Question
The following code doesn't allow for multiple clients to connect simultaneously.Implement the KnockKnock Client/Server to now allow for multiple clients to connect to the server
The following code doesn't allow for multiple clients to connect simultaneously.Implement the KnockKnock Client/Server to now allow for multiple clients to connect to the server simultaneously.
Client-code
package com.cheggg; import java.io.*; import java.net.*; public class KnockKnockClient { public static void main(String[] args) throws IOException { if (args.length != 2) { System.err.println( "Usage: java EchoClient
Server-code
package com.cheggg;
import java.net.*;
import org.json.simple.parser.ParseException;
import java.io.*;
public class KnockKnockServer { public static void main(String[] args) throws IOException, ParseException { if (args.length != 1) { System.err.println("Usage: java KnockKnockServer
protocol code
package com.cheggg;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
public class KnockKnockProtocol {
private static final int WAITING = 0;
private static final int SENTKNOCKKNOCK = 1;
private static final int SENTCLUE = 2;
private static final int ANOTHER = 3;
private static final int NUMJOKES = 5;
private int state = WAITING;
private int currentJoke = 0;
private String[] clues ;
private String[] answers ;
Random random = new Random();
Set
int index = 0;
KnockKnockProtocol() throws FileNotFoundException, IOException, ParseException{
//constructor to load json data
JSONParser parser = new JSONParser();
JSONArray a = (JSONArray) parser.parse(new FileReader("c:\\test.json"));
System.out.println(a.size());
clues=new String[a.size()];
answers=new String[a.size()];
for (Object o : a)
{
JSONObject person = (JSONObject) o;
String cluejson = (String) person.get("clue");
clues[a.lastIndexOf(o)]=cluejson;
String answerjson = (String) person.get("answer");
answers[a.lastIndexOf(o)]=answerjson;
}
index=random.nextInt(clues.length);
currentJoke = index;
validate.add(index);
}
public String processInput(String theInput) {
String theOutput = null;
/* Add First Randomly Genrated Number To Set */
// validate.add(random.nextInt(clues.length));
if (state == WAITING) {
theOutput = "Knock! Knock!";
state = SENTKNOCKKNOCK;
} else if (state == SENTKNOCKKNOCK) {
if (theInput.equalsIgnoreCase("Who's there?")) {
theOutput = clues[currentJoke];
state = SENTCLUE;
} else {
theOutput = "You're supposed to say \"Who's there?\"! " +
"Try again. Knock! Knock!";
}
} else if (state == SENTCLUE) {
if (theInput.equalsIgnoreCase(clues[currentJoke] + " who?")) {
theOutput = answers[currentJoke] + " Want another? (y/n)";
state = ANOTHER;
} else {
theOutput = "You're supposed to say \"" +
clues[currentJoke] +
" who?\"" +
"! Try again. Knock! Knock!";
state = SENTKNOCKKNOCK;
}
} else if (state == ANOTHER) {
if (theInput.equalsIgnoreCase("y")) {
theOutput = "Knock! Knock!";
if (currentJoke == (clues.length - 1)){
currentJoke = index;
}
else{
//System.out.println("index:::"+index);
while(validate.contains(index)) {
index = random.nextInt(clues.length);
//System.out.println("generated index:::"+index);
}
//index = random.nextInt(clues.length);
validate.add(index);
currentJoke=index;
}
state = SENTKNOCKKNOCK;
} else {
theOutput = "Bye.";
state = WAITING;
}
}
return theOutput;
}
}
test.json
[{
"clue":"Turnip",
"answer":"Turnip the heat, it's cold in here!"
},
{
"clue":"Little Old Lady",
"answer":"I didn't know you could yodel!"
},
{
"clue":"Atch",
"answer":"Bless you!"
},
{
"clue":"Who",
"answer":"Is there an owl in here?"
},
{
"clue":"Who",
"answer":"Is there an echo in here?"
}]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
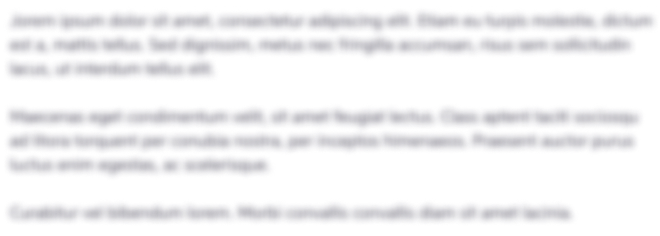
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started