Question
The first part of this assignment is to explore the use of threads in an application that computes PI. Create an appropriate Makefile to support
The first part of this assignment is to explore the use of threads in an application that computes PI. Create an appropriate Makefile to support the creation of the PI application (you can do this by modifying the Project1 Makefile). Compile and run this program a few times to understand the basic operation and to verify that it does approximate PI. Next, time your program and/or add appropriate timing system calls to your code. Run the application varying the number of threads and number of sample points. You may get a core dump when you exceed the number of threads permitted (note that value). Record the time(s) it takes your program to execute when changing the number of threads and number of sample points.
Create a plot of time vs. number of threads (or possibly time vs. log of the number of threads) when the number of sample points is fixed. Create a plot of time vs. number of sample points (or possibly time vs. log of the number of sample points) when the number of threads is fixed. Use an Excel like application to create your plot.
Code below:
#include
#define MAX_THREADS 512
void *compute_pi( void * );
int sample_points; int total_hits; int total_misses; int hits[ MAX_THREADS ]; int sample_points_per_thread; int num_threads;
int main( int argc, char *argv[] ) { /* local variables */ int ii; int retval; pthread_t p_threads[MAX_THREADS]; pthread_attr_t attr; double computed_pi;
/* initialize local variables */ retval = 0;
pthread_attr_init( &attr ); pthread_attr_setscope( &attr, PTHREAD_SCOPE_SYSTEM );
/* parse command line arguments into sample points and number of threads */ sample_points = atoi(argv[1]); num_threads = atoi(argv[2]);
printf( "Enter number of sample points: " ); scanf( "%d", &sample_points ); printf( "Enter number of threads: " ); scanf( "%d%", &num_threads );
total_hits = 0; sample_points_per_thread = sample_points / num_threads;
for( ii=0; ii for( ii=0; ii computed_pi = 4.0 * (double) total_hits / ((double) (sample_points)); printf( "Computed PI = %lf ", computed_pi ); /* return to calling environment */ return( retval ); } void *compute_pi( void *s ) { int seed; int ii; int *hit_pointer; int local_hits; double rand_no_x; double rand_no_y; hit_pointer = (int *) s; seed = *hit_pointer; local_hits = 0; for( ii=0; ii < sample_points_per_thread; ii++ ) { rand_no_x = (double) (rand_r( &seed ))/(double)RAND_MAX; rand_no_y = (double) (rand_r( &seed ))/(double)RAND_MAX; if(((rand_no_x - 0.5) * (rand_no_x - 0.5) + (rand_no_y - 0.5) * (rand_no_y - 0.5)) < 0.25) local_hits++; seed *= ii; } *hit_pointer = local_hits; pthread_exit(0); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
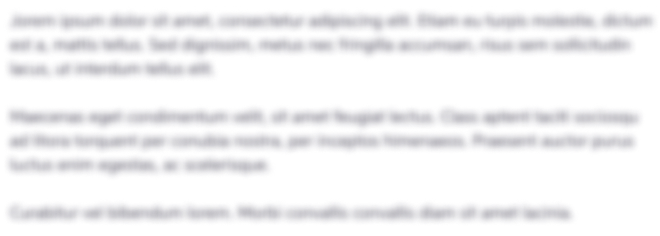
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started