Question
The DirectedGraph Class To this class, you will need to add two public methods and a public indexer (Links to an external site.)Links to an
The DirectedGraph Class
To this class, you will need to add two public methods and a public indexer (Links to an external site.)Links to an external site.. You will also need to modify the AddEdge and GetTuple methods. These changes (specifically the addition of the indexer) will allow edge data to be modified; however, there will still be no way to remove edges. Instead, because all capacities and flows on edges will be positive, you will be able to denote a "removed" edge by setting its data to 0. Requirements for the methods and the indexer are described in what follows.
The GetTuple method
The message to be displayed when the source and destination nodes of an edge are the same (see under "Exception Handling" above) needs to be included in the exception that is thrown in this case.
The AddEdge method
The message to be displayed when some edge occurs more than once should (see under "Exception Handling" above) needs to be included in the exception that is thrown in this case.
A method to add a node
This method should take as its only parameter the node to be added, and it should return nothing. To add the node, it can add it as a key to the _adjacencyLists dictionary, with null as its associated value.
A method to obtain the data item associated with an edge
This method should take the following parameters:
the source node of an edge;
the destination node of an edge; and
an out parameter for the data item associated with the edge from the source to the destination.
It should return a bool indicating whether there is an edge from the source to the destination. If so, the out parameter should be set to the data item associated with that edge; otherwise, the out parameter should be set to the default value for this type. You can accomplish all of this by using the TryGetValue method of the _edges dictionary.
An indexer
This indexer will allow the user to get or modify the data item associated with an edge by using its source and destination nodes as indices. For example, if graph refers to a DirectedGraph and source and dest refer to TNodes, then the user can access the data item associated with the edge from source to dest via the expression graph[source, dest]. The structure of an indexer is described in the section, "Indexers" (Links to an external site.)Links to an external site..
The type of this indexer should be TEdgeData. It will need as its parameters the source and destination nodes. It will need both a get accessor and a set accessor. The get accessor should return the data item associated with the edge from the source to the destination, or throw a KeyNotFoundException if there is no such edge. You can accomplish this by indexing into the _edges dictionary with a Tuple formed from the source and destination nodes. The set accessor should set the data item associated with this edge to the value given via the value keyword. If this edge does not exist, it should be added, along with the given source and/or destination nodes as necessary. If either the source or destination is null, it should throw an ArgumentNullException. If the two nodes are equal, it should throw an ArgumentException. You can pattern your code after the AddEdge method, but instead of throwing an exception if the edge already exists, assign the given value to the edge in the _edges dictionary (the _adjacencyLists dictionary won't change in this case).
The given Code is
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Ksu.Cis300.LinkedListLibrary;
namespace Ksu.Cis300.Graphs { public class DirectedGraph
private Dictionary
public IEnumerable
private static Tuple
private void AddNewEdge(Tuple
public void AddEdge(TNode source, TNode dest, TEdgeData value) { Tuple
public IEnumerable
public class AdjacencyListEnumerator : IEnumerator
private LinkedListCell
private DirectedGraph
private TNode _source;
public AdjacencyListEnumerator(DirectedGraph
public Edge
public void Dispose() {
}
object System.Collections.IEnumerator.Current { get { return Current; } }
public bool MoveNext() { if (_current == null) { return false; } _current = _current.Next; return _current != null; }
public void Reset() { throw new NotImplementedException(); } }
public class AdjacencyList : IEnumerable
private TNode _source;
public AdjacencyList(DirectedGraph
public IEnumerator
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return GetEnumerator(); } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
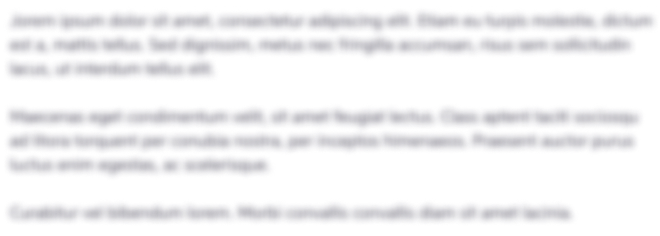
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started