Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The coding language is Python. In class, we looked at how to convert an infix expression to an equivalent postfix form. For this lab, you
The coding language is Python.
In class, we looked at how to convert an infix expression to an equivalent postfix form. For this lab, you are to modify the algorithm so that an infix expression is evaluated and the final value is returned. Pseudo-code for this was given in class. The following is required of your solution. a) Your solution must use two stacks to do this (one for values and one for operators) b) You must define a Stack class that is implemented using linked lists. Your stack class should generate exceptions as appropriate. Please call your class Stack and your methods: pop(), push(), top(), len(), and isEmpty(). c) Your program must be able to handle more general input than what you saw with the example in class. In particular, to handle more input, you must break the input expression into tokens. A token is a single element of a programming language. For example, the expression ((12 + 13)*5)" consists of the tokens (,, 12, +, 13,), *, 5.). (Each token can also be classified as to its role in the programming language, but you only need to recognize constants, parentheses, and operators.) d) Your expression evaluator must be called evalExpression() and it will accept a single string argument. You can assume that the string represents a valid infix expression and all numerical values are positive integers. Your return value should be a floating point number. e) You must also provide a function tokens() that takes a string representing a valid infix expression that prints out a listing of the tokens that makes up the expression (in the order in which they appear). For example the expression above would produce the following output:(, (, 12, +, 13,), *, 5, ) Note: There is a single space after each comma and the tokens are strings. Marking: properly implemented Stack class that uses a linked list 10 points tokens() 10 points evalExpression() - 20 points must use two stacks implemented using linked lists - deduct up to 20 points if not done comments, programming style, design 10 points Bonus: handles negative integers - 5 points, handles floats with decimal points 5 points You must use linked lists to implement the stack. Make sure all data structures and methods/functions are clearly documented. 2 Our test input will be correct; no error checking is needed. In class, we looked at how to convert an infix expression to an equivalent postfix form. For this lab, you are to modify the algorithm so that an infix expression is evaluated and the final value is returned. Pseudo-code for this was given in class. The following is required of your solution. a) Your solution must use two stacks to do this (one for values and one for operators) b) You must define a Stack class that is implemented using linked lists. Your stack class should generate exceptions as appropriate. Please call your class Stack and your methods: pop(), push(), top(), len(), and isEmpty(). c) Your program must be able to handle more general input than what you saw with the example in class. In particular, to handle more input, you must break the input expression into tokens. A token is a single element of a programming language. For example, the expression ((12 + 13)*5)" consists of the tokens (,, 12, +, 13,), *, 5.). (Each token can also be classified as to its role in the programming language, but you only need to recognize constants, parentheses, and operators.) d) Your expression evaluator must be called evalExpression() and it will accept a single string argument. You can assume that the string represents a valid infix expression and all numerical values are positive integers. Your return value should be a floating point number. e) You must also provide a function tokens() that takes a string representing a valid infix expression that prints out a listing of the tokens that makes up the expression (in the order in which they appear). For example the expression above would produce the following output:(, (, 12, +, 13,), *, 5, ) Note: There is a single space after each comma and the tokens are strings. Marking: properly implemented Stack class that uses a linked list 10 points tokens() 10 points evalExpression() - 20 points must use two stacks implemented using linked lists - deduct up to 20 points if not done comments, programming style, design 10 points Bonus: handles negative integers - 5 points, handles floats with decimal points 5 points You must use linked lists to implement the stack. Make sure all data structures and methods/functions are clearly documented. 2 Our test input will be correct; no error checking is neededStep by Step Solution
There are 3 Steps involved in it
Step: 1
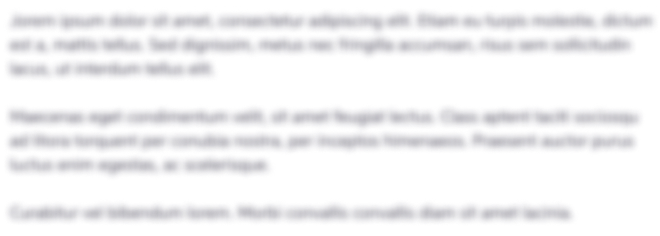
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started