Question
The Files provided to me are here, please don't change main at all. /***************************************************************** * File: assign05.cpp * Purpose: This main function will exercise all
The Files provided to me are here, please don't change main at all.
/*****************************************************************
* File: assign05.cpp
* Purpose: This main function will exercise all the classes of
* this project.
*
* You may comment out certain parts while you are working on the
* program, but you should make sure it is all present when you
* submit.
*****************************************************************/
#include
#include
#include
using namespace std;
#include "order.h"
#include "product.h"
#include "address.h"
#include "customer.h"
int main()
{
///////////////////////////////////////
// Address Class
///////////////////////////////////////
cout
cout
Address home;
home.display();
cout
cout
home.setStreet("Bag End");
home.setCity("The Shire");
home.setState("ME");
home.setZip("12345");
cout
cout
cout
cout
cout
cout
home.display();
cout
cout
Address hotel("The Prancing Pony", "Bree", "BR", "99999");
hotel.display();
cout
/////////////////////////////////////////
// Customer Class
/////////////////////////////////////////
cout
cout
Customer frodo;
frodo.display();
cout
cout
frodo.setName("Frodo Baggins");
frodo.setAddress(home);
cout
cout
frodo.getAddress().display();
cout
cout
Customer gandalf("Gandalf the Grey", hotel);
gandalf.display();
cout
/////////////////////////////////////////////
// Product Class
/////////////////////////////////////////////
cout
cout
Product theRing;
theRing.displayAdvertising();
cout
cout
theRing.setName("Ring of Power");
theRing.setDescription("One Ring to bring them all and in the darkness bind them");
theRing.setWeight(1);
theRing.setBasePrice(999);
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(2);
cout
cout
cout
cout
cout
cout
Product staff("Wizard Staff", "More than just a walking stick", 100, 10);
cout
staff.displayAdvertising();
cout
staff.displayInventory();
cout
staff.displayReceipt();
cout
////////////////////////////////////
// Order Class
////////////////////////////////////
cout
cout
Order orderRing;
orderRing.displayInformation();
cout
cout
orderRing.setProduct(theRing);
orderRing.setQuantity(1);
orderRing.setCustomer(frodo);
cout
orderRing.getProduct().displayReceipt();
cout
cout
orderRing.getCustomer().display();
cout
cout
cout
cout
Order orderStaff(staff, 2, gandalf);
cout
orderStaff.displayShippingLabel();
cout
orderStaff.displayInformation();
return 0;
}
/***************************************************************
* File: product.cpp
* Author: (your name here)
* Purpose: Contains the method implementations for the Product class.
***************************************************************/
#include "product.h"
#include
#include
#include
// put your method bodies here
void Product::prompt()
{
cout
getline(cin,name);
cout
getline(cin,description);
cout
cin >> weight;
while(weight
cout
cin >> weight; // Accept weight
}
do
{
if (cin.fail())
{
cin.clear();
cin.ignore(256, ' ');
}
cout
cin >> basePrice;
}
while (basePrice
}
double Product::getSalesTax()
{
return .06*basePrice;
}
double Product::getShippingCost()
{
if(weight
else
return 2+ (weight-5)*0.1;
}
double Product::getTotalPrice()
{
double total = basePrice+getSalesTax()+getShippingCost();
return total;
}
void Product::printAdvertising()
{
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(2);
cout
}
void Product::printInventory()
{
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(2);
cout
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(1);
cout
}
void Product::printReceipt()
{
cout.setf(ios::fixed);
cout.setf(ios::showpoint);
cout.precision(2);
cout
cout
cout
cout
cout
}
/***************************************************************
* File: product.h
* Author: (your name here)
* Purpose: Contains the definition of the Product class
***************************************************************/
#ifndef PRODUCT_H
#define PRODUCT_H
#include
using namespace std;
// put your class definition here
class Product
{
string name;
double basePrice;
double weight;
string description;
public:
string getName();
void prompt();
double getSalesTax();
double getShippingCost();
double getTotalPrice();
void printAdvertising();
void printInventory();
void printReceipt();
};
#endif
05 PROVE: ASSIGNMENT - ECOMMERCE PRODUCT INVENTORY, PART II Overview Having product lists and a way to compute shipping and total costs from your last project has immensely helped your growing on-line business, but to benefit even more from your program, you need to add customers This week, you will build on your Product library from last week, and create classes to manage orders and customers as wel Instructions Write a C++ program according to the following: 1. Create the following classes (as specified below): Address Contains a customer's address Customer - Contains a name and an Address Product - From last week (we will add a few methods to it) Order-Contains a Product, quantity, and a Customer
Step by Step Solution
There are 3 Steps involved in it
Step: 1
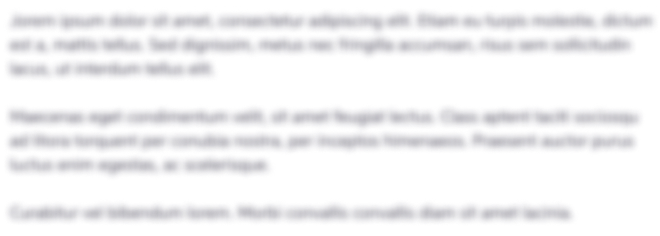
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started