Question
The first 3 classes are already completed, but I need help with the last 2 classes (4 & 5). After completing the classes, I need
The first 3 classes are already completed, but I need help with the last 2 classes (4 & 5). After completing the classes, I need to write a testbed main program that asks for sample data for the various employee types. Then, for each type calculate the employees pay and display her/his pay information on the screen and to an output file "pay.dat.
First 3 finished classes:
public class Employee {
protected String firstName; protected String lastName; protected String ssn; protected String employeeNumber;
public Employee(String firstNAme, String lastName, String ssn, String employeeNumber) { this.firstName = firstNAme; this.lastName = lastName; this.ssn = ssn; this.employeeNumber = employeeNumber; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstNAme) { this.firstName = firstNAme; }
public String getLastName() { return lastName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public String getSsn() { return "XXX-XX-" + ssn.substring(6); }
public String setSsn(String ssn) { if (ssn.length() == 11) { if (ssn.charAt(3) == '-' && ssn.charAt(6) == '-') {
if ('0' <= ssn.charAt(0) && ssn.charAt(0) <= '9' && '0' <= ssn.charAt(1) && ssn.charAt(1) <= '9' && '0' <= ssn.charAt(2) && ssn.charAt(2) <= '9' && '0' <= ssn.charAt(4) && ssn.charAt(4) <= '9' && '0' <= ssn.charAt(5) && ssn.charAt(5) <= '9' && '0' <= ssn.charAt(7) && ssn.charAt(7) <= '9' && '0' <= ssn.charAt(8) && ssn.charAt(8) <= '9' && '0' <= ssn.charAt(9) && ssn.charAt(8) <= '9' && '0' <= ssn.charAt(10) && ssn.charAt(10) <= '9' ) { this.ssn = ssn; return "success"; } } } return "failure"; }
public String getEmployeeNumber() { return employeeNumber; }
public String setEmployeeNumber(String num) { if (num.length() == 5) { if ('0' <= num.charAt(0) && num.charAt(0) <= '9' && '0' <= num.charAt(1) && num.charAt(1) <= '9' && '0' <= num.charAt(2) && num.charAt(2) <= '9' && num.charAt(3) == '-' && 'A' <= num.charAt(4) && num.charAt(4) <= 'M') { employeeNumber = num; return "success"; } } return "failure"; }
public void printDetails() { System.out.println("Name : " + firstName + " " + lastName); System.out.println("SSN : " + getSsn()); System.out.println("Employee Number: " + getEmployeeNumber()); } } =====================================================
public class SalaryEmployeePay extends Employee {
private double annualPay; private double weeklyPay; private char taxRateCode;
public SalaryEmployeePay(String firstName, String lastName, String ssn, String employeeNumber, double annualPay, double weeklyPay, char code) { super(firstName, lastName, ssn, employeeNumber); if (annualPay >= 0 && weeklyPay >= 0 && ('1' <= code && code <= '3')) { this.annualPay = annualPay; this.weeklyPay = weeklyPay; this.taxRateCode = code; } else { throw new IllegalArgumentException("Payments cannot be in negative"); } }
public double getAnnualPay() { return annualPay; }
public void setAnnualPay(double annualPay) { if (annualPay >= 0) this.annualPay = annualPay; }
public double getWeeklyPay() { return weeklyPay; }
public void setWeeklyPay(double weeklyPay) { if (weeklyPay >= 0) this.weeklyPay = weeklyPay; }
public char getTaxRateCode() { return taxRateCode; }
public void setTaxRateCode(char taxRateCode) { if ('1' <= taxRateCode && taxRateCode <= '3') this.taxRateCode = taxRateCode; } } =================================================
public class HourlyEmployee extends Employee {
private double hourlyRate; private double hoursWorked;
public HourlyEmployee(String firstName, String lastName, String ssn, String employeeNumber, double rate, double worked) { super(firstName, lastName, ssn, employeeNumber); if (rate >= 10 && rate <= 75 && worked <= 60) { hourlyRate = rate; hoursWorked = worked; } else { throw new IllegalArgumentException(); } }
public double getHourlyRate() { return hourlyRate; }
public void setHourlyRate(double rate) { if (rate >= 10 && rate <= 75) this.hourlyRate = rate; }
public double getHoursWorked() { return hoursWorked; }
public void setHoursWorked(double worked) { if (worked <= 60) this.hoursWorked = worked; } }
Full instructions: CLASS 1: Design a base class named Employee. The class should keep the following information in its data members:
- Employee name (separate first and last names)
- Social Security Number, in the format xxx-xx-xxxx, where x is a digit within the range 0 through 9
- Employee number, in the format xxx-L, where x is a digit within the range 0 through 9, and the L is a letter within the range A through M.
- Add a constructor and other appropriate member functions to manipulate the class data members (get, set, and print).
- Input Validation: Only accept valid Social Security Numbers (with no alphabetic characters) and valid Employee Numbers (as described above).
- Display of Social Security Number should be masked to only show the last four numbers, (e.g. xxx-xx-1234)
Note: Input validation will be done in the setter functions and no input statements should be used i.e. return a methodStatus value indicating success or failure.
CLASS 2. Design a class named SalaryEmployeePay. This class should be derived from the Employee class. It should
store the following information in data members:
- Annual pay
- Weekly pay (to be calculated from annual pay)
- Tax rate code (1 = 25%, 2 = 20%, 3 = 15%)
Add a constructor and other appropriate member functions to manipulate the class data members.
Input Validation: Do not accept negative values for annual pay. Do not accept values outside the range of 1 to 3 for tax rate code.
CLASS 3. HourlyEmployee. This class should be derived from the Employee class. It should store the following
information in data members:
- Hourly pay rate
- Number of hours worked
Add a constructor and other appropriate member functions to manipulate the class data members.
Input Validation: Hourly pay rate cannot be less than $10.00 per hour or more than $75.00 per hour. Do not accept values over 60 for hours worked.
CLASS 4. HourlyEmployeePay. This class should be derived from the HourlyEmployee class. It should store the
following information in data members:
- Overtime pay rate (1.5 times hourly rate if over 40 hours, calculate from HourlyEmployee hourly pay rate)
- Tax rate code (1 = 25%, 2 = 20%, 3 = 15%)
- Work status (F = Full time, P = Part Time)
Add a constructor and other appropriate member functions to manipulate the class data members.
Input Validation: Do not accept values outside the range of 1 to 3 for tax rate code, F or P for work status.
CLASS 5. AgencyEmployeePay. This class should be derived from the HourlyEmployee class. It should store the following information in data members:
- Company to pay
Add a constructor and other appropriate member functions to manipulate the class data members.
Using the classes you created, write a testbed main program that asks for sample data for the various employee types. Then, for each type calculate the employees pay and display her/his pay information on the screen and to an output file pay.dat.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
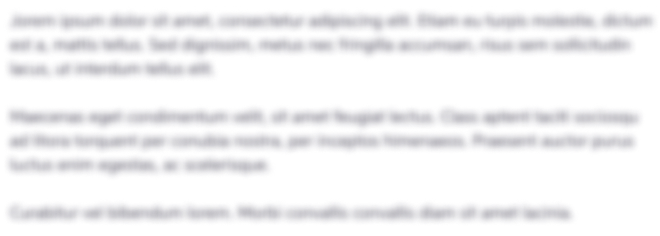
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started