounts, log_in, username, old_password, new_password) elif option == "4": username = input("Please input the username ") password = input("Please input the password ")
# add code to delete account delete_account(user_accounts, log_in, bank, username, password) elif option == "5": username = input("Please input the username ") amount = input("Please input the amount ") try: amount = float(amount)
# add code to update amount update(bank, log_in, username, amount) except: print("The amount is invalid. Please reenter the option ")
elif option == "6": userA = input("Please input the user who will be deducted ") userB = input("Please input the user who will be added ") amount = input("Please input the amount ") try: amount = float(amount)
# add code to transfer amount transfer(bank, log_in, userA, userB, amount) except: print("The amount is invalid. Please re-enter the option. ") elif option == "7": break; else: print("The option is not valid. Please re-enter the option. ")
#This will automatically run the main function in your program #Don't change this if __name__ == '__main__': main()
This function is used to create a bank dictionary. The given argument is the filename to load. Every line in the file will look like key: value Key is a user's name and value is an amount to update the user's bank account with. The value should be a number, however, it is possible that there is no value or that the value is an invalid number. What you will do: - Try to make a dictionary from the contents of the file. - If the key doesn't exist, create a new key:value pair. If the key does exist, increment its value with the amount. You should also handle cases when the value is invalid. If so, ignore that line and don't update the dictionary. - Finally, return the dictionary. Note: All of the users in the bank file are in the user account file. This function allows users to sign up. If both username and password meet the requirements, updates the username and the corresponding password in the use and returns True. If the username and password fail to meet any one of the following requirements, returns False. - The username already exists in the user_accounts. - The password must be at least 8 characters. - The password must contain at least one lowercase character. The password must contain at least one uppercase character. The password must contain at least one number. - The username & password cannot be the same. The given argument is the f: This function is used to create an user accounts dictionary and another login dictionary. Every line in the file will look like username - password If the username and password fulfills the requirement, add the username and password into the user accounts dictionary To make sure that the password fulfills these requirements, be sure to use the signup function that you wrote above. For the login dictionary, the key is the username, and its value indicates whether the user is logged in, or not. Initially, all users are not logged in. Finally, return the dictionaries. Note: All of the users in the bank file are in the user account file. This function allows users to log in with their username and password. The users accounts stores the usernames and passwords. If the username does not exist or the password is incorrect, return False. Otherwise, return TrueStep by Step Solution
There are 3 Steps involved in it
Step: 1
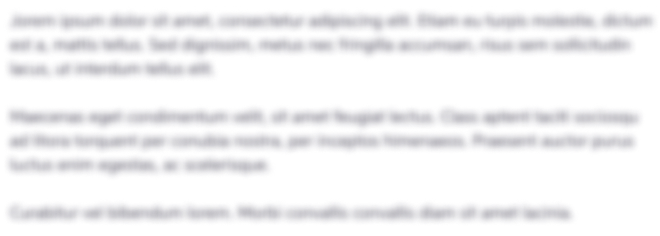
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started