Question
***************The data file is flags.csv****************** For project 2 we are changing our focus to loops and functions. We will of course need to use selection
***************The data file is flags.csv******************
For project 2 we are changing our focus to loops and functions. We will of course need to use selection statements and as always order or our statements is important.
Details
In this project we will be writing a function that will accept 2 parameters. This function will be our primary function. This will be the function that Web-CAT calls. The two parameters will be strings and the strings will contain the names of files. The first file will be used to read in commands. The second file will be used to output our results. Most of our assignments to Web-CAT will follow a similar pattern.
The input file will have a series of commands. There might be 1 command or there might be many. To handle this we will use the input controlled failure loop (prime, test, process, re-prime). Ill describe the commands below.
The commands will be asking you to process a data file that contains information about flags. This data is in comma separated variable format, or CSV. I have some videos in the Helpful Videos on how to read in CSV data that I highly encourage you to watch. The first one is long ~45 minutes and the second one is shorter and builds from the first one.
Our data has 30 fields. Yes 30. I know its a lot, but I think the data here will help emphasize the use of the functions. Ill describe the data down below as well.
Make sure you have read the details about the commands and data and understand what Im asking before you start writing code.
Commands
There are 3 commands we are going to be handling.
colors This command will be followed by 1 or more strings that could be possible colors in the flags. You need to read this string and then determine which flags, if any, have that exact combination of colors. For example, if red and white were the colors you would only find flags with red and white, not flags with red, white, and other colors too. Ill explain how I would approach this below. If you find matches you will output information about that flag.
name This will be followed by a word or part of a word. The word may or may not be found in the name of the country or countries in the file. You will use the find method on the name to search within the name for the given string. In the videos on reading data I describe the find method. If you find matches you will output information about that flag.
landmass This will be followed by the name of a potential landmass that is found in the file. You will do an exact match on the landmass from the file. If you find the landmass you will display information about the flag.
For all of the commands you will also first output the command and the string you are using to do the search. Then you will output any results you find. If you dont find any results then there is a message you will output in that case.
Ill provide samples of the output below and in the sample zip file.
Sample
Here is a sample input file.
colors red white name US name ur landmass Asia colors red purple name Not here! landmass Atlantis
You can see that the string after the name might contain spaces. I recommend using getline to read in that string. Dont forget there is a space before that string, so you have to ignore that space before you use getline.
Output
As stated above, each command will have some echo output, meaning each command will let you know what it is and what arguments it was given. This is a debugging technique and also good programming style. It can help you figure out how many commands your program is processing and imagine this was a program you were using, it would let you know what you entered and if you made a mistake you could see that.
So, each output will start with the word Command:. After that you need to output the command and any and all arguments for the command. Following that line there could be a lot of output. For each matching flag you will first output the word Flag: followed by which flag # it is in the file. For example the first flag is flag 1 and the last flag is 194.
Then on the next four lines you will output the following:
Line 1: Name Landmass Zone Area Population Language Religion Line 2: # of Bars # of Stripes # of Colors List of it's colors Main hue Line 3: Shapes Line 4: Top left color Bottom right color
Any part of this output might be left out. For example if the flag has no bars, then in that part of the output there wont be anything. See the samples to make sure you understand what I want.
I recommend writing a function to do this output.
Below is part of the output for the given sample input. Ill attach the complete output as a file. My file has 422 lines.
Command: colors red white Flag: 12 Austria Europe NE 84 1000KM^2 8Mil German Catholic Bars: 0 Stripes: 3 Colors: 2 Red White Main hue: red Shapes: Top left: red Bottom Right: red Flag: 14 Bahrain Asia NE 1 1000KM^2 0Mil Arabic Muslim Bars: 0 Stripes: 0 Colors: 2 Red White Main hue: red Shapes: Top left: white Bottom Right: red --snip-- Flag: 176 Turkey Asia NE 781 1000KM^2 45Mil Japanese/Turkish/Finnish/Magyar Muslim Bars: 0 Stripes: 0 Colors: 2 Red White Main hue: red Shapes: Sunstars Crescent Top left: red Bottom Right: red Total flags found: 15 Command: name US Flag: 183 US-Virgin-Isles N.America NW 0 1000KM^2 0Mil English Other Christian Bars: 0 Stripes: 0 Colors: 6 Red Green Blue Yellow White Main hue: white Shapes: Icon Animate Text Top left: white Bottom Right: white Flag: 184 USA N.America NW 9363 1000KM^2 231Mil English Other Christian Bars: 0 Stripes: 13 Colors: 3 Red Blue White Main hue: white Shapes: Quarters Sunstars Top left: blue Bottom Right: red Flag: 185 USSR Asia NE 22402 1000KM^2 274Mil Slavic Marxist Bars: 0 Stripes: 0 Colors: 2 Red Yellow Main hue: red Shapes: Sunstars Icon Top left: red Bottom Right: red Total flags found: 3 Command: name ur Flag: 28 Burkina Africa NW 274 1000KM^2 7Mil French Ethnic Bars: 0 Stripes: 2 Colors: 3 Red Green Yellow Main hue: red Shapes: Sunstars Top left: red Bottom Right: green --snip-- Total flags found: 11 Command: landmass Asia Flag: 1 Afghanistan Asia NE 648 1000KM^2 16Mil Other Muslim Bars: 0 Stripes: 3 Colors: 5 Red Green Yellow White Black Main hue: green Shapes: Sunstars Icon Top left: black Bottom Right: green Flag: 14 Bahrain Asia NE 1 1000KM^2 0Mil Arabic Muslim Bars: 0 Stripes: 0 Colors: 2 Red White Main hue: red Shapes: Top left: white Bottom Right: red --snip-- Flag: 189 Vietnam Asia NE 333 1000KM^2 60Mil Other Marxist Bars: 0 Stripes: 0 Colors: 2 Red Yellow Main hue: red Shapes: Sunstars Top left: red Bottom Right: red Total flags found: 39 Command: colors red purple Sorry no flags with just red purple found. Command: name Not here! Sorry no flags with part of its name Not here! found. Command: landmass Atlantis Sorry no flags in area Atlantis found.
Above where I have snip that indicates Ive cut out parts of the output. See the attached file for the complete output.
The blank lines in the output are not necessary, Ive added them because I like them. Web-CAT will not care if you have them or not. It does not count blank lines so in the hints, if there are blank lines in your output those will not be part of the line count. Ill see if I can fix that.
Functions
Since this project is about functions youll need to write some. Each of the 3 commands must be implemented as a function. The videos on Reading CSV Data in the Helpful Videos goes over some ideas on how to handle that.
I highly recommend you write a function that reads in 1 single line of the data and returns the data as pass-by-reference parameters. Later in the term well learn a better way to do this. Since there are 30 data fields, that function will have 31 parameters, the ifstream and the fields.
I also highly recommend you write a function to output the data. Ill describe the data below this, but youll see that it contains numbers to represent the landmass, region, language, religion, etc. So this function can handle the conversion (using selection statements) from a number to what should be output.
Finally, there are lots of other places where you can (and should) write functions. One final function I highly recommend is a function that will take the string containing the colors for the color command and return what is known as a mask. A mask is simply a way to encode data.
There are 7 possible colors in the flags. So for example the colors red and blue could be represented as 11. Where one of the 1s means red and the other means blue. Ive used the following constants to build my mask:
const int mask_end = 10000000; const int red_mask = 1000000; const int green_mask = 100000; const int blue_mask = 10000; const int gold_mask = 1000; const int white_mask = 100; const int black_mask = 10; const int orange_mask = 1;
So if I have the colors green and orange in my flag, I add together the mask_end along with the masks for green and orange. This produces the int 10100001. In this way, I can figure out what colors are present in each flag and then compare the flag to the colors I want. If the masks match, then the flag is a match. So I wrote a function that converts the colors into this mask. This might be strange so Ill probably make a video that explains what Im talking about.
Data
Heres the first 5 lines from the data file:
Afghanistan,5,1,648,16,10,2,0,3,5,1,1,0,1,1,1,0,green,0,0,0,0,1,0,0,1,0,0,black,green Albania,3,1,29,3,6,6,0,0,3,1,0,0,1,0,1,0,red,0,0,0,0,1,0,0,0,1,0,red,red Algeria,4,1,2388,20,8,2,2,0,3,1,1,0,0,1,0,0,green,0,0,0,0,1,1,0,0,0,0,green,white American-Samoa,6,3,0,0,1,1,0,0,5,1,0,1,1,1,0,1,blue,0,0,0,0,0,0,1,1,1,0,blue,red Andorra,3,1,0,0,6,0,3,0,3,1,0,1,1,0,0,0,gold,0,0,0,0,0,0,0,0,0,0,blue,red
You can probably tell from just this small snippet that this data is not human friendly, meaning its very hard for us to determine what it means. Heres what each of the fields in the record represent.
name Name of the country concerned
landmass 1=N.America, 2=S.America, 3=Europe, 4=Africa, 5=Asia, 6=Oceania
zone Geographic quadrant, based on Greenwich and the Equator 1=NE, 2=SE, 3=SW, 4=NW
area in thousands of square km
population in round millions
language 1=English, 2=Spanish, 3=French, 4=German, 5=Slavic, 6=Other Indo-European, 7=Chinese, 8=Arabic, 9=Japanese/Turkish/Finnish/Magyar, 10=Other
religion 0=Catholic, 1=Other Christian, 2=Muslim, 3=Buddhist, 4=Hindu, 5=Ethnic, 6=Marxist, 7=Other
bars Number of vertical bars in the flag
stripes Number of horizontal stripes in the flag
colours Number of different colours in the flag
red 0 if red absent, 1 if red present in the flag
green same for green
blue same for blue
gold same for gold (also yellow)
white same for white
black same for black
orange same for orange (also brown)
mainhue predominant colour in the flag (tie-breaks decided by taking the topmost hue, if that fails then the most central hue, and if that fails the leftmost hue)
circles Number of circles in the flag
crosses Number of (upright) crosses
saltires Number of diagonal crosses
quarters Number of quartered sections
sunstars Number of sun or star symbols
crescent 1 if a crescent moon symbol present, else 0
triangle 1 if any triangles present, 0 otherwise
icon 1 if an inanimate image present (e.g., a boat), otherwise 0
animate 1 if an animate image (e.g., an eagle, a tree, a human hand) present, 0 otherwise
text 1 if any letters or writing on the flag (e.g., a motto or slogan), 0 otherwise
topleft colour in the top-left corner (moving right to decide tie-breaks)
botright Colour in the bottom-left corner (moving left to decide tie-breaks)
I found this data online and it is real data. It is also dated, i.e. its from 1986. So I apologize if you country is not present or if the information is no longer current. I picked the data because its messy and represents the kind of data you could encounter on a job or internship.
So in the data you can see for example that a 1 in the second field represents North America. When you output your flag information you need to use these numbers to convert the number into the corresponding string for output. I recommend using a selection statement. An if works or this is also an opportunity for the switch statement if you want to try that one. Either is fine just talking design here.
Design
Speaking of design, you probably (hopefully) realize this is a larger project than the last one. That is by design. I want you to recognize that you have to work a little at a time.
My first recommendation is to write a program that will read each flag and output it they way I want it. Once you have that, you really have a good chunk done. This will form the basis of the individual command processing functions. Also, until you can read each flag you are really stuck. So work on this first. The data is hard to read, so make sure you get each 1 of the 30 fields. My output function is long, over 100 lines of code. Take your time with these and get them right. Once you have those, then you can start tackling the rest of the project.
Requirements
For full credit make sure you follow the requirements. We will use these requirements for the remaining 25 points.
You must declare your function void flags( string input, string output ); in a file named flag.h
You may not use global variables. This is a big one to me. Learn how to correctly pass parameters to functions and when to use pass-by-reference and when to use pass-by-copy.
You may not use arrays, structs, classes, pointers.
You must write a function to each of the commands.
It is highly recommend to write functions to read in a flag, output a flag, create the mask for the colors.
The name of the flag data file is: flags.csv This may be hardcoded into your program if you wish.
You may not store more than a single flags information in memory at a time.
You may not use temporary files to store flag data.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
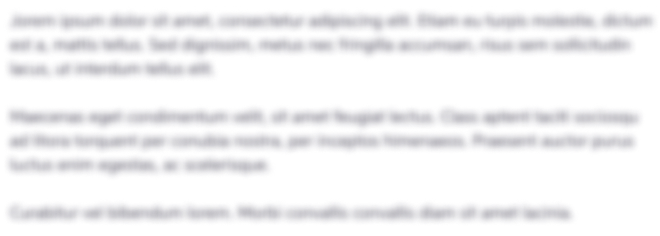
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started