Question
The current site uses a hard-coded page in index.html to display a page containing 8 twits. Your next task in the assignment is to implement
The current site uses a hard-coded page in index.html to display a page containing 8 twits. Your next task in the assignment is to implement a templatized version of this twits page, and to use data stored on the server side to dynamically generate the twits page when a client requests it. Specifically, you are provided with raw data in twitData.json representing the current set of 8 twits. You should use that data in conjunction with a set of templates you write to replace the functionality index.html. Here are some specific things you'll have to do to make this happen:
Implement one or more .handlebars template files to replicate the structure of index.html.
Your new set of templates can use a layout template if you'd like. This isn't strictly necessary here, but you'll have to do it eventually to earn full credit for the assignment.
In these new templates, instead of hard-coding the twits to be displayed, use the twit template you created in step 1 as a partial to render each twit in an array of twits that's passed as a template argument.
In your server process in server.js, set up your Express server to use express-handlebars as the view engine. Note that you'll need to install express-handlebars as a dependency of your package.
Implement a route in your server process for the root path /. Make sure this route's middleware is called before the middleware function that serves index.html. Within this new route, you should respond to the client by using your newly-created template(s) to render the twits page (which should look the same as index.html). In particular, make sure you load the raw twit data from twitData.json and pass all of this twit data into your template(s) using the appropriate template argument(s). When you render the twits page this way, make sure to respond with status 200.
//--------------------------index.html---------------------------------//
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
tweeter | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Sitting in web dev... This class is so awesome! | |
| |
| |
CSMajor2018 | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Anyone watch the baseball game last night? The Beavs are still in 1st place! | |
| |
| |
BeaverBeliever | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
A body in motion must remain in motion unless acted upon by an outside force. | |
| |
| |
NewtonRulez | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Huh? | |
| |
| |
ConfusedTweeterer | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Why did the calf cross the road? | |
| |
| |
Setup | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
To get to the udder side! | |
| |
| |
Punchline | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Any questions about flexboxes? | |
| |
| |
Hess | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Friendly reminder: your taxes were due yesterday. | |
| |
| |
TheIRS | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
Create a Twit | |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
| |
//------------------------------style.css--------------------------//
font-family: Roboto, Helvetica, sans-serif; | |
margin: 0; | |
background-color: #eee; | |
} | |
| |
a { | |
text-decoration: none; | |
} | |
| |
.site-title { | |
margin: 0; | |
padding: 10px 20px; | |
font-size: 30px; | |
font-weight: 100; | |
background-color: #319df6; | |
color: #fff; | |
} | |
| |
.navbar { | |
background-color: #fff; | |
font-size: 18px; | |
line-height: 22px; | |
} | |
| |
.navlist { | |
margin: 0 0 0 20px; | |
padding: 0; | |
} | |
| |
.navitem { | |
display: inline-block; | |
} | |
| |
a { | |
color: #343434; | |
} | |
| |
a:hover, | |
a:focus { | |
color: #319df6; | |
} | |
| |
a:focus { | |
outline: none; | |
} | |
| |
.navlink a { | |
padding: 8px 10px; | |
display: inline-block; | |
} | |
| |
.navlink.active { | |
box-shadow: inset 0 -3px #319df6; | |
} | |
| |
.navbar-search { | |
float: right; | |
margin-right: 20px; | |
padding: 0; | |
line-height: 38px; | |
} | |
| |
#navbar-search-input { | |
padding: 4px; | |
font-size: 16px; | |
background-color: #eee; | |
border: 1px solid #dadada; | |
border-radius: 2px; | |
} | |
| |
#navbar-search-input:focus { | |
outline: none; | |
background-color: #fff; | |
} | |
| |
#navbar-search-button { | |
margin-left: -5px; | |
font-size: 16px; | |
background: none; | |
border: none; | |
color: #343434; | |
cursor: pointer; | |
} | |
| |
#navbar-search-button:hover { | |
color: #319df6; | |
} | |
| |
#navbar-search-button:focus { | |
color: #319df6; | |
outline: none; | |
} | |
| |
.twit-container { | |
margin: 50px 10%; | |
display: flex; | |
flex-wrap: wrap; | |
} | |
| |
.twit { | |
flex: 0 1 28%; | |
display: flex; | |
margin: 2px; | |
padding: 20px; | |
border: 1px solid #dadada; | |
border-radius: 2px; | |
background-color: #fff; | |
} | |
| |
.twit-icon { | |
font-size: 24px; | |
} | |
| |
.twit-content { | |
flex: 1 1 80%; | |
margin-left: 10px; | |
} | |
| |
.twit-content p { | |
margin: 0; | |
} | |
| |
.twit-content .twit-attribution { | |
margin-top: 8px; | |
text-align: right; | |
font-weight: bold; | |
} | |
| |
#create-twit-button { | |
position: fixed; | |
right: 30px; | |
bottom: 30px; | |
height: 60px; | |
width: 60px; | |
border: none; | |
border-radius: 30px; | |
font-size: 30px; | |
background-color: #ff1010; | |
color: #fff; | |
cursor: pointer; | |
box-shadow: 4px 4px 12px rgba(0, 0, 0, 0.25); | |
} | |
| |
#create-twit-button:hover, | |
#create-twit-button:focus { | |
right: 27px; | |
bottom: 27px; | |
height: 66px; | |
width: 66px; | |
border-radius: 33px; | |
font-size: 36px; | |
background-color: #319df6; | |
} | |
| |
#create-twit-button:focus { | |
outline: none; | |
} | |
| |
#modal-backdrop { | |
position: fixed; | |
top: 0; | |
bottom: 0; | |
left: 0; | |
right: 0; | |
z-index: 1000; | |
background-color: rgba(0, 0, 0, 0.85); | |
} | |
| |
#create-twit-modal { | |
position: fixed; | |
top: 0; | |
bottom: 0; | |
left: 0; | |
right: 0; | |
z-index: 1010; | |
overflow: scroll; | |
} | |
| |
.hidden { | |
display: none; | |
} | |
| |
.modal-dialog { | |
width: auto; | |
max-width: 600px; | |
min-width: 350px; | |
min-height: 300px; | |
margin: 40px auto; | |
background-color: #fff; | |
border-radius: 15px; | |
} | |
| |
.modal-header { | |
position: relative; | |
padding: 10px 20px; | |
} | |
| |
.modal-header h3 { | |
margin: 0; | |
font-size: 28px; | |
} | |
| |
.modal-close-button { | |
position: absolute; | |
right: 10px; | |
top: 5px; | |
font-size: 24px; | |
border: none; | |
background: none; | |
padding: 0; | |
cursor: pointer; | |
} | |
| |
.modal-body { | |
padding: 20px; | |
} | |
| |
.twit-input-element { | |
display: flex; | |
align-items: center; | |
font-size: 20px; | |
margin: 10px 0; | |
} | |
| |
.twit-input-element label { | |
font-weight: 600; | |
flex: 1 75px; | |
margin-right: 10px; | |
} | |
| |
.twit-input-element input, | |
.twit-input-element textarea { | |
flex: 6 200px; | |
padding: 8px; | |
font-size: 20px; | |
border: 1px solid #aaa; | |
} | |
| |
.twit-input-element textarea { | |
min-height: 64px; | |
} | |
| |
.modal-footer { | |
display: flex; | |
justify-content: flex-end; | |
padding: 10px 10px 20px 10px; | |
} | |
| |
.modal-footer button { | |
font-size: 20px; | |
background-color: #fff; | |
color: #333; | |
border: 1px solid #333; | |
border-radius: 5px; | |
padding: 10px; | |
margin-right: 20px; | |
cursor: pointer; | |
} | |
| |
.modal-footer .modal-cancel-button:hover { | |
background-color: #eee; | |
} | |
| |
.modal-footer .modal-accept-button { | |
background-color: #319df6; | |
color: #fff; | |
} | |
| |
.modal-footer .modal-accept-button:hover { | |
background-color: #087dde; | |
} | |
| |
.error-container { | |
margin-top: 50px; | |
text-align: center; | |
} | |
| |
.error-container h2 { | |
margin: 10px 0; | |
font-size: 96px; | |
font-weight: 100; | |
} | |
| |
.error-container h3 { | |
margin: 10px 0; | |
font-size: 36px; | |
font-weight: 400; | |
} |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
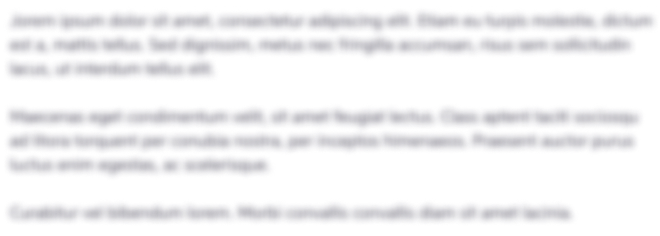
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started