Question
The following C program is an implementation of Queue using an array. #include #include #define size 11 void enqueue(int *q,int *tail, int element); int dequeue(int
The following C program is an implementation of Queue using an array. #include #include #define size 11 void enqueue(int *q,int *tail, int element); int dequeue(int *q,int *head); int full(int tail,const int size); int empty(int head,int tail); void init(int *head, int *tail); void init(int *head, int *tail) {*head = *tail = 0;} void enqueue(int *q,int *tail, int element) { q[(*tail)++] = element;} int dequeue(int *q,int *head) { return q[(*head)++];} int full(int tail,const int size) {return tail == size ? 1 : 0;} int empty(int head, int tail) {return head == tail ? 1 : 0;} } void main(){ int head,tail,element; int queue[size]; init(&head,&tail); // enqueue elements while(full(tail,size) != 1){ element = rand(); printf("enqueue %d ",element); enqueue(queue,&tail,element); printf("head=%d,tail=%d ",head,tail); } printf("queue is full "); // dequeue elements from while(!empty(head,tail)){ element = dequeue(queue,&head); printf("dequeue element %d ",element); } printf("queue is empty "); } Change it to an implementation in a circular fashion. Change it to handle the exceptions. Change the main() function, such that it receives integers from the standard I/O and perform enqueue() operations until the queue is full. performs one more enqueue() operation to show your program able to handle fullQueue exception. prints out the size and all the elements in the queue to show the queue is full with totally 10 integers in it. performs dequeue() operations until the queue is empty. performs one more dequeue() operation to show your program is able to handle emptyQueue exception.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
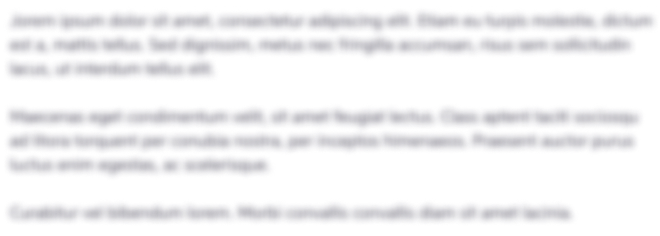
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started