Question
The ColorDisplay Class Call and write methods with parameters and return values. Use the DrawingPanel, Graphics, and Color classes. Use if statements to validate input
The ColorDisplay Class
Call and write methods with parameters and return values.
Use the DrawingPanel, Graphics, and Color classes.
Use if statements to validate input and control assignments.
Introduction
The goal of this project is to display a variety of colors in a window. Here is one example.
A drawing panel of size 550x550 will be divided into 11 rows and 11 columns, so that each color will be displayed in a 50x50 rectangle. The colors in the four corners will be based on user input. All the other colors will be constructed from combinations of these four colors.
The SimpleDisplay program illustrates the display of four predefined colors at the corners and five other colors on the sides and in the middle. Study this program. In particular, note how the program constructs the other colors by averaging the components of the predefined colors. To run this program, you need to copy DrawingPanel.java to the same folder as SimpleDisplay.java.
To construct the 11x11 color combinations in your window, your program will need to perform a more sophisticated weighted average of the four colors within a double for loop. More detail on how to code this is provided below.
Initial Zip File
You can start your project by downloading project2.zip. This file contains DrawingPanel.java, ReplaceMe.class, and this initial version of ColorDisplay.java.
import java.awt.*;
import java.util.*;
public class ColorDisplay {
public static final Scanner CONSOLE = new Scanner(System.in);
public static void main(String[] args) {
System.out.println("This project written by YOURNAME ");
Color c1 = ReplaceMe.readColor();
String s1 = ReplaceMe.colorToString(c1);
System.out.println("The first color is " + s1 + " ");
Color c2 = ReplaceMe.readColor();
String s2 = ReplaceMe.colorToString(c2);
System.out.println("The second color is " + s2 + " ");
Color c3 = ReplaceMe.readColor();
String s3 = ReplaceMe.colorToString(c3);
System.out.println("The third color is " + s3 + " ");
Color c4 = ReplaceMe.readColor();
String s4 = ReplaceMe.colorToString(c4);
System.out.println("The fourth color is " + s4 + " ");
DrawingPanel panel = new DrawingPanel(550,550);
Graphics g = panel.getGraphics();
ReplaceMe.displayColors(g, c1, c2, c3, c4);
}
}
The main method inputs four colors from the user, prints the colors, creates a DrawingPanel object, gets the Graphics object for drawing on the window, and displays the colors on the window. You should run this program with different inputs to see how it behaves.
To implement this project, you need to replace the ReplaceMe methods with your own methods. For example, each call to ReplaceMe.readColor should be replaced with readColor, which means that you must implement a readColor method in your ColorDisplay.java.
User Input
Four colors will be entered by the user. A Color object is defined by three components: red, green, and blue. The value of each component must be an int between 0 and 255. You get black if all three values are 0; you get white when all three values are 255. If the user enters invalid input, the user should get another chance to enter input. The interaction should go like this (user input is underlined).
Enter a color (three values between 0 and 255): 255 0 0
The first color is [r=255,g=0,b=0]
Enter a color (three values between 0 and 255): 0 256 0
The green value 256 is out of range
Try again to enter a color (three values between 0 and 255): 0 255 0
The second color is [r=0,g=255,b=0]
Enter a color (three values between 0 and 255): -1 0 256
The red value -1 is out of range
The blue value 256 is out of range
Try again to enter a color (three values between 0 and 255): 0 0 255
The third color is [r=0,g=0,b=255]
Enter a color (three values between 0 and 255): -1 -1 -1
The red value -1 is out of range
The green value -1 is out of range
The blue value -1 is out of range
Try again to enter a color (three values between 0 and 255): 0 0 0
The fourth color is [r=0,g=0,b=0]
If the user does not enter a valid value on the second try, then the program should exit by System.exit(1). The readColor method should have the following header:
// This method reads three color components (ints between 0 and 255)
// from the user and returns a color.
public static Color readColor()
If red, green, and blue are the three color components, then a new color can be constructed by:
Color newColor = new Color(red, green, blue);
Printing Colors
The colorToString method should have a Color parameter as input and should return a String. A simple, but not quite correct way of returning a useful String is.
return " " + color;
where color is the Color parameter. The problem with this implementation is that it includes java.awt.Color in the String.
Displaying Colors
The displayColors method has the header:
// This method displays the four colors and combinations of these colors
// in the window.
public static void displayColors (Graphics g, Color c1, Color c2, Color c3, Color c4)
As described earlier, the window is 550x550, with each color displayed on a 50x50 rectangle. This divides the window into 11 rows and 11 columns. For convenience, think of the rows going from 0 (on the top) to 10 (on the bottom), and the columns going from 0 (on the left) to 10 (on the right). The obvious solution is to code a double for loop:
Pseudocode for Displaying the Colors
For each value of row from 0 to 10:For each value of column from 0 to 10:
Construct a color based on the row and column.
Use the setColor method.
Use the fillRect method with the coordinates based on the row and column.
Constructing Colors
To construct a color for a particular row and column, all four predefined colors need to be taken into account. This can be done using a weighted average. Suppose that v1, v2, v3, and v4 are the values of one of the color components, for example:
int v1 = c1.getRed();
int v2 = c2.getRed();
int v3 = c3.getRed();
int v4 = c4.getRed();
As you might guess, there are getGreen and getBlue methods for Color objects as well.
Now you need to calculate values for the "weights": w1, w2, w3, and w4, so that the weighted average for the color component can be calculated by:
(w1 * v1 + w2 * v2 + w3 * v3 + w4 * v4) / (w1 + w2 + w3 + w4)
As long as the weights are nonnegative and at least one weight is positive, then the value is guaranteed to be between the minimum and maximum of v1, v2, v3, and v4. The values that are stored in the weights need to take the following considerations into account.
At any corner, a high value should be stored in one of the weights, and zeroes should be stored in the other weights. For example, if the row is 0 and column is 0, then a high value should be stored in w1, and zeroes should be stored in w2, w3, and w4.
For other rows and columns, the value of the weights should correspond to how close they are to their "home" corner, with higher values closer to the corner. For example, row 0 and column 0 is the home corner for w1, so w1 should have a higher value for row 2 and column 3 compared to row 8 and column 6. This is because (2,3) is closer to (0.0).
One way of taking these considerations into account is to calculate the weights by:
int w1 = (10 - row) * (10 - column); // home is (0,0)
int w2 = (10 - row) * column; // home is (0,10)
int w3 = row * (10 - column); // home is (10,0)
int w4 = row * column; // home is (10,10)
You will need to perform three weighted averages to calculate the three color components. If red, green, and blue are the three color components, then a new color can be constructed by:
Color newColor = new Color(red, green, blue);
Examples
This window was the result of the four colors: [r=255,g=0,b=0], [r=0,g=255,b=0], [r=0,g=0,b=255], and [r=0,g=0,b=0].
This window was the result of the four colors: [r=0,g=0,b=0], [r=255,g=255,b=255], [r=255,g=255,b=255], and [r=0,g=0,b=0].
--------------------------------------------------------------------------------------------------------
I have written this much of the program, I need a loops :
import java.awt.*;
import java.util.*;
public class ColorDisplay {
public static final Scanner CONSOLE = new Scanner(System.in);
public static void main(String[] args) {
System.out.println("This project written by Arash Maadani ");
Color c1 = readColor();
String s1 = colorToString(c1);
System.out.println("The first color is " + s1 + " ");
Color c2 = readColor();
String s2 = colorToString(c2);
System.out.println("The second color is " + s2 + " ");
Color c3 = readColor();
String s3 = colorToString(c3);
System.out.println("The third color is " + s3 + " ");
Color c4 = readColor();
String s4 = colorToString(c4);
System.out.println("The fourth color is " + s4 + " ");
//---------------------------------------------------------------------
DrawingPanel panel = new DrawingPanel(550,550);
Graphics g = panel.getGraphics();
//---------------------------------------------------------------------
//ReplaceMe.displayColors(g, c1, c2, c3, c4);
displayColors(g, c1, c2, c3, c4);
}
public static Color readColor(){
System.out.print("Enter a color (three values between 0 and 255):");
int r = CONSOLE.nextInt();
int g = CONSOLE.nextInt();
int b = CONSOLE.nextInt();
Color mynew = new Color(r,g,b);
return mynew;
}
public static String colorToString(Color c){
int r = c.getRed();
int g = c.getGreen();
int b = c.getBlue();
String myString = "The second color is [r="+r+",g="+g+",b="+b+"]";
return myString;
}
public static void displayColors (Graphics g, Color c1, Color c2, Color c3, Color c4){
g.setColor(c1);
g.fillRect(0,0,50,50);
g.setColor(c2);
g.fillRect(500,0,50,50);
g.setColor(c3);
g.fillRect(0,500,50,50);
g.setColor(c4);
g.fillRect(500,500,50,50)
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
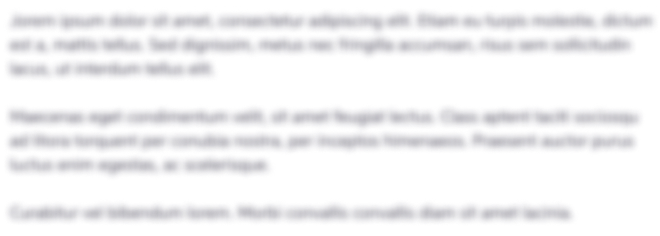
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started