Answered step by step
Verified Expert Solution
Question
1 Approved Answer
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers. Here's how a Fibonacci series function
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers. Here's how a Fibonacci series function works: Functionality: The function typically takes an integer n as input, representing the number of elements desired in the Fibonacci sequence. Steps: Base Cases: The function usually defines two base cases to handle the starting values of the sequence, which are typically and Recursive or Iterative approach: The function implements either a recursive or iterative approach to calculate the remaining Fibonacci numbers. Recursive approach: Defines a function that calls itself with n and n as input to calculate the previous two numbers and then returns their sum. This approach can be less efficient for larger values of n due to repeated function calls. Iterative approach: Uses a loop to iterate through the desired number of elements, keeping track of the previous two numbers and calculating the next one based on their sum. This approach is generally more efficient for larger sequences. Return the Sequence: The function returns the complete Fibonacci sequence up to the nth element often as a list or array or just the nth Fibonacci number here's the Fibonacci sequence function in pseudocode: function fibonaccin Base cases if n return n Initialize variables to store the previous two numbers prev : prev : Loop to calculate remaining Fibonacci numbers for i from to n current : prev prev prev : prev prev : current Return the nth Fibonacci number return current end function Implement fibonacci in at least two programming languages one interpreted the other compiled. run each implementation using different inputs n Report the time needed for each run. use graphs and tables Compare the performance. Discuss and conclude the timing results. The deliverables : Code: Two correctly implemented versions of the fibonaccin function: One in an interpreted language eg Python One in a compiled language eg C The code should be wellformatted, indented, and include comments explaining the logic. Analysis and Results: A table or list showing the chosen input values n for running the Fibonacci functions. Corresponding output resulting Fibonacci numbers for each input value. A table or chart displaying the execution time required for each run interpreted vs compiled languages using different input values. Report: A discussion comparing the performance of the interpreted and compiled languages based on the execution time results. An explanation for the observed performance variations. A conclusion summarizing the key takeaways from the assignment, including the impact of interpreted vs compiled languages and any optimizations explored. Optional Bonus Deliverables: Code demonstrating optimizations made to improve the performance of the function eg memoization in a recursive approach A graph or chart visualizing the execution time data for different input values. A discussion of potential future improvements or further exploration related to the Fibonacci sequence function or performance analysis. Overall Presentation: The assignment should be wellwritten, organized, and use proper grammar and formatting. Assignment evaluation methodology for TA aattention Implementation Correctness points: The provided Fibonacci function fibonaccin must be correctly implemented in at least two programming languages: one interpreted eg Python and one compiled eg C Each implementation points each should accurately calculate the Fibonacci sequence for different input values. Code Clarity points: The code should be wellformatted, indented, and include comments explaining the logic behind each step variable names, loops, calculations Efficiency Considerations points: Bonus If applicable to the chosen languages, discuss any optimizations made to improve the performance of the function, especially for larger input values eg using memoization in a recursive approach Analysis and Results Running the Implementations points: The assignment should demonstrate running each implementation fibonaccin with different input values n Include a table or list showing the chosen input values and the corresponding output resulting Fibonacci numbers Performance Measurement points: The assignment should report the execution time required for each run interpreted vs compiled languages using different input values. This can be achieved using builtin functions in the languages to measure execution time. Data Visualization points: Bonus Present the execution time data for different input v
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers. Here's how a Fibonacci series function works:
Functionality:
The function typically takes an integer n as input, representing the number of elements desired in the Fibonacci sequence.
Steps:
Base Cases: The function usually defines two base cases to handle the starting values of the sequence, which are typically and
Recursive or Iterative approach: The function implements either a recursive or iterative approach to calculate the remaining Fibonacci numbers.
Recursive approach: Defines a function that calls itself with n and n as input to calculate the previous two numbers and then returns their sum. This approach can be less efficient for larger values of n due to repeated function calls.
Iterative approach: Uses a loop to iterate through the desired number of elements, keeping track of the previous two numbers and calculating the next one based on their sum. This approach is generally more efficient for larger sequences.
Return the Sequence: The function returns the complete Fibonacci sequence up to the nth element often as a list or array or just the nth Fibonacci number
here's the Fibonacci sequence function in pseudocode:
function fibonaccin
Base cases
if n
return n
Initialize variables to store the previous two numbers
prev :
prev :
Loop to calculate remaining Fibonacci numbers
for i from to n
current : prev prev
prev : prev
prev : current
Return the nth Fibonacci number
return current
end function
Implement fibonacci in at least two programming languages one interpreted the other compiled.
run each implementation using different inputs n
Report the time needed for each run. use graphs and tables
Compare the performance.
Discuss and conclude the timing results.
The deliverables :
Code:
Two correctly implemented versions of the fibonaccin function:
One in an interpreted language eg Python
One in a compiled language eg C
The code should be wellformatted, indented, and include comments explaining the logic.
Analysis and Results:
A table or list showing the chosen input values n for running the Fibonacci functions.
Corresponding output resulting Fibonacci numbers for each input value.
A table or chart displaying the execution time required for each run interpreted vs compiled languages using different input values.
Report:
A discussion comparing the performance of the interpreted and compiled languages based on the execution time results.
An explanation for the observed performance variations.
A conclusion summarizing the key takeaways from the assignment, including the impact of interpreted vs compiled languages and any optimizations explored.
Optional Bonus Deliverables:
Code demonstrating optimizations made to improve the performance of the function eg memoization in a recursive approach
A graph or chart visualizing the execution time data for different input values.
A discussion of potential future improvements or further exploration related to the Fibonacci sequence function or performance analysis.
Overall Presentation:
The assignment should be wellwritten, organized, and use proper grammar and formatting.
Assignment evaluation methodology for TA aattention
Implementation
Correctness points: The provided Fibonacci function fibonaccin must be correctly implemented in at least two programming languages: one interpreted eg Python and one compiled eg C Each implementation points each should accurately calculate the Fibonacci sequence for different input values.
Code Clarity points: The code should be wellformatted, indented, and include comments explaining the logic behind each step variable names, loops, calculations
Efficiency Considerations points: Bonus If applicable to the chosen languages, discuss any optimizations made to improve the performance of the function, especially for larger input values eg using memoization in a recursive approach
Analysis and Results
Running the Implementations points: The assignment should demonstrate running each implementation fibonaccin with different input values n Include a table or list showing the chosen input values and the corresponding output resulting Fibonacci numbers
Performance Measurement points: The assignment should report the execution time required for each run interpreted vs compiled languages using different input values. This can be achieved using builtin functions in the languages to measure execution time.
Data Visualization points: Bonus Present the execution time data for different input v
Step by Step Solution
There are 3 Steps involved in it
Step: 1
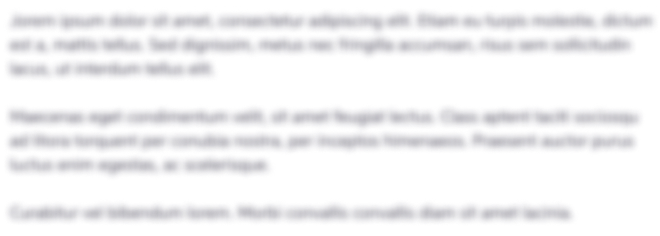
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started