Question
// the code is too long so i have posted the first half already and this would be the second half where there would be
// the code is too long so i have posted the first half already and this would be the second half where there would be 5 more todo and each are explained in the comments
/** * func: union_pareto_sorted * precondition: calling object and parameter collections must both be sorted and pareto (if not, nullptr is returned). * desc: constructs "the sorted, pareto" union (as in set-union excluding dominated options) of the two collections and returns * it as a newly created object. * RUNTIME: linear in the length of the two lists (suppose one list is of length n and the other is of length m, * then the overall runtime must be O(n+m)) * COMMENTS: REMEMBER: after this operation, the collection must be both pareto and sorted. * TIPS: Think about what must be the FIRST option in the sorted union (bootstrap) -- then think about what is the * candidate for the 2nd option (if any). * Remember: a pareto-sorted list must be strictly increasing and price and strictly decreasing in time. * * status: TODO * */ TravelOptions * union_pareto_sorted(const TravelOptions &other)const{ if(!is_pareto_sorted() || !other.is_pareto_sorted()) return nullptr;
return nullptr; } /** * func: prune_sorted * precondition: given collection must be sorted (if not, false is returned). * desc: takes sorted list of options and removes dominated entries * (and eliminates any duplicates). * RUNTIME: linear in the length of the list (O(n)) * COMMENTS: the resulting list will be sorted AND pareto. * status: TODO * */ bool prune_sorted(){ if(!is_sorted()) return false;
return true; }
/** * func: join_plus_plus * preconditions: none -- both the calling object and parameter are just TravelOptions objects (not necessarily * sorted or pareto). * param: other; a reference to another to a list of TravelOptions (thus, there are two option lists: the calling * object and the parameter). * desc: suppose you are planning a trip composed of two "legs": * * first you travel from city X to city Y (this is part of your plan - you need to stop in city Y) * then you continue from city Y to city Z * * Let the calling object be the options for the X-to-Y leg and the parameter be the options for the * Y-to-Z leg. * * Your job is to construct a pareto-sorted set of options for the entire trip and return the resulting * TravelOptions object as a pointer. * * In principle, any option
return nullptr; // placeholder to make the compiler happy
}
/** * func: join_plus_max * preconditions: both the calling object and the parameter are sorted-pareto lists (if not, nullptr is returned). * desc: imagine a different scenario (vs. join_plus_plus): you are a parent with two children -- one living in city A and * the other living in city C -- and they are both coming home for the holidays (let's call your home C). * You have a pareto-sorted option list for the A-to-C trip (this is the calling object) and a pareto-sorted option list * for the B-to-C trip. * Consider a particular option for the A-to-C trip
if(!is_pareto() || !(other.is_pareto())) return nullptr;
return nullptr; }
/** * func: sorted_clone * desc: returns a sorted TravelOptions object which contains the same elements as the current object * status: DONE [but relies on to do item insert_sorted] */ TravelOptions * sorted_clone() { TravelOptions *sorted = new TravelOptions(); Node *p = front; while(p != nullptr) { sorted->insert_sorted(p->price, p->time); p = p->next; } return sorted; }
/** * func: split_sorted_pareto * precondition: given list must be both sorted and pareto (if not, nullptr is returned; * code already given). * desc: This function takes a sorted-pareto option list and splits into two option lists: * * - the options with price less than or equal to max_price (if any) are retained in the calling * object (and only those are retained in the calling object). * - the other, more expensive options are used to populate a new TravelOptions object which * is returned as a pointer (i.e., the expensive options are returned).. * * returns: pointer to expensive options or nullptr if the calling object is not pareto-sorted. * RUNTIME: linear in the length of the given list (O(n)). * ADDITIONAL REQUIREMENT: for full credit you MAY NOT ALLOCATE ANY NEW NODES! Think about it -- * suppose your given list has 100 options and 40 of them are below the max_price threshold; * the other 60 options end up in the returnd list. Still a grand total of 100 options and * therefore 100 nodes. So... there should be no reason to delete or allocate any nodes. * status: TODO */ TravelOptions * split_sorted_pareto(double max_price) {
if(!is_pareto_sorted()) return nullptr;
return nullptr; // placeholder to make compiler happy with skeleton
}
/** * func: display * desc: prints a string representation of the current TravelOptions object * status: DONE */ void display(){ printf(" PRICE TIME "); printf("--------------------- "); Node * p = front; while(p!=nullptr) { printf(" %5.2f %5.2f ", p->price, p->time); p = p->next; } }
/** * func: checksum * desc: Performs and XOR of all node pointers and returns result as * an unsigned int. * * status: DONE * * NOTES: YOU MAY NOT TOUCH OR MODIFY THIS FUNCTION!! */ unsigned long int checksum() const { unsigned long int s = 0; Node *p = front;
while (p != nullptr) { s = s ^ ((unsigned long int)p); p = p->next; } return s; }
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
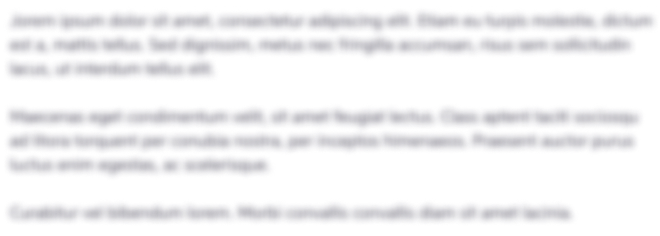
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started