Question
The DVD Manager Application Java Files DVD.java - A class to model a single DVD, including its title, rating and total running time. DVDCollection.java -
The DVD Manager Application
Java Files
- DVD.java - A class to model a single DVD, including its title, rating and total running time.
- DVDCollection.java - A class to model a collection of DVDs using an array.
- DVDUserInterface.java - An interface that describes the operation required for any user interface to this DVD collection.
- DVDGUI.java - A class that implements the DVDUserInterface interface and provides a graphical user interface to the DVD collection.
- DVDConsoleUI.java - A class that implements the DVDUserInterface interface and provides a console user interface to the DVD collection.
- DVDManager.java - A class that contains a main method that launches one of the two user interfaces for the user to work with the DVD collection based on the user input.
*Your assignment is to complete the first two classes (DVD and DVDCollection) to create a correctly functioning application.
*Complete the DVD class by completing the given methods (constructor, accessors and mutators).
The DVDCollection class uses an array to maintain the collection of DVDs. The DVDs should be stored in alphabetical order based on title starting at position 0 in the array, using adjacent cells. All titles should be stored in uppercase only and are assumed to be unique (no duplicates). You may assume titles do not contain commas. Complete the following methods in the DVDCollection class in the order shown below and test each one before moving on.
- toString - returns a string containing all of the DVDs in the collection, separated by newlines characters, along with the value of numdvds and the length of the DVD array for debugging purposes. The string should be formatted as shown in the example below:
numdvds = 3
dvdArray.length = 7
dvdArray[0] = ANGELS AND DEMONS/PG-13/138min
dvdArray[1] = STAR TREK/R/127min
dvdArray[2] = UP/PG/96min
- addOrModifyDVD - given the title, rating and running time of a DVD, add this DVD to the collection if the title is not present in the DVD collection or modify the DVD's rating and running time if the title is present in the collection. Do this operation only if the rating and running time are valid. If a new DVD is added to this collection, insert the DVD so that all DVDs are in alphabetical order by title. (NOTE: The collection should already be in alphabetical order when this method is called since this is the only method available to insert DVDs.)
- removeDVD - given the title, this method should remove the DVD with this title from the collection if present. The title must match exactly (in uppercase). If no title matches, do not change the collection.
- getDVDsByRating - given the rating, this method should return a string containing all DVDs that match the given rating in the order that they appear in the collection, separated by newlines.
- getTotalRunningTime - this method should return the total running time of all DVDs in the collection. If there are no DVDs in the collection, return 0.
- loadData - given a file name, this method should try to open this file and read the DVD data contained inside to create an initial alphabetized DVD collection. HINT: Read each set of three values (title, rating, running time) and use the addOrModifyDVD method above to insert it into the collection. If the file cannot be found, start with an empty array for your collection. If the data in the file is corrupted, stop initializing the collection at the point of corruption.
- save - save the DVDs currently in the array into the same file specified during the load operation, overwriting whatever data was originally there.
**Do not try to write all of these methods before testing your program. Instead, write the first two methods (and any associated helper methods) and test your program. Once you feel your program is tested enough, then move on to the other methods, one by one.
**Do the file input/output methods last. This way, if they dont work correctly, you can always go back to the previous version and hand in something that works correctly without using files, rather than hand in something that does not work at all.
Additional Requirements:
**You may not change any code given to you already. That is, do not alter the methods so that your methods will work. You should be able to complete the assignment starting from the code given to you.
DVD.java
public class DVD {
// Fields:
private String title; // Title of this DVD private String rating; // Rating of this DVD private int runningTime; // Running time of this DVD in minutes
public DVD(String dvdTitle, String dvdRating, int dvdRunningTime) { } public String getTitle() {
return null; // STUB: Remove this line. } public String getRating() {
return null; // STUB: Remove this line. } public int getRunningTime() {
return 0; // STUB: Remove this line. }
public void setTitle(String newTitle) {
}
public void setRating(String newRating) {
}
public void setRunningTime(int newRunningTime) {
}
public String toString() {
return null; // STUB: Remove this line. } }
DVDCollection.java
import java.io.*;
public class DVDCollection {
// Data fields /** The current number of DVDs in the array */ private int numdvds; /** The array to contain the DVDs */ private DVD[] dvdArray; /** The name of the data file that contains dvd data */ private String sourceName; /** Boolean flag to indicate whether the DVD collection was modified since it was last saved. */ private boolean modified; /** * Constructs an empty directory as an array * with an initial capacity of 7. When we try to * insert into a full array, we will double the size of * the array first. */ public DVDCollection() { numdvds = 0; dvdArray = new DVD[7]; } public String toString() { // Return a string containing all the DVDs in the // order they are stored in the array along with // the values for numdvds and the length of the array. // See homework instructions for proper format.
return null; // STUB: Remove this line. }
public void addOrModifyDVD(String title, String rating, String runningTime) { // NOTE: Be careful. Running time is a string here // since the user might enter non-digits when prompted. // If the array is full and a new DVD needs to be added, // double the size of the array first. } public void removeDVD(String title) {
} public String getDVDsByRating(String rating) {
return null; // STUB: Remove this line.
}
public int getTotalRunningTime() {
return 0; // STUB: Remove this line.
} public void loadData(String filename) { } public void save() {
}
// Additional private helper methods go here }
DVDConsoleUI:
DVDGUI:
DVDManager:
DVDUserInterface:
**dvddata**:
Please answer in Java language and test all function works. Thank you.
import java. util. *; * This class is an implementation of DVDUser Interface * that uses the console to display the menu of command choices public class DVD ConsoleUI implements DVDUser Interface { private DVDCollection dvdlist; private Scanner scan; public DVD ConsoleUI (DVDCollection dl) { dvdlist = dl; scan = new Scanner(System.in); public void process Commands String[] commands = ["Add/Modify DVD", "Remove DVD", "Get DVDs By Rating", "Get Total Running Time", "Save and Exit" }; int choice; do for (int i = 0; iStep by Step Solution
There are 3 Steps involved in it
Step: 1
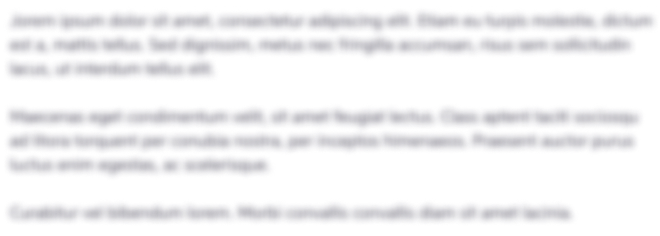
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started